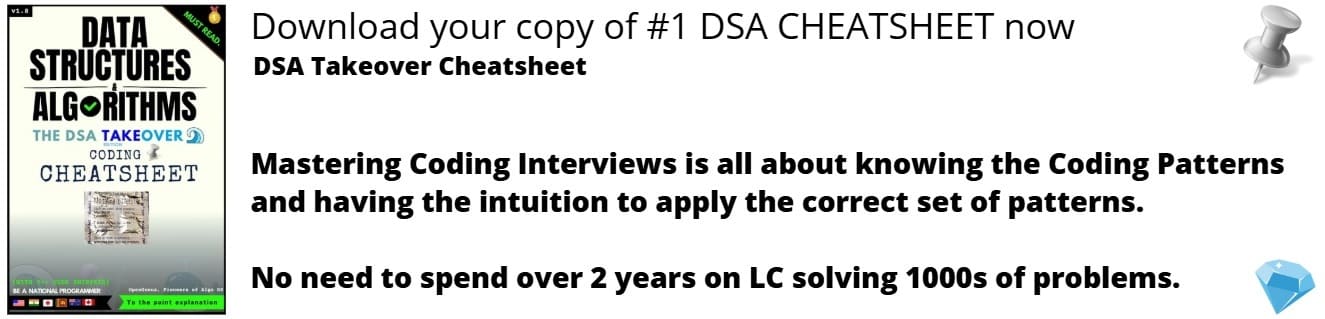
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented different ways using which one can set all elements in an array to 0 in C++. We have presented an assembly level approach (advanced) as well.
Table of contents:
- Solution 1: memset
- Solution 2: Static initialization
- Solution 3: std::fill()
- Solution 4: loop
- Solution 5: Using AVX instructions [Advanced]
Solution 1: memset
The fastest way to set all elements of an array to 0 is C++ is to use memset() function which is defined in string header file. memset is an intrinsic so the compiler will convert it to assembly instructions directly making its use highly optimal.
memset(array, 0, sizeof(array));
// OR
memset(array, 0, N*sizeof(*array));
memset will place the value 0 in each byte of array. So, the 3 parameters specifies the number of bytes to set. With this, the array can be set to any constant value.
Following is the complete C++ code using memset to set all elements of an array to 0:
#include <string.h>
int main() {
int* array = (int*)malloc(10 * sizeof(int));
memset(array, 0, 10 * sizeof(int);
return 0;
}
Solution 2: Static initialization
If array is statically initialized, then this approach works best and is a clean approach as well. If array is dynamically initialized, then memset or other approaches should be used.
T array[10] = {0};
Following is the complete C++ code using std::fill to set all elements of an array to 0:
#include <string.h>
int main() {
int array[10] = {0};
return 0;
}
Solution 3: std::fill()
In this approach, we use std::fill() method from <algorithm>
to fill an array with 0s. This is a decent method in terms of performance but still, memset outperforms this when used correctly.
std::fill(array, array+N, 0);
Following is the complete C++ code using std::fill to set all elements of an array to 0:
#include <string.h>
int main() {
int* array = (int*)malloc(10 * sizeof(int));
std::fill(array, array+10, 0);
return 0;
}
Solution 4: loop
This is the slowest method. In this, we will loop through all elements of an array and manually set each element to 0.
Following is the complete C++ code using std::fill to set all elements of an array to 0:
#include <string.h>
int main() {
int* array = (int*)malloc(10 * sizeof(int));
for(int i=0; i<10; ++i) {
array[i] = 0;
}
return 0;
}
One can use other looping alternatives to for loop as well.
Solution 5: Using AVX instructions [Advanced]
This approach use core concepts of AVX instructions to implement an efficient solution to set all elements of an array to 0.
In specific cases, this approach works even faster than memset. If your system supports AVX512, then you can update this code accordingly to use AVX512 instructions to outperform all code for this task.
The AVX instructions used for this are:
_mm256_storeu_ps
: This is used to store/ move a single precision floating point values from a float32 vector to a 256-bit unaligned memory._mm256_setzero_ps
: This is used to set YMM registers of type float32 (vector) to 0 value. It returns the same float32 vector._mm_storeu_ps
: This is used to store four 32 but float values into memory (128 bits). This is similar to assembly instructions VMOVUPS and MOVUPS._mm_setzero_ps
: This is used to set a 128 bit memory to 0.
The idea is to load parts of array to 256 bit registers, initialize them to 0, bring to back to original memory. Do this till the entire array is covered.
Following is the complete C++ code using AVX instructions:
#include<immintrin.h>
#define set_ZERO(a,n) {
size_t x = 0;
// size of 256 bit register over size of variable
const size_t inc = 32 / sizeof(*(a));
for (X = 0; x < n-inc; x += inc)
_mm256_storeu_ps((float *)((a)+x),_mm256_setzero_ps());
if(4 == sizeof(*(a))) {
switch(n-x) {
case 3:
(a)[x] = 0;
x++;
case 2:
_mm_storeu_ps((float *)((a)+x),_mm_setzero_ps());
break;
case 1:
(a)[x] = 0;
break;
case 0:
break;
};
}
With this article at OpenGenus, you must have the complete idea of how to set all array elements to 0.