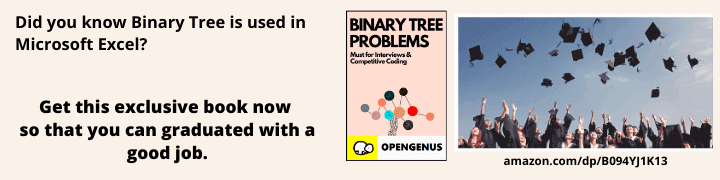
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will explore 4 different methods for initializing an unordered set in C++ STL. By the end of this discussion, you will have a better understanding of the different techniques available for creating and initializing an unordered set, and how to choose the right approach for your specific use case.
Table of contents:
- Introduction to unordered set
- Using default constructor
- Using initializer list
- Using range constructor
- Using copy constructor
Introduction to unordered set
An unordered set in C++ is an associative container that stores a set of unique objects in an unordered fashion. It is implemented using a hash table data structure that provides fast access, insertion, and deletion of elements based on their keys. The average time complexity of the operations like insertion, deletion, and search is O(1).
There are many ways to initialize unordered set in C++ such as:
- Using default constructor
- Using initializer list
- Using range constructor
- Using copy constructor
Using default constructor
The default constructor is the most commonly used method to initialize an unordered set in C++. It allows you to create an empty unordered set with no elements.
#include <unordered_set>
#include <iostream>
int main() {
std::unordered_set<int> mySet({1, 2, 3, 4});
for (const auto& val : mySet) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
Using initializer list
Another common way to initialize an unordered set in C++ is by passing a predefined list of elements as an argument to the default constructor of the unordered set.
#include <unordered_set>
#include <iostream>
int main() {
std::unordered_set<int> mySet = {1, 2, 3, 4};
for (const auto& val : mySet) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
Using range constructor
Another way to initialize an unordered set in C++ is by using the range constructor and passing a vector of the same data type. This allows you to create an unordered set with the same elements as the vector.
#include <unordered_set>
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1,2,3,4};
std::unordered_set<int> mySet(vec.begin(), vec.end());
for (const auto& val : mySet) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
Using copy constructor
You can also initialize an unordered set in C++ by using the copy constructor and copying the contents from another set one by one. This allows you to create a new unordered set with the same elements as the original set.
#include <unordered_set>
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::unordered_set<int> mySet = {1, 2, 3, 4};
std::unordered_set<int> copySet(mySet);
for (const auto& val : copySet) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
With this article at OpenGenus, you must have the complete idea of how to initialize unordered map in C++ STL.