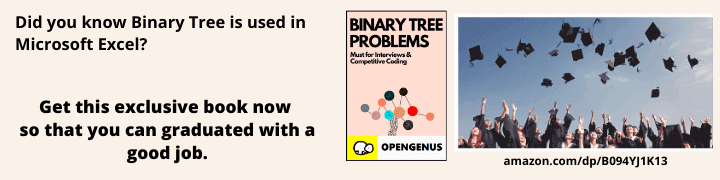
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the differences between 3 output methods in C++ namely, puts vs printf vs cout in C++.
Table of content-
- Introduction to puts,printf and cout
- Their properties and implementation.
- Differences between puts,printf and cout
Introduction to puts,printf and cout -
1. puts -
puts is a c library function declared in header file stdio.h. it is used to write a string to stdout puts(str) it writes a string without including the null character .so the new line character is appended to the output. i.e moves the cursor to new line.
return value -
On successful non-negative value is returned and if error arises it returns EOF.
its implementation is given below:
#include <bits/stdc++.h>
using namespace std;
int main () {
char s1[30];
char s2[20];
strcpy(s1,"this is post about puts");
strcpy(s2,"hello world ");
puts(s1);
puts(s2);
return(0);
}
Output :
this is post about puts
hello world
2. printf -
printf is a library function defined in stdio.h header file . it can be used in both c and c++.
printf needs parameters and format specifiers for sending formated string on output screen.e.g.
printf("character = %c", ch);
printf("number = %f\n", number);
printf("two numbers are x and y %d and %f", x,y);
By using printf we can not access user defined datatypes.e.g class,struct,union etc because they don't have any format specifiers.printf is assigned at runtime.
printf returns an integer value i.e the number of characters printed.
e.g
#include <bits/stdc++.h>
int main(){
char str[] = "This is opengenus";
printf("\n The value returned by printf() is : %d", printf(" %s", str));
return 0;
}
Output :
This is opengenus
The value returned by printf() is : 18
3. cout -
cout is an predefined object of class ostream under the header file iostream .
cout doesn't require any format specifiers and it doesn't return any value .
cout can access user defined datatype directly with the help of operator overloading(>> ,<<) .cout is assigned at compile time.
e.g.
#include <bits/stdc++.h>
using namespace std;
int main( ) {
int date ;
cout << "Enter todays date ";
cin >> date;
cout << "todays date is: " << date << endl;
}
Output :
Enter todays date 30
todays date is: 30
Differences between puts,printf and cout
puts | printf | cout |
---|---|---|
puts is a complete predefined library function in stdio.h | printf() is a function used to print formatted string on stdout it is defined in cstdio header file | cout is an object of ostream class it is defined in iostream header file |
puts is not extensible at all it only used to print strings | printf is not extensible to large extent, except user defined data types it can take all types of arguments in formatted specifier | cout is by default extensible as it can take custom formats and user defined data types becuase of overloading of << output stream operator. |
puts function syntax is int puts(const char* str) | printf is a function it takes parameters and format specifiers like %c,%f etc. | cout is an object with defined overload operator << ,it is an stream oriented output stream object . |
puts append a newline character \n at the end of the passed string . | printf writes output to stdout under format strings without new line character | cout also writes to stdout under format strings without new line character |
puts is simpler version of printf used only to print strings | The real advantage of printf lies in Translation as printf format string is also a string so it can be translated easily | Translation in cout is more difficult due to operator overloading (<<) and having a large extensibility. |
As puts works only on single data type string so it is type safe | printf is not typesafe | cout is type safe |
puts doesn't uses flush by deafult so we have to flush stdout using fflush function manually. | printf also doesn't uses flush by deafult so we have to flush stdout manually | cout flushes automatically after each output operation |
puts is simpler and preferred for printing strings over printf as it is faster and less expensive than printf | printf is much faster as compared to cout as it doesn't need to sync with c library's stdio buffer all time. | cout is relatively slower as compared to printf, cause it has to keep in sync with c library's stdio buffer |
Above table shows the differences and properties related to puts,printf and cout.