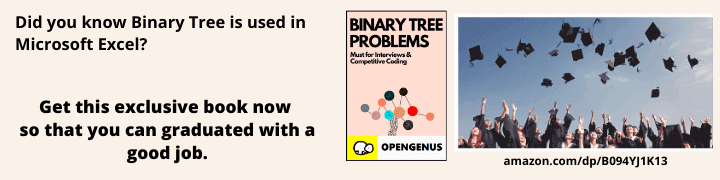
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will learn to print Rhombus pattern in C++ language. We will go with two types of Rhombus patterns that is solid Rhombus and hollow Rhombus.
- Solid Rohmbus Pattern in C++
- Hollow rohmbus pattern in C++
1. Solid Rhombus Pattern in C++
Making a solid square pattern for an i'th row with n-i spaces is quite similar to making a solid rhombus design for any given integer n.
Approach -
- Initialize variables i and j.
- Loop from i = 1 to n:
- Loop from j = 1 to n ā i and print spaces.
- Loop from j = 1 to n and print asterisks.
- Print a new line.
Code-
#include <bits/stdc++.h>
using namespace std;
// Function for Solid Rhombus
void solidRhombus(int n) {
int i, j;
for (i = 1; i <= n; i++) {
for (j = 1; j <= n - i; j++) {
std::cout << " ";
}
for (j = 1; j <= n; j++) {
std::cout << "*";
}
std::cout << std::endl;
}
}
int main()
{
int rows = 4;
solidRhombus (rows);
return 0;
}
Output -
*****
*****
*****
*****
2. Hollow Rhombus Pattern in C++
The concept behind the hollow squares and solid rhombuses is used to create hollow rhombuses. A hollow rhombus is formed when a hollow square has nāi blank spaces before the stars in each ith row.
Approach-
- Initialize variables i and j.
- Loop from i = 1 to n:
- Loop from j = 1 to n ā i and print spaces.
- Loop from j = 1 to n:
- If i = 1 or i = n or j = 1 or j = n, print an asterisk.
- Else, print a space.
- Print a new line.
Code -
#include <bits/stdc++.h>
using namespace std;
void hollowRhombus(int n) {
int i, j;
for (i = 1; i <= n; i++) {
for (j = 1; j <= n - i; j++) {
std::cout << " ";
}
for (j = 1; j <= n; j++) {
if (i == 1 || i == n || j == 1 || j == n) {
std::cout << "*";
} else {
std::cout << " ";
}
}
std::cout << std::endl;
}
}
int main()
{
int rows = 5;
hollowRhombus (rows);
return 0;
}
Output-
*****
* *
* *
* *
*****
Because there are two nested loops that repeatedly iterate over all the rows and columns of the rhombus, the time complexity of both functions is O(n2).
Due to the fact that no matter how big the rhombus is, only a fixed amount of memory is required, the space complexity is O(1).
With this article at OpenGenus, you must have the complete idea of how to print Rhombus Pattern in C++.