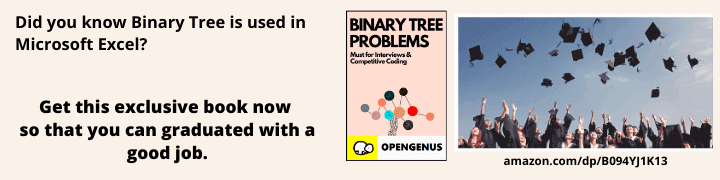
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
We can run any command from shell scripts. We can get the output of the commands in a variable and run commands in loops with different parameters as well. The shell script is a file with file extension "sh".
- Add shebang construct
- Add commands
- Complete code
- Add execution permission
- Run shell script
- Define a variable
- Get Input
- Define a loop
1. Add shebang construct
Add a shebang construct so that the UNIX system knows that it is a shell script.
#!/bin/bash
OR
#!/bin/sh
2. Add commands
We can add any command in the shell script and it will be executed one by one. For example, we need add two commands:
pip install numpy
python code.py
ls
On this, once the first command "pip install numpy" completes, then only the second command "python code.py" starts executing.
The command "ls" executes after the first two commands.
3. Complete code
The complete code is as follows:
#!/bin/bash
pip install numpy
python code.py
ls
4. Add execution permission
Add permission to execute the code
chmod +x commands.sh
5. Run shell script
Run the script code file
bash commands.sh
6. Define a variable
We can define a variable in a shell script as follows:
MESSAGE = "This is a variable"
echo $MESSAGE
7. Get Input
To get input from user, we can use "read".
echo "Enter a number between 1 and 10"
#reading input
read NUMBER
echo $NUMBER
To get input from output of a command:
PW_DIR=$(pwd)
8. Define a loop
We can define a loop as follows:
a=0
while [ "$a" -lt 10 ]
echo $a
done
This will run for values of a = 0 to 9 (both inclusive).
With a loop, you can run a command with different parameters.
With this, you must have a good idea of how to run UNIX commands from shell scripts.