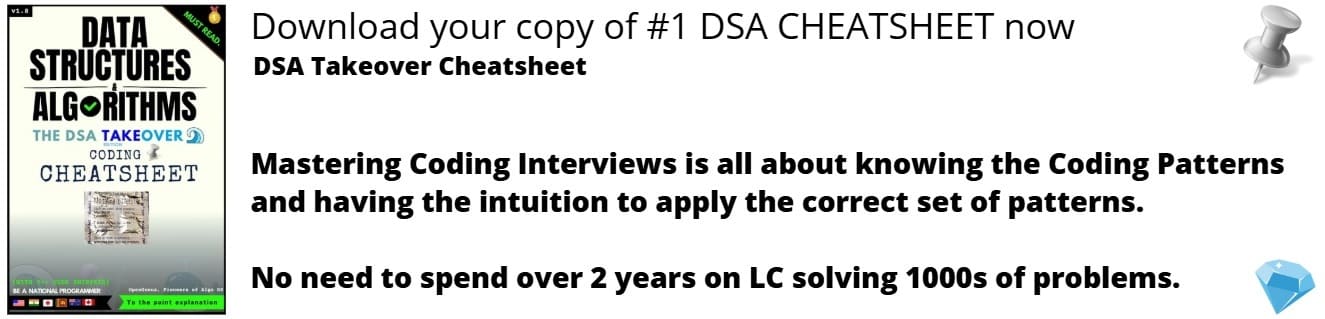
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about static_cast in C++ Programming Language and understand when to use it in contrast to similar functions like dynamic_cast.
Table of contents:
- static_cast in C++
- Examples of using static_cast
- Example of using dynamic_cast
static_cast in C++
In C++, static_cast is a type casting operator which is used to convert a value of one datatype to another.
It is typically used to perform conversions between numeric types, such as int to float or double, or to convert a pointer to a different type of pointer. As long as there is an inheritance relationship between the two classes, it can also be used to convert a class type into one of its base classes.
The type conversion between two datatypes with a known and well-defined relationship is done using static_cast. As long as the two types are compatible and the conversion is defined by the programming language, it can be used to convert values between different types.
The syntax of using static_cast:
static_cast<new_datatype>(expression)
- new_datatype is the data type to which you want to convert the value of expression.
Examples of using static_cast:
int x = 12;
double y = static_cast<double>(x); // y is now 12.0
char ch = 'a';
int n = static_cast<int>(ch); // n is now 97
const int* p = &x;
int* q = static_cast<int*>(p); // q points to x
class Base {};
class Derived : public Base {};
Derived* d = new Derived;
Base* b = static_cast<Base*>(d);
Note: static_cast does not perform any runtime type checking, we need to ensure that the conversion is valid. If the conversion is not valid, the behavior is undefined. This indicates that the conversion's result is not predetermined by the C++ standard and may differ based on the C++ compiler's particular implementation.
Consider the following code:
struct X {};
struct Y {};
X a;
Y b = static_cast<Y>(a); // invalid conversion
In the above code, we are trying to use static_cast to convert an object of type X into an object of type Y, but these types are not related in any way. This is an invalid conversion, and the behavior of the static_cast operation is undefined.
We need to ensure that the conversion we are trying to do with static_cast is valid to prevent this kind of problem. It means that the datatypes which we are trying to convert between are related in some way, either through inheritance or through a built-in type conversion.
If we are not sure whether a conversion is valid or not,we can use dynamic_cast or reinterpret_cast. These operators provide additional type safety and can help you detect invalid conversions at runtime.
Example of using dynamic_cast:
struct Base { virtual ~Base() {} };
struct Derived : Base {};
Base* b = new Derived;
Derived* d = dynamic_cast<Derived*>(b); // d points to the derived object
In this example, we have a polymorphic type hierarchy with a base class Base and a derived class Derived. We create a pointer to a Derived object and assign it to a pointer of type Base*. We then use dynamic_cast to convert the Base* pointer to a Derived* pointer. Because the object pointed to by b is actually a Derived object, the conversion is successful and d points to the Derived object.
dynamic_cast returns a pointer or reference to the converted value if the conversion is successful and if the conversion is unsuccessful, dynamic_cast returns a null reference or a null pointer.
With this article at OpenGenus, you must have the complete idea of static_cast in C++.