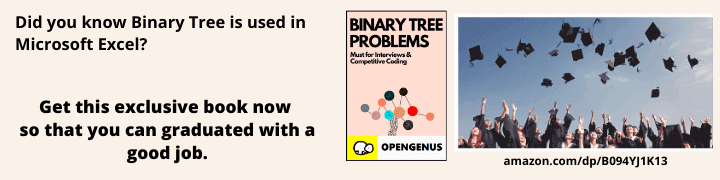
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction to React
React is a free and open-source front-end JavaScript library for building user interfaces based on UI components. React is not a framework, but a js library. React is created and maintained by Facebook.
Why React?
-
React has a component based architecture. This lets the developer to break down the application into small encapsulated components, which can then be composed to make complex UIs.
-
React components also make it possible to write reusable code.
-
React is declarative.
-
React can be integrated with any application.
Pre requisites to learn React:
HTML,CSS,JS,ES6
React Folder Structure
These are the major important folders and files in a React project:
-
package.json:
- This file contains the dependencies and the scripts required for the project.
-
package-lock.json:
- This file ensures consistent installation of the dependencies in the project.
-
node modules(Folder):
- All the dependencies for the project are installed inside this folder.
-
public(Folder):
- This folder contains 3 files. They are:
- manifest.json:
- This file is concerned with Progressive WebApps.
- favicon.ico:
- This is the icon file.
- index.html:
- This is the only html file in the application.
- manifest.json:
- This folder contains 3 files. They are:
-
src(Folder):
- This folder is mostly used during developing the application.
- This folder contains the following files:
- index.js:
- This file is the starting point for a react application.
- App.js:
- This file is responsible for the html displayed in the browser.
- App.css:
- This file is responsible for styling App.js.
- App.test.js:
- This file is responsible for unit tests.
- index.css:
- This file applies styling to the body tag.
- index.js:
When users executes "npm start" in the terminal, the index.html file is served in the localhost.
To start a React project, open the terminal and paste the command:
npx create-react-app my-app
Then do the following steps:
cd my-app
npm start
Now, the project will open in the localhost in your browser and you can see the changes live in the localhost as you add or update the components.
React Components
In React, a component represents a part of the UI. Components are also reusable. Components can also contain other components.
Code for a component is usually placed in a JavaScript file. Component files can also have jsx (JavaScript XML) extension.
Components are of the following types:
- Stateless Functional Component
- JavaScript Functions.
- Stateful Class Component
- Class extending component class.
- must contain a render method returning HTML.
Functional components
Functional components are just JavaScript functions.
They can optionally receive an object of properties (referred to as props) and return html(JSX) which describes the UI.
Example:
// Using ES6 Arrow Function:
const Welcome = (props) => {
return <h1>Hello, {props.name}</h1>;
}
// Using Normal JS Function:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
export default Welcome;
We are exporting the functonal component Welcome so that it can be called wherever needed.
Class components
In React, class components are basically ES6 classes. Similar to a functional component, a class component can also optionally receive props as input and return html(JSX). A class component can also maintain a private internal state.
Example:
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Functional Components vs Class Components
Functional components:
- Functional components are just simple functions.
- Advantage of functional components is the absence of 'this' keyword. The developers are forced to think of a solution without using state.
- Functional components are mainly responsible for the UI (User Interface). That is why they're also called Stateless components / Presentational components.
Class components:
- Class components are more feature rich.
- Class components maintain their own private data - state and they also contain complex UI logic.
- Class components provide lifecycle hooks.
- Class components are also called Statefull / Container components.