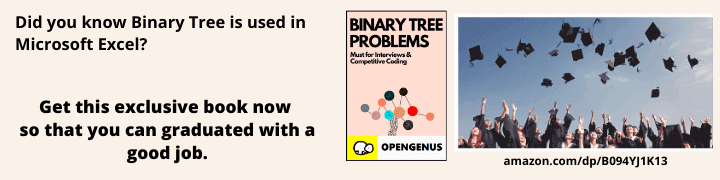
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the System Design of StackOverflow which will one of the most widely used websites for online question and answers. The design is pretty simple which one would not expect considering its scale.
Table of contents:
- INTRODUCTION
- SYSTEM REQUIREMENTS AND SPECIFICATIONS
- DESIGN
- IMPLEMENTATION AND UNIT TESTING
- Technology stack
1. INTRODUCTION
Stack Overflow is an online community platform for developers. Its main activity is allowing developers to ask and provide answers to technical questions, as well as providing a private collaboration platform for different team members over the world to share organizational knowledge.
2. SYSTEM REQUIREMENTS AND SPECIFICATIONS
Users and use cases
Unregistered users (any person with no account) can search questions, Stack Overflow user accounts, collaborations, associated companies and tags.
Registered users have accounts, They are capable of asking, and answering questions, create a team, join a team, follow a question, join a collective and perform some other basic actions. With more privileges, they can comment, vote down or up a question, set bounties on questions, create tags and tags synonyms, delete questions etc. More abilities are available with higher privileges.
Team members are those account owners who are belong to a team. They can ask questions and answer questions in their teams. Anything happening within a team is unavailable to any external person.
Collaborators of a team are those team members who can delete content from the team and edit articles.
Admins are team members who can activate and deactivate members of the team, invite any registered user to join the team, grant and revoke privileges to any team member.
The use case diagram below shows some users and some of their use cases.
The various use cases are analyzed to yield the functional requirements of the software system
Functional requirements
Includes operations that any user of Stack Overflow is capable of performing.
1 Visitors
Unregistered users who can:
. List all available public questions.
. List all companies associated to Stack Overflow.
. Sign up to Stack Overflow
. List all available tags.
. List all communities of Stack Overflow.
2 Members
Users who own account and depending on their privileges, they are capable of:
. Posting questions
. Modifying questions
. Posting answers
. Modifying answers
. comment a question
. Up vote or down vote a post.
. Delete asked question
. Delete any question.
. Sign in
. Join teams
. Create teams
. change email settings.
. logout
and more
3 Team members
Any member of the platform who participates in a team can:
. Ask a question in a team
. Answer a question in a team.
4 Team Moderators
A member of a given team who can:
. edit an article in a team.
. Delete contents of the team.
5 Admin
Team members with the highest privileges:
. Deactivate or activate any member of the team.
. Invite a Stack Overflow member to join his team.
. Change team member role.
Non-Functional requirements
These requirements define how well the system is going to perform its functions. Non-Functional requirements of Stack Overflow include:
- Scalabilty
A plat-form like StackOverflow should be capable of handling and increase in the amount of work through addition of resources.
- Performance
This includes small response time, high throughput (amount of work done per unit time), determines how fast StackOverflow responds to user request.
- Availability
The probability that a user can access Stack Overflow at any time should be very high even though millions of developers over the world are using StackOverflow at different moments
- Usability
Using the platform should be very easy. Stack Overflow has extensive use cases, hence should provide good graphical interfaces with easy to use tools for good user experience.
- Security
Important information on users, teams, collectives and companies should protected from unauthorized individuals. Prevent users from granting themselves privileges.
- Extensibility
Addition of new function or feature should not be difficult.
Design Constraints
Includes decisions that were already made for the developers restricting their degree of freedom.
- Using C# as Development language.
- ASP.NET MVC used as development framework.
- Using a relational database management system for data storage (Microsoft SQL server)
Another activity which happens simultaneously with this phase is the database analysis. This includes getting the real-world entities which are going to represented in a database.
3. DESIGN
System Architecture.
Stack Overflow is designed with respect to the Model-View-Controller Architectural pattern (MVC) which is a modern representation of a monolithic architecture. Such an architecture can be represented as follows
System modeling.
Modeling a system like Stack Overflow is done using the Object Oriented approach. The internal structure of the software system will depend on the structure of what is to be modeled, "an online platform for developers to share knowledge", which consists of entities such as developers (users), questions, teams, answers, solutions, tags etc , some of which are represented below.
The abstract dataBaseAccess
contains method declarations for accessing the database, these methods are defined in it's child classes where operations are performed according to a particular set of data.
Sequence diagrams are necessary to show the interaction logic between the objects in the system in the time order that the interactions take place.
The database tables and relations structures are setup with a relational database management system. The data in some of these tables are analogous to those in some classes.
The algorithms for each classes are written, new methods are often discovered since it's not easy to figure out all the methods at once(some methods are just there to assist other methods)
4. IMPLEMENTATION AND UNIT TESTING
Having established the software architecture, it is now easy to organize the source code.
Using System;
class Question{
private ulong id;
private int voteCount;
private string title;
private string content;
//getters and setters
public string Content{
get{return content;}
set{id=value;}
}
public ulong Id{
get{return id;}
set{id=value;}
}
public int VoteCount{
get{return voteCount;}
set{voteCount=value;}
}
public string Title{
get{return title;}
set{title=value;}
}
//methods
public void voteUp(){
++this.voteCount;
}
public void voteDown(){
--this.voteCount;
}
public void delete(){
//...
}
public void undelete(){
//...
}
// ...
}
class Comment{
private ulong id;
private string content;
Comment( string content){
this.content = content;
}
//getters and setters
public ulong Id{
get{return id;}
set{id=value}
}
public string Content{
get{return content;}
set{content=value;}
}
public void editComment();
//...
}
class User{
//Methods
public void listQuestions();
public void listTags();
public void signup();
}
class Account : User {
protected string name;
protected ulong id;
protected string passwd;
public Question question;
//getters and setters
public Account( string name, string passwd ){
this.name = name;
this.passwd=passwd;
}
public string Name{
get{return name;}
set{name=value;}
}
public string Id{
get{return id;}
set{id=value}
}
public string Passwd{
get{return passwd;}
set{passwd=value;}
}
//User methods
public void joinTeam();
}
class TeamMember : Account{
//...
}
//...
Each coded class are tested individually from the database access class to the user class.
5. Integration and integration testing
Assembling units and testing them progressively until a whole system is integrated.
6.System testing
Once fully integrated, the system is tested to ensure that it covers all the requirements stated above.
7.Installation
Deploy the system on servers making it available to its end users.
Technology stack
Stack Overflow used the following technologies for its development;
ASP.NET MVC
Highly optimized and light weight web development framework supporting C#, F#, Visual Basic, C++. Some of features of this framework are;
Model validation: which is done through data annotation validation attributes. Validation attributes are checked before posting values on to the server.
Dependency Injection: follows explicit dependency principles, enables the registration of dependency logic for improving class reusing, reducing class coupling, increasing code maintainability and improve application testing
Cross plat-form application development: applications developed with ASP.NET do not only support windows, but also support other platforms such as GNU/linux and Mac OS
Visual studio IDE
Contains components for ASP.NET MVC and web development. Provide tools for easy coding with C#. Visual studio is also highly extensible.
Microsoft SQL server
SQL server is a relational database management system developed and introduces Microsoft. It includes its SQL language and Transact-SQL, Microsoft's proprietary language with capabilities of exception handling, declaring variable, and store procedures.
SQL server offers enhanced performance to secure and encrypt the data, users need not modify program.
SQL server is highly secured, uses sophisticated encryption algorithms making it difficult to break its security layers.
Jquery
A JavaScript a library for developing interactive web pages
jquery makes it very easy and straightforward to perform common tasks like manipulating the webpage, responding to events, getting data from servers, building effects and animations etc.
With this article at OpenGenus, you must have the complete idea of System Design of StackOverflow.