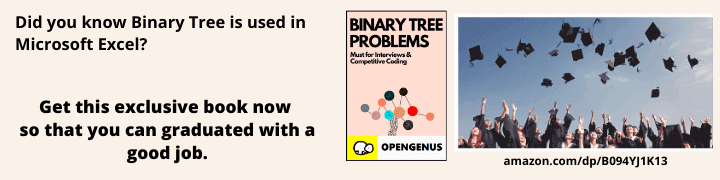
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 2 minutes
The goto statement is a jump statement which is sometimes also referred to as unconditional jump statement. The goto statement can be used to jump from anywhere to anywhere within a function.
Syntax
1 | 2
goto label; | label:
. | .
. | .
. | .
label: | goto label;
In the above syntax the first line tells the compiler to go to or jump to the statement marked as label. Here label is a user defined identifier which indicates the target statement. The statement immediately followed after ‘label:’ is the destination statement. The ‘label:’ can also appear before the ‘goto label;’ statement in above syntax.
Syntax1
Example:
In this case we will see a situation similar to as shown in Syntax1 above. Suppose we need to write a program where we need to check if a number is even or not and print accordingly using goto statement. Below program explains how to do this:
// C program to check if a number is
// even or not using goto statement
#include <stdio.h>
void checkEvenOrNot(int num)
{
if (num % 2 == 0)
goto even; // jump to even
else
goto odd; // jump to odd
even:
printf("%d is even",num);
return; // return if even
odd:
printf("%d is odd",num;
}
int main()
{
int num = 26;
checkEvenOrNot(num);
return 0;
}
Output:
26 is even
Note the return statement after ‘even:’ in checkEvenOrNot function. Once the control jumps to the label with even:, the program will execute every piece of code after it. So it is important to return if the number is even. Otherwise the code after label odd will also gets executed as it comes after even.
Syntax2
Example: In this case we will see a situation similar to as shown in Syntax1 above. Suppose we need to write a program which prints numbers from 1 to 10 using goto statement. Below program explains how to do this.
// C program to print numbers
// from 1 to 10 using goto statement
#include <stdio.h>
// function to print numbers from 1 to 10
void printNumbers()
{
int n = 1;
label:
cout << n << " ";
n++;
if (n <= 10)
goto label;
}
int main()
{
printNumbers();
return 0;
}
Output:
1 2 3 4 5 6 7 8 9 10
Converting a program without goto statement to with goto
//Voting age criteria
//Without goto statement
#include<stdio.h>
void main()
{
int age = 19;
if(age>=18)
printf("You are Eligible\n");
else
printf("You are not Eligible");
getch();
}
Output:
You are Eligible
//Voting age criteria
//With goto statement
#include<stdio.h>
void main()
{
int age = 19;
g: printf("You are Eligible\n");
s:printf("You are not Eligible");
if(age>=18)
goto g; //goto label g
else
goto s; //goto label s
getch();
}
Output:
You are Eligible
Convert a loop into goto statements
Every loop can be replaced by a set of goto statements.
Code without goto statement:
//without goto statment loop
#include<stdio.h>
int main()
{
int count=1;
if(count<=10)
{
printf("%d\n", count);
count++;
}
}
Output:
1
2
3
4
5
6
7
8
9
10
Code with goto statement:
//goto statment with loop
#include<stdio.h>
int main()
{
int count=1;
loop:
printf("%d\n", count);
count++;
if(count<=10)
goto loop;
}
Output:
1
2
3
4
5
6
7
8
9
10
Disadvantages of using goto statement:
-
The use of goto statement is highly discouraged as it makes the program logic verycomplex.
-
Use of goto makes really hard to modify the program.
-
Use of goto can be simply avoided using break and continue statements.
Question
Consider the following C code:
#include <stdio.h>
int main()
{
printf("%d ", 1);
goto l1;
printf("%d ", 2);
l1:goto l2;
printf("%d ", 3);
l2:printf("%d ", 4);
}
What will be the output of the above C code?
2) goto l1 will lead to goto l2
3) Hence last printf statement will be excuted