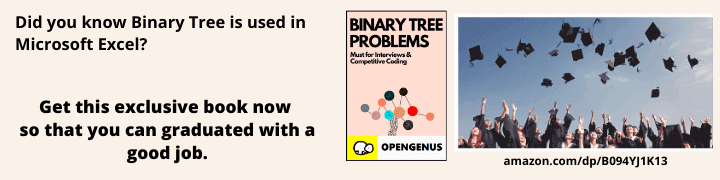
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents:
- Introduction to Django Template System
- Template Syntax and Structure
- Context and Template Inheritance
- Integrating Templates with Models and Views
Introduction to Django Template System
In the context of web development, templates are files or structures that specify the layout, structure, and presentation of a web page. They act as a blueprint or foundation for creating dynamic HTML content that users see.
Templates in Django are usually written in HTML. Django's template system enables developers to construct dynamic web pages by combining HTML markup with dynamic information such as variables, loops, conditions, and template tags.
The primary goal of templates is to isolate the display layer from the backend, in accordance with the Model-View-Controller (MVC). Templates offer a more ordered codebase by keeping HTML markup distinct from backend code.
When user request data from bakcend from appropriate template the Django application receives the request and response to the appropriate template by rendering to the user's browser, which displays the dynamic web page. More on this later.
Template Syntax and Structure
To create dynamic web pages Django Provides as with a powerful template syntax and structures. Those syntaxes, we can use it inside HTML templates after out templates are connected to Django.
Best Practices for Template Structure
To ensure the templates are easily located and rendered within a Django project it worth following specific organization and structure. Here is a common Django template structure:
-
Project-Level Templates:
- It holds templates that provide a consistent layout or functionality throughout the entire project.
- The directory that contains those templates are located at the top lever of the Django project.
- We have to create the directory manualy and is not generated by Django.
-
App-Level Templates:
- Each Django app within your project can have its own templates directory.
- The templates directory within an app holds templates specific to that app.
- By organizing templates within the app directory, it becomes easier to locate and manage app-specific templates.
Here's an example of how the directory structure may look:
In the above structure:
- The project directory represents the root directory of the Django project.
- Each app has its own template directory within it.
- The templates directory at project level contain the project-templates.
Understanding Django template syntax
In the context of Django, template syntax refers to the syntax used in Django's built-in template language.
Here are some key elements of Django's template syntax:
Variables
Variables in Django templates are indeed enclosed in {{ }}
tags, and they represent dynamic data that will be rendered in the template. If you have a Python variable in the Django backend and you want to display its value in a template, you can include the variable name within the {{ }}
tags to display its value.
Before you can use the variable in the template, you need to pass it to the template context. The context is a Python dictionary that contains the data you want to make available in the template.
For example, let's say you want to display a "welcome" message in HTML and include the name of the request user. If you have the user's first name stored as a variable in the backend, your code in the template would look like this:
Welcome, {{ user.first_name }}!
In the above code, user.first_name
is the variable representing the first name of the request user. By enclosing it within the {{ }}
tags, Django will render the value of the user.first_name variable in that position in the template.
The context dictionary would look something like this:
context = {
'user': request.user,
}
Template Tags
Template tags in Django are indeed enclosed in {% %}
and provide control flow and logic within templates. These tags allow us to use high-level language constructs such as if statements and loops directly in the templates.
Examples of commonly used template tags include {% if %}
, {% for %}
, {% block %}
, {% include %}
, and many more.
For instance, let's say we want to display the names of all products in our template. We can use the {% for %}
tag to loop through all the products and display their names. Additionally, we can use the {% if %}
tag to conditionally display products within a certain price range. Here's an example:
{% for product in products %}
{% if product.price >= 10 and product.price <= 100 %}
<p>{{ product.name }}</p>
{% endif %}
{% endfor %}
Template Filters
Template filters are applied to variables or values using |
character. Filters modify the values before they are rendered in the template.
Examples of filters include {{ variable|filter }}
, where filter can be date, uppercase, lower, truncatewords, and more.
Template Comment
Template comments are enclosed in {# #}
and serve as documentation or notes within templates.
For example:
{# this message won't be rendered #}
Context and Template Inheritance
Template Context and Variable
In Djang, template context and variable play a crucial role in connecting the backend with the frontend templates. They allow to pass dynamic data from your Python code to the templates, enabling to render customized content.
Context is data available to the template from the backend. In Django, it is represented as Python dictionary, where as the keys are the variables represented in templates where the values are the corresponding data in the backend.
To include variables in the template, you need to pass the context dictionary when rendering the template. This can be done using the render() function in Django views or by utilizing the context parameter when using class-based views.
Example:
def myView(request):
# Data to be passed to the template
context = {
'name': 'John Smith',
'age': 25,
}
return render(request, 'my_template.html', context)
In the above code we can access John Smith
and 25
using name
and age
variable respectively.
Template Inheritance for Code Reuse
Template inheritance is Django feature that allows you to create reusable templates by defining a base template and extending and overriding specific sections in child templates.
Steps to implement template inheritance are:
-
Create Base Template:
- Base template contains common layout or elements that we want to reuse across multiple templates.
- Inlude
{% %}
tag include elements that can be overriden in child templates. - For example:
<html> <head> <title>Base Template</title> </head> <body> {% block content %} {% endblock %} </body> </html>
In the above code anything that we want to include in the child template will be included in the block content.
-
Create Child Template:
- In each child template, extend the base template using the
{% extends %}
tag and provide the path to the base template. - Override the sections defined in the base template using the {% block %} tags and add content specific to each child template.
- For example:
{% extends 'base.html' %} {% block content%} <p> Child Template <p/> {% endblock content %}
- In each child template, extend the base template using the
The result of this implementation is that the child template inherits the structure and content defined in the base template.
Integrating Templates with Models and Views
In Django, integrating templates with views and models is critical for connecting frontend templates with backend data and logic. This connection enables you to generate dynamic content, manage user interactions, and show structured data from the database.
Here's an overview of MVT(Model View Template) of Django:
By integrating templates with views and models, you can create dynamic web pages that leverage the power of Django's data models, business logic, and template rendering capabilities, enabling the creation of interactive and data-driven applications.