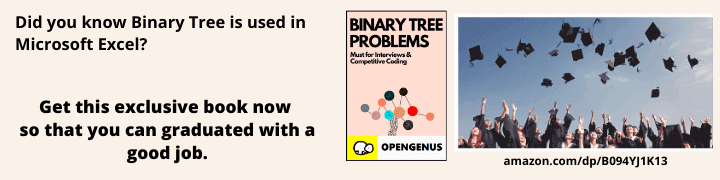
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we are going to study about the time.h standard header file in C Programming Language and cover the different methods available in it.
Table of Content
Prerequisites
You should have basic knowledge about :-
Introduction and Declaration
- The <time.h> is a standard header file in C programming language which is used for manipulating date and time.
- It consists of Data Types, MACROS and Built In Functions.
#include<time.h> //Header File
MACROS
-
NULL :- A null pointer constant.
-
CLOCKS_PER_SEC :- It defines number of clock ticks /sec.There are 1,000,000 ticks/sec.
Data Types
-
size_t :- A type for storing date and time.
-
clock_t :- A type to measure processor time.
-
struct tm :- It is a structure that stores all kinds of useful information about the calender time.
struct tm{
int tm_sec; //represents seconds (0, 59)
int tm_min; //represents minutes (0, 59)
int tm_hour; //represents hour (0, 23)
int tm_mday; //represents day of a month (1, 31)
int tm_mon; //represents months (0, 11)
int tm_year; //represents year since 1900
int tm_wday; //represents days (0, 6)
int tm_yday; //represents days since January 1(0,365)
int tm_isdst; //represents daylight saving time flag
/*
tm_isdst is positive is in effect,
tm_isdst is zero if not in effect,
tm_isdst is negative if otherwise.
*/
};
Built In Function
Time Manipulation Functions
Function | Description |
---|---|
clock() | This function determines the processors time used. |
time() | This function determines the current calender time in seconds. |
difftime() | This function outputs the difference between two calender time in seconds. |
mktime() | This function converts the the local time in the structure (tm) into calender time format. |
Time Conversion Functions
-
These functions return a pointer to one of two types of static objects.
Examples :- A broken-down time structure or an array of characters. -
Programs will clarify these statements given ahead.
Function | Description |
---|---|
ctime() | This function converts the calendar time pointed to by timer to local time in the form of a string. |
localtime() | This function converts the calendar time pointed to by timer into a broken-down time. |
gmtime() | This function converts the calendar time pointed to by timer into a broken-down time expressed as Coordinated Universal Time(UTC). |
asctime() | This function returns a pointer to the string. |
clock() Function
time_t clock(void); //Function Declaration
- It returns processor time used by program or returns -1 if time is not available.
#include<stdio.h>
#include<time.h>
int main(){
time_t ticks, timer; //Declaring variable of time_t type
for(int i=0;i<600000;i++) //running loop to consume processor time
{}
ticks = clock();
timer = clock()/CLOCKS_PER_SEC;
printf("Number of clock ticks %d\n", ticks);
printf("Number of seconds the processor was in use %f sec", timer);
return 0;
}
Output
1990
0.000000
Why is time in seconds consumed by processor zero ?
- Our computer is fast so the process is executed instantly.
time() Function
time_t time(time_t *timer); //Function Declaration
- This function returns the calender time in seconds or -1 if time is not available.
#include <stdio.h>
#include <time.h>
int main ()
{
time_t timer;
printf("The number of seconds passed %d",time(&timer)); // get current time; same as: timer = time(NULL)
return 0;
}
Output
1680107360
What is this ?
- This value represents the number of seconds passed since 00:00 hours, Jan 1, 1970 UTC .
So if we run the program again then,
1680107368
- The difference between these two outputs represents the number of seconds passed.
Why 1970 ?
- This represents UNIX epoch(birthday).
difftime() function
double difftime(time_t end, time_t start); //Function Declaration
#include<stdio.h>
#include<time.h>
int main(){
time_t start, end; //Declaring variable of time_t type
start = clock();
for(int i=0;i<50577;i++) //Running for loop to consume processor time.
{}
end = clock();
printf("%f", difftime(end, start));
return 0;
}
Output
147.000000
mktime() Function
time_t mktime(struct tm *tp); //Function Declaration
- This function returns the calendar time as a value of type time_t or returns the value (time_t)(-1), if the calendar time cannot be represented.
#include<stdio.h>
#include<time.h>
int main() {
struct tm t;
time_t time;
t.tm_year = 2023 - 1900; //Since the year starts from 1900 not from 0
t.tm_mon = 2;
t.tm_mday = 30;
t.tm_hour = 11;
t.tm_min = 40;
t.tm_sec = 03;
t.tm_isdst = 0;
time = mktime(&t);
printf(ctime(&time)); //This function is explained ahead.
}
Output
Thu Mar 30 11:40:03 2023
ctime() Function
char *ctime(const time_t *timer); //Function Declaration
#include<stdio.h>
#include<time.h>
int main() {
time_t t;
t = time(NULL);
printf(ctime(&t));
}
Output
Thu Mar 30 05:18:53 2023
localtime() Function
struct *localtime(const time_t *timer); //Function Declaration
#include<stdio.h>
#include<time.h>
int main() {
time_t t;
struct tm *local;
t = time(NULL);
local = localtime(&t);
printf("Time %d:%d:%d\n", local->tm_hour, local->tm_min, local->tm_sec); //Variables from in-built data type struct tm
printf("Date %d:%d:%d", local->tm_mday, local->tm_mon, local->tm_year);
return 0;
}
Output
Time 5:36:39
Date 30:2:123
Why the year is 123 ?
- Here the year starts from 1900 rather than 0.
- So, 1900 + 123 = 2023
gmtime() Function
struct *gmtime(const time_t *timer); //Function Declaration
#include<stdio.h>
#include<time.h>
int main() {
time_t t;
struct tm *global;
t = time(NULL);
global = gmtime(&t);
printf("Time %d:%d:%d\n", global->tm_hour, global->tm_min, global->tm_sec); //Variables from in-built data type struct tm
printf("Date %d:%d:%d", global->tm_mday, global->tm_mon, global->tm_year);
return 0;
}
Output
Time 0:8:21
Date 30:2:123
asctime() Function
char *asctime(const time_t *timer); //Function Declaration
#include<stdio.h>
#include<time.h>
int main() {
time_t t;
struct tm *local;
t = time(NULL);
local = localtime(&t);
printf(asctime(local));
return 0;
}
Output
Thu Mar 30 05:41:45 2023
Conclusion
So, in this article at OpenGenus, we have study all about the <time.h> header file in depth.