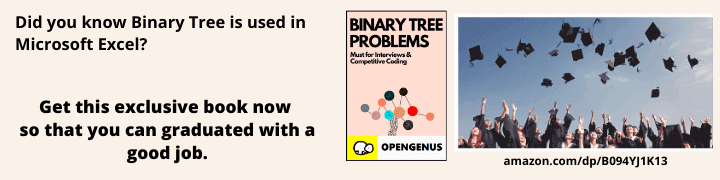
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
When it comes to advanced programming scenarios in C, the setjmp.h
header stands as a unique tool that enables the implementation of non-local jumps and control flow manipulation. In this article at OpenGenus, we'll delve into the intricacies of setjmp.h
, uncovering how it works, its practical applications, and the advantages it brings to the table.
Table of contents:
- Understanding Non-Local Jumps
- The Basics of setjmp() and longjmp()
- Working Mechanism
- Advantages and Disadvantages
- Alternatives to Non-Local Jumps
- Best Practices and Considerations
- Real-World Applications
- Conclusion
Understanding Non-Local Jumps
Before we dive into the specifics of setjmp.h
, let's understand the fundamental difference between local and non-local jumps in C programming. Local jumps, facilitated by the goto
statement and labels, allow for control transfers within the same function. These jumps are typically frowned upon due to their potential to make code hard to maintain and understand.
To illustrate, consider the following code snippet that demonstrates a local jump:
#include <stdio.h>
void localJumpExample() {
int i = 1;
start:
if (i <= 5) {
printf("Local Jump: %d\n", i);
i++;
goto start;
}
}
int main() {
printf("Local Jump Example:\n");
localJumpExample();
return 0;
}
In this example, the localJumpExample()
function uses the goto
statement and labels to perform a local jump. The goto start;
statement transfers control back to the start:
label, effectively creating a loop-like behavior within the same function.
The output of the above program would be:
Local Jump Example:
Local Jump: 1
Local Jump: 2
Local Jump: 3
Local Jump: 4
Local Jump: 5
To learn more about goto
statements in C, head over to this amazing article.
On the other hand, non-local jumps extend beyond the confines of a single function. They involve transferring control from one function to another, across different levels of the call stack. This is where setjmp.h
comes into play. The header provides two crucial functions: setjmp()
and longjmp()
, which work together to achieve non-local jumps.
The Basics of setjmp() and longjmp()
The foundation of non-local jumps is laid by the setjmp()
function. When setjmp()
is invoked, it captures the current execution context, including register values and the program counter, and stores it in a buffer. This captured context becomes a jump point that can be returned to later using the longjmp()
function.
To better understand the mechanics, consider the following code snippet illustrating non-local jumps:
#include <stdio.h>
#include <setjmp.h>
jmp_buf jump_buffer;
void nonLocalJumpExample() {
int i = 1;
if (setjmp(jump_buffer) == 0) {
printf("Non-Local Jump: %d\n", i);
i++;
longjmp(jump_buffer, 1); // Jump back to setjmp point
} else {
printf("Continuing after Non-Local Jump\n");
}
}
int main() {
printf("Non-Local Jump Example:\n");
nonLocalJumpExample();
return 0;
}
In this example, the nonLocalJumpExample()
function demonstrates a non-local jump using setjmp()
and longjmp()
. It prints the number 1 and then sets up a jump point using setjmp()
. It increments the value of i
, and then invokes longjmp()
to perform a non-local jump back to the setjmp()
point. This effectively bypasses the increment of i
and continues execution from the point of the setjmp()
call.
Working Mechanism
To further illustrate the working mechanism of setjmp.h
, consider a real-world scenario where error handling requires immediate control transfer to an error-handling routine. Let's see how setjmp()
and longjmp()
can accomplish this:
#include <stdio.h>
#include <setjmp.h>
jmp_buf jump_buffer;
void errorHandler() {
printf("An error occurred. Handling it...\n");
longjmp(jump_buffer, 1); // Non-local jump to the setjmp point
}
void performTask() {
if (setjmp(jump_buffer) == 0) {
// Perform a task that might encounter an error
printf("Performing a critical task...\n");
errorHandler(); // Simulate an error condition
} else {
printf("Continuing after error handling.\n");
}
}
int main() {
printf("Error Handling Example:\n");
performTask();
return 0;
}
In this example, the errorHandler()
function simulates an error condition and invokes longjmp()
to perform a non-local jump back to the setjmp()
point in the performTask()
function. This demonstrates how non-local jumps can be used for immediate control transfer to handle exceptional situations.
Advantages and Disadvantages
Non-local jumps using setjmp()
and longjmp()
offer several advantages in specific scenarios. For instance, they can simplify error handling in nested function calls, where multiple levels of the call stack need to be unwound to reach an error-handling routine. Additionally, they can be useful in situations requiring resource cleanup.
However, non-local jumps also come with certain disadvantages. The code that employs non-local jumps can become harder to read, understand, and debug. Managing resources properly across non-local jumps can be challenging, potentially leading to memory leaks and other unexpected behavior. Therefore, careful consideration should be given to the complexity and readability of the code.
Alternatives to Non-Local Jumps
While setjmp.h
provides a mechanism for non-local jumps, it's essential to explore alternative control flow mechanisms that enhance code clarity and maintainability. For example, structured error handling using setjmp()
and longjmp()
can be replaced with modern practices like exceptions in languages that support them. These alternatives can help avoid the convoluted control flow associated with non-local jumps.
Best Practices and Considerations
When using setjmp.h
, it's crucial to follow best practices to mitigate potential issues. Clear documentation of non-local jumps and their jump points helps maintain code readability. Additionally, ensure that resources are properly managed, taking into account any allocated memory, open files, or other system resources that may need cleanup after a non-local jump.
Real-World Applications
setjmp.h
finds practical application in various scenarios. For instance, interpreters and compilers often use it to manage complex control flows during program execution. It's also valuable in handling asynchronous events, such as signal handling, where the program's state needs to be preserved across non-local jumps.
Conclusion
In the realm of advanced C programming, setjmp.h
provides a unique approach to non-local jumps and control flow manipulation. By enabling developers to transfer control from one function to another, it offers solutions for complex error handling and intricate control flows. However, the intricacies and potential complexities of non-local jumps call for their careful use. Consider alternatives where possible, and always prioritize code readability and maintainability in your programming endeavors.