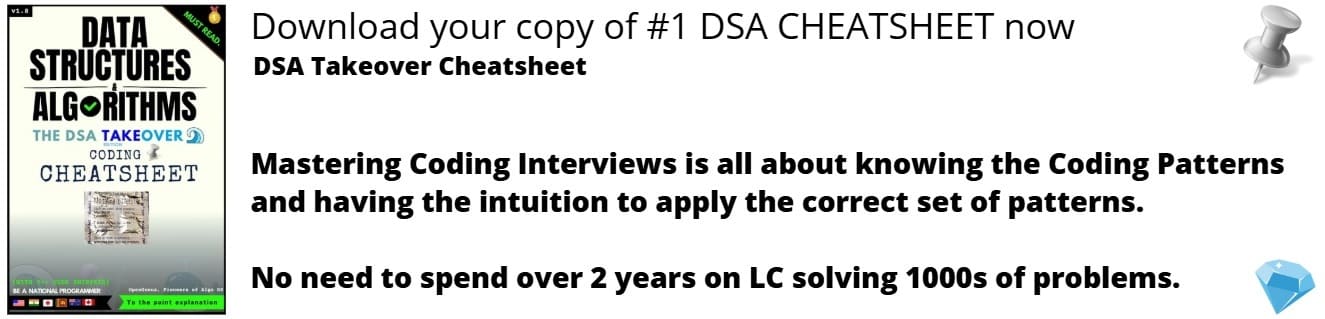
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will develop an approach to find different ways to convert vector to map in C++.
Table of contents::
- what is map?
- what is vector?
- Implementation of map to vector
- Different ways to convert vector to map in C++
- Implementation Using std::copy
- Implementation Using copy constructor
- Implementation Using std::transform
- Applications
- Question
First we need to know what are Vectors and Map
Maps are a part of the C++ Standard Template Library
maps are used to replicate associative arrays.
maps contains sorted key-value pair , in which each key is unique and cannot be changed and it can be inserted or deleted but cannot be altered.
Each element has a key value and a mapped value. No two mapped values can have same key values.
Maps store the key values in ascending order by default.
map<string, int> sample_map;
Vectors are part of the C++ Standard Template Library. vector can provide memory flexibility.
vector being a dynamic array, doesn't need size during declaration
vectors are like arrays that are used to store elements of similar data types. However, unlike arrays, the size of a vector can grow dynamically.
i.e we can change the size of the vector during the execution of a program as per our requirements.
The vector class provides various methods to perform different operations on vectors.
To use vectors, we need to include the vector header file in our program.
#include <vector>
std::vector<T> vector_name;
Vector of maps can be used to design complex and efficient data structures.
Syntax:
Vector of Ordered Map:
vector<map<datatype, datatype> > VM;
Vector of Unordered map:
vector<unordered_map<datatype, datatype> > VUM;
For example,
Suppose we have a map of integer keys and integer values
map<int,int> mp; //mp[key] =value
mp[5] = 11;
mp[8] = 8;
mp[9] = 6;
To store these we need a vector of integer paired with an integer.
vector<pair<int,int>> vec;
//this vec will store the map as:
[[5,11] , [8,8] , [9,6]]
Now let us assume a map of string keys and integer values:
map<string,int> mp1;
mp1["Shivani"] = 500;
mp1["Kumari"] = 100;
mp1["Hacktoberfest"] =400;
To store these we need a vector of string paired with an integer.
vector<pair<string,int>> vec1;
//this vec1 will store the map as:
[["Shivani",500] , ["Kumari",100] , ["Hacktoberfest",400]]
Implementations
Map to vector
To convert a map to vector is to iterate over the map and store the key-value pairs into vector one by one.
Consider the following example for Integer key and integer value
#include <bits/stdc++.h>
using namespace std;
int main() {
map<int,int> mp;
mp[5] = 11;
mp[2] = 16;
mp[9] = 6;
mp[10] = 6;
vector<pair<int,int>> vec;
for(auto i : mp) //inserting map values into vector
{
vec.push_back(make_pair(i.first,i.second));
}
for(auto j : vec)
cout<<j.first<<" : "<<j.second<<endl;
return 0;
}
Output
2 : 16
5 : 11
9 : 6
10 : 6
Consider the another example for String key and integer values
#include <bits/stdc++.h>
using namespace std;
int main() {
map<string,int> mp1;
mp1["Shivani"] = 500;
mp1["Kumari"] = 100;
mp1["Hacktoberfest"] = 400;
vector<pair<string,int>> vec1;
for(auto i : mp1) //inserting map values into vector
{
vec1.push_back(make_pair(i.first,i.second));
}
for(auto j : vec1)
cout<<j.first<<" : "<<j.second<<endl;
return 0;
}
Output
Shivani : 500
Kumari : 100
Hacktoberfest : 400
Different ways to convert vector to map in C++.
Now we will discuss How to convert a vector of pairs to a map in C++
Using std::copy
If you have vectors of pairs, where the first item in the pair will be the key for map, and the second item will be the value associated with that key, you can just copy the data to the map with an insert iterator
#include <iostream>
#include <map>
#include <vector>
int main()
{
std::vector<std::pair<std::string, int>> values = {
{"one", 1},
{"two", 2},
{"three", 3}
};
std::map<std::string, int> map;
std::copy(values.begin(), values.end(), std::inserter(map, map.begin()));
for (const auto &entry: map) {
std::cout << "{" << entry.first << ", " << entry.second << "}" << std::endl;
}
return 0;
}
Output:
{one, 1}
{three, 3}
{two, 2}
Time complexity for std::copy
Worst case: O(n)
Average case: O(n)
Best case: O(n)
Space complexity for std::copy
O(1);
2. Using copy constructor
we can also use the copy constructor to initialize a map from the vector of pairs. This is the shortest way to convert a vector of pairs to a map.
#include <iostream>
#include <map>
#include <vector>
int main()
{
std::vector<std::pair<std::string, int>> values = {
{"one", 1},
{"two", 2},
{"three", 3}
};
std::map<std::string, int> map(values.begin(), values.end());
for (const auto &entry: map) {
std::cout << "{" << entry.first << ", " << entry.second << "}" << std::endl;
}
return 0;
}
Output:
{one, 1}
{three, 3}
{two, 2}
3. Using std::transform
Another option to convert a vector to a Map is using the standard function
std::transform is a function that takesall the elements from one range and applies some functions to those elements then it saves the result to another range.
Here is an example,
#include <iostream>
#include <map>
#include <vector>
#include <algorithm>
int main()
{
std::vector<std::pair<std::string, int>> values = {
{"one", 1},
{"two", 2},
{"three", 3}
};
std::map<std::string, int> map;
std::transform(values.begin(), values.end(), std::inserter(map, map.end()),
[](std::pair<std::string, int> const &p) {
return p;
});
for (const auto &entry: map) {
std::cout << "{" << entry.first << ", " << entry.second << "}" << std::endl;
}
return 0;
}
Output:
{one, 1}
{three, 3}
{two, 2}
Here is another way to use the std::transform algorithm, we can zip two separate vectors of keys and values together into a map. Here’s what the code would look like:
#include <iostream>
#include <map>
#include <vector>
#include <algorithm>
int main()
{
std::vector<std::string> keys = {"one", "two", "three"};
std::vector<int> values = {1, 2, 3};
std::map<std::string, int> map;
std::transform(keys.begin(), keys.end(), values.begin(), std::inserter(map, map.end()),
[](std::string const &s, int i) {
return std::make_pair(s, i);
});
for (const auto &entry: map) {
std::cout << "{" << entry.first << ", " << entry.second << "}" << std::endl;
}
return 0;
}
Output:
{one, 1}
{three, 3}
{two, 2}
Time complexity for std::copy
Worst case: O(n)
Average case: O(n)
Best case: O(n)
Space complexity for std::copy
O(1);
Applications
- Vector of maps can be used to design complex and efficient data structures.
- Eliminating multiple occurrence of values in a list.
- Sorting a list without taking into account multilple occurrences of any value.
Question
** Q.which is optional in declaration of vector?**
- Number of elements
- Type
- Name
- Vector
Answer
The correct option is 1. number of elements
Explanation
The number of elements is optional, An empty vector means the vector which contains 0 elements
That’s all about converting a vector of pairs to a map in C++.