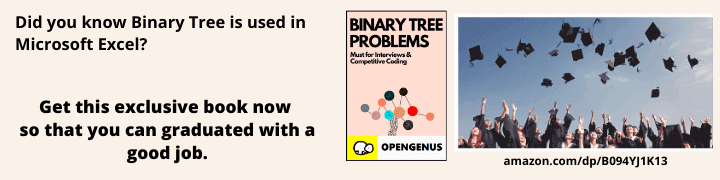
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained MVC pattern in iOS apps in depth. MVC stands for Model View Controller which is a software design pattern to organize the software project into three parts model,view and controller
Table of content:
- What is MVC software design pattern?
- How MVC works?
- Implementing MVC in iOS apps
We will dive into it.
What is MVC software design pattern?
MVC stands for Model View Controller which is a software design pattern to organize the software project into three parts model,view and controller.
Model
The model is a data storage component of the pattern it uses a data strucutre to deal with all the UI independent data.It also directly manages the rules and data of the application.
View
The view is the user interface through which user interacts with the application. It includes UI components like buttons,tableview,collection view etc.
Controller
The controller act as a bridge between Model and View.Both Model and View can communicate with each other only through controller.
How MVC works?
The basic functionality of MVC is shown below
Here the user sees the view and interact with the app. For example consider a app which which list numbers in ascending order in a table view. When user want to sort the numbers in descending order then the user sends the request by selecting sort option in UI(View) which sends request to the controller the controller will go to Model component and make the model component to sort the data in descending order and update data displayed in View with sorted numbers.
Implementing MVC in iOS apps
We are going to create a Quiz app which uses MVC patter where all the questions along with their answers will be stored in model layer.The UI will display the question which is fetched from Model layer by using Controller.
- Open up Xcode and create a blank UIKit project
- In project navigator create three new groups Model,View,Controller and add the following files inside them
- Open up Main.storyboard where we are going to design our UI which comes under View component.Design a UI similar to shown below
- Now open QuizModel file and add the following code
import Foundation
class QuizModel
{
static let SharedObj = QuizModel()
private let Questions: [String] = [
"2 + 10 = ?",
"What is capital of Vermont?",
"Who is the founder of Microsoft"
]
private let Answers: [String] = [
"12",
"Montpelier",
"Bill and Paul"
]
func getQuestions() -> [String]
{
return Questions
}
func getAnswers() -> [String]
{
return Answers
}
}
This structure holds two array one for question and another for answers.Here we use a singleton object to interact with them through the controller.
- Open up CustomButton file and add the below code
import Foundation
import UIKit
class CustomButton: UIButton
{
override func awakeFromNib() {
super.awakeFromNib()
layer.cornerRadius = 5
backgroundColor = UIColor.systemOrange
layer.borderWidth = 2
layer.borderColor = #colorLiteral(red: 0, green: 0, blue: 0, alpha: 1)
}
}
Here we define custom properties for UIButton by sub classing the UIButton class.
- Open up controller file and add the IBOutlets and IBActions for the UI components and add the below lines of code
class ViewController: UIViewController {
var Questions = [String]()
var Answers = [String]()
var currentquestionindex = 0
@IBOutlet weak var QuestionLabel: UILabel!
@IBOutlet weak var AnswerLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
Questions = QuizModel.SharedObj.getQuestions()
Answers = QuizModel.SharedObj.getAnswers()
QuestionLabel.text = Questions.randomElement()
}
@IBAction func NextQuestionButtonPressed(_ sender: Any) {
currentquestionindex += 1
if(currentquestionindex == Questions.count)
{
currentquestionindex = 0
}
else
{
QuestionLabel.text = Questions[currentquestionindex]
}
}
@IBAction func ShowAnswerButtonPressed(_ sender: Any) {
AnswerLabel.text = Answers[currentquestionindex]
}
}
Here we define the core function of the app. This file act as the controller. It receives user interaction with Views using IBOutlets and IBAction and send the user request to model and fetch data from model and update the view with the data.
All set now build the project and run it in the simulator.
Let's end this article with a quiz
Question
What is the other software design pattern often used in iOS apps
With this article at OpenGenus, you must have the complete idea of MVC pattern in iOS apps.