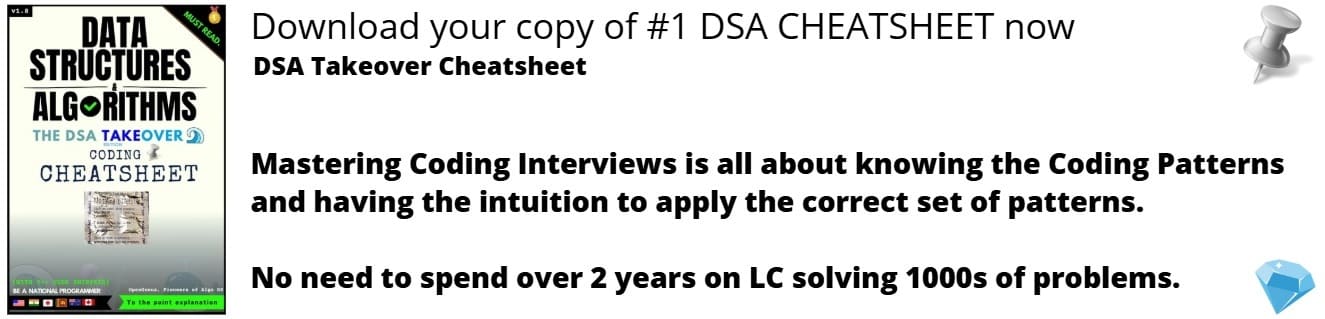
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
A structure is defined as a collection of same/different data types. All data items thus grouped logically related and can be accessed using variables.
Table of contents:
- Basics of Structure in C
- Structure Declaration
2.1. Tagged Structure
2.2. Structure Variables
2.3. Type Defined Structures - Structure Initialization in C
- Accessing Structures in C
- Array of Structure in C
- Nested Structures in C
Let us get started with Structure (struct) in C.
Basics of Structure in C
"Struct" keyword is used to identify the structure.
Example:
struct studentDetails {
char name[10];
char gender[2]; // F for female or M for male
int number;
char parentsName[20];
char address[20];
};
Memory Allocation
-
Always, contiguous(adjacent) memory locations are used to store structure members in memory.Consider above example to understand how memory is allocated for structures.
-
There are 5 members declared for structure in above program. In 32 bit compiler, 4 bytes of memory is occupied by int datatype. 1 byte each of memory is occupied by char datatype.
-
Memory is reserved only if the above definition is associated with variables.That is once the structure are defined,they have to be declared .Then only 56 bytes of memory space is reserved.
Structure Declaration
Structure can be declare using three different ways:
1: Tagged Structure
2: Structure Variables
3: Type Defined Structures
1: Tagged Structure
The structure name with tag name is called tagged structure.The tag name is the name of the structure.
struct studentDetails {
char name[10];
char gender[2]; // F for female and M for male
int number;
char parentsName[20];
char address[20];
};
- Here studentDetails is the tag name.
- To allocate the memory for the structure, we have to declare the variable as shown below
struct studentDetails Jack,Jonas;
Once the structure variables are declared, the compiler allocates memory for the structure, So 32 bytes are reserved for the variable Jonas and another 32 bytes are reserved for the variable Jack.The size of the memory allocated is the sum of size of individiual members
2: Structure Variables
It is possible to declare variables of a structure, either along with structure definition or after the structure is defined.
Structure variable declaration is similar to the declaration of any normal variable of any other data type.
struct Student {
char name[25];
int age;
char branch[10];
//F for female and M for male
char gender;
} S1,S2 ;
Observe that 40 bytes of memory is allocated for the variable S1 and another 22 bytes of memory is allocated for the variable S2.
Note: We avoid this way of defining and declaring the structure variablesm because of the following reasons:
- Without a tag,it is not possible to declare variables in later stages inside the functions.
- It is not possible to use them as parameter in the function,because all the parameter have to be declared.
- We can define them only in the beginning of the program.In such situations,they are treated as global variables.In the structured programming it is better to avoid the usage of global variables.
3: Typed-Defined Structure
The Structure definition associated with keyword typedef is called type-defined structure.This is the most powerful way of defining the structure
typedef struct Person
{
int age;
char name[25];
}p1,p2,p3;
Structure Initialization in C
It can be initialized in various ways
Method 1: Specify the initializers within the braces and seperated by commas when the variables are declared as shown below:
struct Student {
char name[25];
int age;
char branch[10];
//F for female and M for male
char gender;
}S1 = {"John",14,"CSE","M"};
Method 2: Specify the initializers within the braces and seperated by commas when the variables are declared as shown below:
struct Student
{
char name[25];
int age;
char branch[10];
//F for female and M for male
char gender;
};
struct S1 = {"John",14,"CSE","M"};
Accessing Structures in C
We can access structure in two ways:
- By . (memeber or dot operator)
- By -> ( structure pointer operator)
#include<stdio.h>
struct Student
{
char name[25];
int age;
char branch[10];
//F for female and M for male
char gender;
};
int main()
{
struct Student s1;
// s1 is a variable of Student type and
// age,name,branch,gender is a member of StudeNT and assigning values to them using // dot(.)operator
s1.age = 18;
s1.name = "John";
s1.branch = "C.S.E";
s1.gender = "F";
/*
displaying the stored values
*/
printf("Name of Student 1: %s\n", s1.name);
printf("Age of Student 1: %d\n", s1.age);
printf("Branch of Student 1: %s\n", s1.branch);
printf("Gender of Student 1: %s\n", s1.gender);
return 0;
}
Output:
Name of student 1: John
Age of Student 1: 18
Branch of Student 1: C.S.E
Gender of Student 1:F
Array of Structure in C
The array of structures in C are used to store information about various member of different data types.It is also known as collection of structure.
#include <stdio.h>
struct Employee
{
char name[10];
int sal;
};
int main() {
struct Employee emp[2];
for(int i = 0; i<2 ;i++){
printf("%d employee name",i+1);
scanf("%s",emp[i].name);
printf("salary of %d employee",i+1);
scanf("%d",&emp[i].sal);
}
return 0;
}
Output:
1 employee name John
salary of 1 employee 120000
2 employee name Jonas
salary of 2 employee 130000
Nested Structures in C
Nested structure means structure within structure. As we have declared members inside the structure in the same we can declare declare structure.
#include <stdio.h>
struct Student
{
// here additionalInfo is a structure
struct additionaInfo
{
char address[20];
char parentsname[30];
int mobileno;
}info;
char collegeId[10];
char name[10];
int age;
} stu;
int main()
{
printf("Give Student college id:");
scanf("%s",stu.collegeId);
printf("Give Student Name:");
scanf("%s",stu.name);
printf("Give Student age:");
scanf("%d",stu.age);
printf("Give Student address:");
scanf("%s",stu.info.address);
printf("Give Student parentsName:");
scanf("%s",stu.info.parentsname);
printf("Give Student mobileno:");
scanf("%d",stu.info.mobileno);
return 0;
}
Output:
Give Student college id:12345
Give Student Name: John
Give Student age:20
Give Student address: Bangalore
Give Student parentsName: Jonas
Give Student mobileno : 12456
With this article at OpenGenus, you must have a complete idea of structure (Struct) in C / C++ Programming Language.