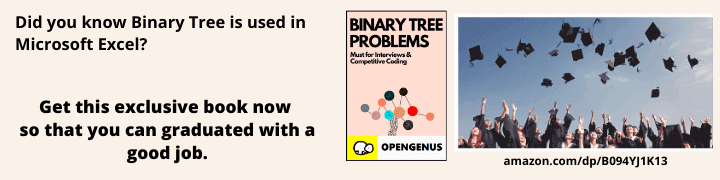
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
TensorFlow is a popular open-source library for machine learning and deep learning tasks. It provides a wide range of operations and functions to build and train neural networks effectively. One of the fundamental operations is addition, which is essential for combining or summing up tensors.
In this article at OpenGenus, we will dive into the TensorFlow layers.Add operation, explore its usage in Deep Learning models through both mathematical expressions and Python code snippets.
But first of all lets answer one fundamental question.
Why to add Layers in a machine learning model ?
Adding layers to a machine learning model, particularly in deep learning, can bring several benefits. Here are a few reasons why adding layers is often beneficial:
- Increased Model Capacity: By adding layers, you increase the model's capacity to learn complex patterns and relationships in the data.
- Hierarchical Feature Extraction: Each layer in a deep neural network can learn and extract different levels of features from the input data.
- Improved Model Performance: Deeper models have the potential to achieve better performance compared to shallow models, especially when dealing with complex tasks.
- Non-Linear Transformations: Deep neural networks are composed of multiple non-linear activation functions sandwiched between layers.
- End-to-End Learning : Adding layers enables end-to-end learning, where the model learns directly from the raw input to the desired output without the need for explicit feature engineering.
- Transfer Learning: Deep models with multiple layers can be pre-trained on large datasets and then fine-tuned on smaller, task-specific datasets.
Mathematical Expression:
The layers.Add operation in TensorFlow allows us to add multiple tensors element-wise. Given two tensors, say A and B, the layers.Add operation computes a new tensor C such that each element of C is the sum of the corresponding elements from A and B.
The Add operation in TensorFlow.Layers.Add is used to add two tensors together. The tensors can be of any shape or size, and the operation will automatically broadcast the smaller tensor to match the size of the larger tensor.The operation can be represented using the following mathematical expression:
C = A + B
In this expression, A, B, and C are tensors of the same shape.
Python Code:
In python code, the Add operation can be called using the tf.add() function. The following python code adds the tensors x and y:
import tensorflow as tf
# Define input tensors
tensor_a = tf.constant([1, 2, 3])
tensor_b = tf.constant([4, 5, 6])
# Perform element-wise addition using layers.Add
output_tensor = tf.layers.Add()([tensor_a, tensor_b])
# Create a TensorFlow session and run the computation
with tf.Session() as sess:
result = sess.run(output_tensor)
print("Result:", result)
In this code example, we start by importing the TensorFlow library. We then define two input tensors, tensor_a and tensor_b, using the tf.constant function. These tensors represent the numbers [1, 2, 3] and [4, 5, 6], respectively.
Next, we use the tf.layers.Add() function to perform element-wise addition on the two input tensors. This function takes a list of tensors as input and returns a tensor that represents the element-wise sum of the inputs.
Finally, we create a TensorFlow session and run the computation by calling sess.run(output_tensor). The result is stored in the result variable and printed to the console.
Multiple ways to use add operation:
The Add operation can be used in a variety of different ways. For example, it can be used to add two tensors together, to add a constant to a tensor, or to add two tensors together and then apply a function to the result.
-
Adding Two Tensors Together
We can add two tensors together using the add operation.
For example, the following code adds the tensors x and y:
x = tf.constant([1, 2, 3])
y = tf.constant([4, 5, 6])
z = tf.add(x, y)
print(z)
This code will print the following output:
#output
[5 7 9]
-
Adding a Constant to a Tensor
We can add a constant to tensor using the add operation.
For example, the following code adds the constant 10 to the tensor x:
x = tf.constant([1, 2, 3])
z = tf.add(x, 10)
print(z)
This code will print the following output:
#output
[11 12 13]
-
Adding Two Tensors Together and Then Applying a Function
We can add two tensors together and then apply a function to the result using the add opertion. For example, the following code adds the tensors x and y and then applies the square root function to the result:
x = tf.constant([1, 2, 3])
y = tf.constant([4, 5, 6])
z = tf.add(x, y)
z = tf.sqrt(z)
print(z)
This code will print the following output:
#output
[1.0 1.41421356 1.73205081]
Hands on Machine Learning
Now we will explore the use of Add operation of keras library in popular machine learning models like ResNet50, LSTMs and GRU.
-
ResNet50
ResNet50 is a popular deep convolutional neural network (CNN) architecture used for image classification. In ResNet50, the layers.add function is used to add residual blocks to the model. Residual blocks allow the network to learn residual mappings, which helps alleviate the vanishing gradient problem and enables the training of deeper networks. Here's an example of how layers.add is used in ResNet50:
from tensorflow.keras.applications import ResNet50
from tensorflow.keras.layers import Dense, Input, add
input_tensor = Input(shape=(224, 224, 3))
base_model = ResNet50(include_top=False, input_tensor=input_tensor)
x = base_model.output
x = layers.GlobalAveragePooling2D()(x)
x = Dense(256, activation='relu')(x)
# Add a residual block
residual = x
x = Dense(256, activation='relu')(x)
x = Dense(256, activation='relu')(x)
x = add([residual, x])
# Add more layers as needed
# ...
model = Model(inputs=base_model.input, outputs=x)
In this example, after the global average pooling layer and a dense layer, we add a residual block using layers.add. The add function combines the residual tensor with the x tensor, effectively creating a shortcut connection that allows the gradient to flow directly through the residual path.
-
LSTMs
LSTM is a type of recurrent neural network (RNN) architecture that is particularly effective at capturing long-term dependencies in sequential data. In an LSTM model, the layers.add function is used to stack multiple LSTM layers. Here's an example:
from tensorflow.keras.layers import LSTM, Dense, Input
from tensorflow.keras.models import Model
inputs = Input(shape=(timesteps, features))
x = LSTM(64, return_sequences=True)(inputs)
x = LSTM(64, return_sequences=False)(x)
# Add more layers as needed
# ...
outputs = Dense(num_classes, activation='softmax')(x)
model = Model(inputs=inputs, outputs=outputs)
In this example, we add two LSTM layers using layers.add. The first LSTM layer has return_sequences=True to return the sequence output, while the second LSTM layer has return_sequences=False to return the final output. This stacking of LSTM layers allows the model to learn hierarchical representations of the input sequence.
-
GRU
GRU is another type of recurrent neural network architecture similar to LSTM, but with a simplified gating mechanism. In a GRU model, layers.add is used to stack multiple GRU layers. Here's an example:
from tensorflow.keras.layers import GRU, Dense, Input
from tensorflow.keras.models import Model
inputs = Input(shape=(timesteps, features))
x = GRU(64, return_sequences=True)(inputs)
x = GRU(64, return_sequences=False)(x)
# Add more layers as needed
# ...
outputs = Dense(num_classes, activation='softmax')(x)
model = Model(inputs=inputs, outputs=outputs)
Similar to the LSTM example, we stack two GRU layers using layers.add. The return_sequences=True and return_sequences=False arguments control whether the layers return sequence outputs or only the final output.
Conclusion:
The layers.Add operation in TensorFlow provides a simple and efficient way to perform element-wise addition on tensors. By using this operation, we can easily combine tensors and incorporate addition operations into our neural network architectures. Whether you are working on a simple mathematical problem or building complex deep learning models, understanding and utilizing the layers.Add operation is crucial for efficient computation and achieving accurate results in TensorFlow.
If you liked the article do upvote and share!