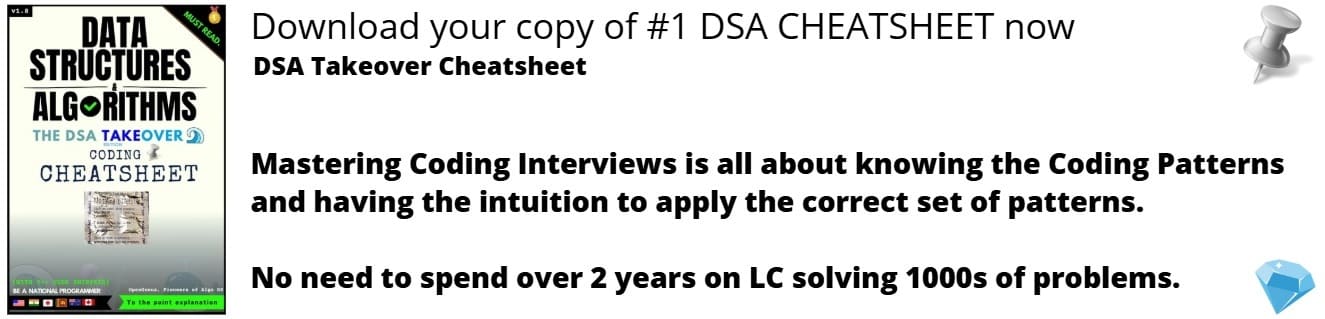
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will learn how to print a parallelogram star pattern using C++. This is a common programming exercise that can help beginners get familiar with loops and basic C++ syntax.
Understanding the Parallelogram Star Pattern
A parallelogram star pattern is a pattern of stars arranged in a parallelogram shape, like the following:
**********
**********
**********
**********
As you can see, the pattern consists of rows of stars that are shifted to the right by one space for each row. In other words, the first row has no spaces, the second row has one space, the third row has two spaces, and so on. Each row contains the same number of stars.
Writing the C++ Program
Let's write a C++ program to print the parallelogram star pattern. Here's the code:
#include <iostream>
using namespace std;
int main() {
int rows, cols;
// Input the number of rows and columns
cout << "Enter the number of rows: ";
cin >> rows;
cout << "Enter the number of columns: ";
cin >> cols;
// Print the pattern
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= i - 1; j++) {
cout << " ";
}
for (int j = 1; j <= cols; j++) {
cout << "*";
}
cout << endl;
}
return 0;
}
Let's break down this code step by step.
Including the Libraries
First, we include the iostream library, which is needed for input/output operations in C++. We also declare that we will be using the std namespace to simplify our code.
using namespace std;
Declaring Variables
Next, we declare two variables, rows and cols, which will hold the number of rows and columns in the pattern, respectively. We use the cout and cin objects to prompt the user for these values and read them from the keyboard.
cout << "Enter the number of rows: ";
cin >> rows;
cout << "Enter the number of columns: ";
cin >> cols;
Printing the Pattern
Now comes the main part of the program: the nested loops that print the pattern. We use a for loop to iterate over the rows of the pattern. For each row, we use another for loop to print the required number of spaces before the stars. We calculate the number of spaces by subtracting 1 from the row number, since the first row has no spaces.
for (int j = 1; j <= i - 1; j++) {
cout << " ";
}
After printing the spaces, we use another for loop to print the required number of stars. We simply print the cols variable number of stars each time.
cout << "*";
}
Finally, we use endl to move to the next line and start the next row. We repeat this process for each row of the pattern.
cout << endl;
}
With this article at OpenGenus, you must have the complete idea of how to print Parallelogram Star Pattern in C++.