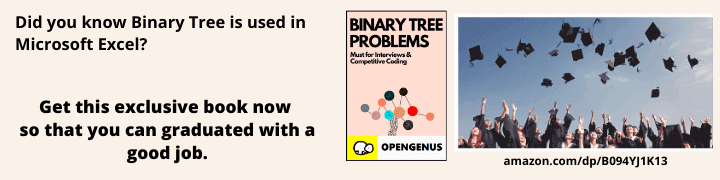
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about Array of Vectors in C++ STL with C++ code snippets. Before getting started with array of vectors we should know about the arrays and vectors in C++.
Array:-
An array is a collection of elements of the same datatype and these elements are stored in contiguous memory locations.We cannot change the size of array once we have declared it.
Creating an Array:
The syntax for declaring an array is:
data_type array_name[array_size];
For example:
int marks[10]; //marks is the name of array of size 10
Vectors:-
Vectors are dynamic arrays which can also be used to store the elements of the same datatype but we can redefine it's size when we perform insertion or deletion in it.
Creating a Vector:
The syntax for declaring a vector is:
vector <data_type> vector_name;
For example:
vector <int> age; //age is the name of the vector
Array of Vectors:-
Array of Vectors is a two dimensional array with a fixed number of rows but there can be any number of columns in each row.
Creating an Array of Vectors:
The syntax for declaring an array of vector is:
vector <data_type> array_name[array_size];
For example:
vector <int> Vec[5]; //Vec is the array of vectors of size 5
Inserting an element in Array of Vectors:-
We can insert an element in array of vectors using push_back() function.
For example:
for(int i=0;i < n;i++){
Vec[i].push_back(12);
}
Traversal:
Since each row contains a vector, we can traverse and access the elements using iterators.
For example:
for(int i=0;i < n;i++){
for(auto it:Vec[i]){
cout<<it<<endl;
}
}
C++ program to illustrate the array of vectors
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vec[5]; //initialising array of vectors vec of size 5
//Inserting elements in array of vectors
int num = 1;
for (int i = 0; i < 5; i++) {
//Inserting elements at every row i using push_back() function in vector
for (int j = 0; j < 5; j++) {
vec[i].push_back(num);
num += 2;
}
}
//printing elements of array of vectors
for (int i = 0; i < 5; i++) {
//Traversing of vectors vec to print the elements stored in it
for (auto itr : vec[i]){
cout<<itr<<" ";
}
cout<<endl;
}
return 0;
}
Output:
1 3 5 7 9
11 13 15 17 19
21 23 25 27 29
31 33 35 37 39
41 43 45 47 49
Question:
Which of the following is the correct syntax of initialising array of vectors?
(a) vector<vector<int>> V;
(b) vector<int> V[N];
(c) int vector<int> V;
(d) None of the above