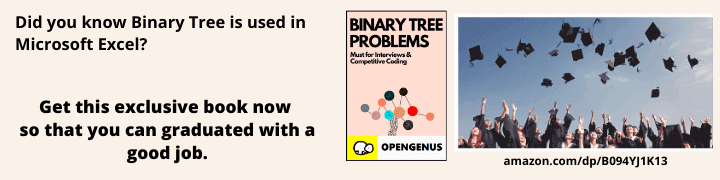
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will explore different ways to insert elements in unordered_map in C++ STL. This can be greatly helpful in using maps and solving complex coding questions.
TABLE OF CONTENTS
- Introduction to unordered_map
- Insertion in Unordered_map
- Method 1 : Insert()
- Syntax
- Code
- Output
- Method 2 : [] operator
- Syntax
- Code
- Output
- Method 3 : Emplace()
- Syntax
- Code
- Output
- Time Complexity
INTRODUCTION TO UNORDERED_MAP
Unordered_map is a container that stores elements formed by the combination of a key value and a mapped value. The key value is used to uniquely identify the element and the mapped value is the content associated with the key. The elements are stored in random fashion i.e. they are not sorted.
Syntax :
unordered_map<char,int> mp;
INSERTION IN UNORDERED_MAP
For inserting the elements in the map that is key-value pair in the map we have many different ways. We will look at all of them one by one and understand how to effectively use them.
METHOD 1 : INSERT()
The unordered_map::insert() is a built-in function in C++ STL which is used to insert elements with a specific key in the unordered_map container. This function increases container size by 1.
Syntax 1:
iterator map_name.insert({key,value});
This returns an iterator which points to the new element inserted into the map.
#include<bits/stdc++.h> //including all the liberarires
using namespace std;
int main()
{
unordered_map<char, int> cmap; // declaration of map
cmap.insert({'a', 1}); // inserting the key 'a' with corresponding pair value as 1
cmap.insert({'b', 2});
cmap.insert({'c', 3});
cmap.insert({'d', 4});
for (auto &it : cmap) //traversing the map with auto iterator
{
cout << it.first << " " << it.second << "\n";
}
}
OUTPUT
KEYS | VALUES |
---|---|
a | 1 |
c | 3 |
b | 2 |
d | 4 |
To copy whole container
If we want to copy one map onto the other map, we can do the following.
Syntax 2 :
iterator map_name.insert(iterator start, iterator end);
#include<bits/stdc++.h> //including all the liberarires
using namespace std;
int main()
{
unordered_map<char, int> cmap; // declaration of map
cmap.insert({'a', 1}); // inserting the key 'a' with corresponding pair value as 1
cmap.insert({'b', 2});
cmap.insert({'c', 3});
cmap.insert({'d', 4});
unordered_map<char, int> mp; // another map
mp.insert(cmap.begin(), cmap.end()); // copying all the contents of cmap onto mp
for (auto &it : mp) //traversing the map with auto iterator
{
cout << it.first << " " << it.second << "\n";
}
}
OUTPUT
KEYS | VALUES |
---|---|
d | 4 |
b | 2 |
a | 1 |
c | 3 |
METHOD 2 : INSERT USING []
If you have come across the array container then you must have used the sqaure brackets. We can also use them to insert elements into our unordered_map. It is a very convenient way to insert elements and unlike previous methos it also looks comparatively cleaner.
Syntax
map_name[key] = value;
#include<bits/stdc++.h> //including all the liberarires
using namespace std;
int main()
{
unordered_map<char, int> cmap; // declaration of map
cmap['a'] = 1; // inserting using the square brackets.
cmap['b'] = 2;
cmap['c'] = 3;
cmap['d'] = 4;
for (auto &it : cmap) //traversing the map with auto iterator
{
cout << it.first << " " << it.second << "\n";
}
}
OUTPUT
KEYS | VALUES |
---|---|
b | 3 |
c | 2 |
d | 4 |
a | 1 |
This method also allocates defualt values to the key if no value is provided for the corresponding key. For accessing the values also we can use the same method.
#include<bits/stdc++.h> //including all the liberarires
using namespace std;
int main()
{
unordered_map<int, int> cmap; // declaration of map
cmap[1] = 1;
cmap[2] = 2;
cmap[3] = 3;
cmap[4] = 4;
cmap[5]; // default value of zero would be assigned here
cmap[6];
for (int i = 1; i <= 6; i++) //traversing the map
{
cout << i << " --> " << cmap[i] << "\n"; // using [] to access the elements
}
}
OUTPUT
KEYS | VALUES |
---|---|
1 | 1 |
2 | 2 |
3 | 3 |
4 | 4 |
5 | 0 |
6 | 0 |
METHOD 3 : EMPLACE()
It is an inbuilt C++ function used to insert values. In C++ we can use both insert and emplace to insert elements. Both are used to add an element in the container.
The advantage of emplace is, it does in-place insertion and avoids an unnecessary copy of object. For primitive data types, it does not matter which one we use. But for objects, use of emplace() is preferred for efficiency reasons.
Syntax
iterator.emplace(key, value);
#include<bits/stdc++.h> //including all the liberarires
using namespace std;
int main()
{
unordered_map<char, int> cmap; // declaration of map
cmap.emplace('a', 21); //using emplace to insert
cmap.emplace('b', 22);
cmap.emplace('c', 23);
cmap.emplace('d', 24);
cmap.emplace('e', 25);
for (auto &it : cmap)
{
cout << it.first << " --> " << it.second << "\n";
}
}
OUTPUT
KEYS | VALUES |
---|---|
b | 22 |
c | 23 |
d | 24 |
a | 21 |
e | 25 |
TIME COMPLEXITY
For the three methods mentioned above the time complexity :
- INSERT() : log(N)
- [] operator : log(N)
- EMPLACE() : log(N)
where N is the size of the map.
In this article at OpenGenus, you must have got the complete "Different ways to insert element in unordered_map in C++ STL".