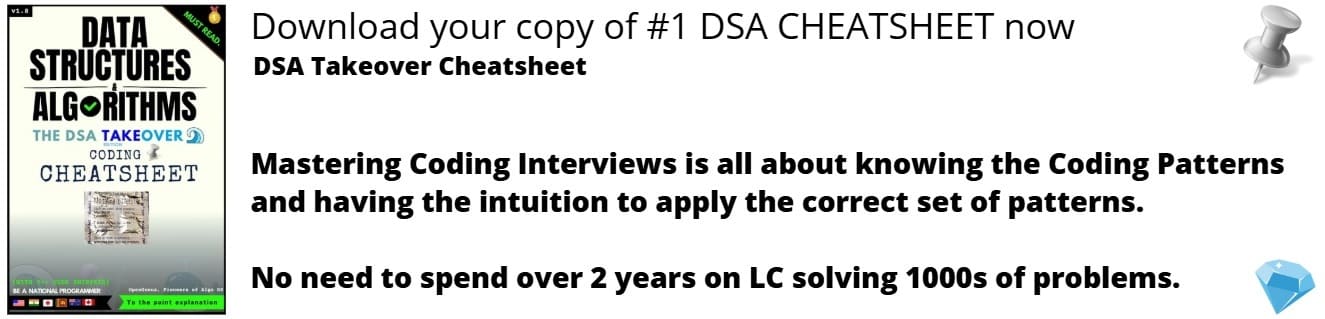
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 5 minutes | Coding time: 5 minutes
aligned_alloc() like malloc() and calloc() is used for dynamic allocation of memory how it differs from the rest is how it enables the user to dynamically allocate the memory as well as allows us to specify the alignment for the newly allocated memory.
Need for aligned memory space.
Speed
The CPU reads at it's word size (4 bytes on 32 bit processor, 8 bytes on 64 bit processor), so if we execute and unaligned address access if the processor supports it. The processor will have to read multiple words for accessing our data This inceases our memory transactions required to access the data.
Range
For any address space if it is know that the two LSB's are always 0(eg. 32 bit machines) it can access 4 times more memory as the 2 saved bits can act as 4 states or the 2 saved bits can be utilised as flags. Taking the 2 LSBs off of an adress gives you 4 byte alignment.
Atomicity
The CPU can operate on an aligned word of memory atomically, meaning that no other instruction can interrupt its opeartion This is critical to many lock free data structures.
Usage
Aligned alloc like malloc is used for dynamic allocation of memory and but we can ask for this memory to be aligned to certain power of two.
#include <stdlib.h>
void *aligned_alloc( size_t alignment, size_t size );
Here alignment is the alignment of the memory. This should be a power of two.
and size is the number of bytes we need to allocate, this should be integral multiple of alignment.
Return value
On success it return a pointer to the newly allocated memory this memory should be deallocated with free() or realloc() to prevent memory leaks.
Upon failure aligned_alloc() return NULL and errno is set to the following values
- EINVAL the value of alignment is not supported or or the value of alignment is not a power of two.
- ENOMEM There was insufficient memory to satisfy the request.
Implementation
#include <cstdio>
#include <cstdlib>
#include <cerrno>
#include <corecrt_malloc.h>
int main()
{
int *x = aligned_alloc(8, 16*sizeof(*x));
if(!p1){
printf("error in allocation of memory", errno);
return -1;
}
else{
printf("memory successfully allocated at", (void*)x);
free(x);
}
return 0;
}
Referneces
Allocating aligned memory blocks by gnu orgAligned alloc man page at ibm docs