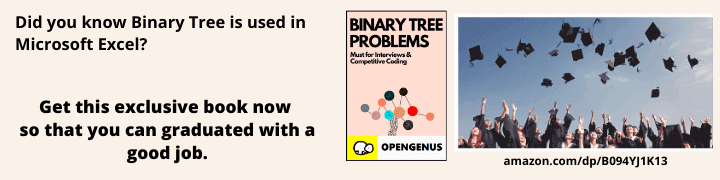
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented different ways to convert an array of uint8_t (uint8_t*) to string in C++.
Table of contents:
- Introduction to array of uint8_t (uint8_t*) and string
- Approach 1: Explicit Casting
- Approach 2: reinterpret_cast
- Approach 3: ostringstream
Introduction to array of uint8_t (uint8_t*) and string
uint8_t is a typedef for char so the compiler treats it as an ASCII character. The size of uint8_t is 8 bits (1 byte) and the size of char is same (1 byte).
String is a special datatype in C++ and is equivalent to an array of characters that is char*.
In this case, we are converting array of uint8_t that is uint8_t*. So, uint8_t* is equivalent to char* or string.
Approach 1: Explicit Casting
As uint8 and char are same, so an array of uint8_t can be converted to an array of char just by casting. Once we get an array of char, assigning it to a string completes the conversion.
uint8_t* input = {12, 1, 65, 90};
char* input2 = (char *) input;
string input3 = input2;
Following is the complete C++ code using explicit casting:
#include <stdlib.h>
int main() {
uint8_t* input = {12, 1, 65, 90};
char* input2 = (char *) input;
string input3 = input2;
return 0;
}
Approach 2: reinterpret_cast
As alternative to explicit casting will be to use reinterpret_cast.
uint8_t* input = {12, 1, 65, 90};
std::string str = reinterpret_cast<char *>(input);
Following is the complete C++ code using reinterpret_cast:
#include <stdlib.h>
int main() {
uint8_t* input = {12, 1, 65, 90};
std::string str = reinterpret_cast<char *>(input);
return 0;
}
Approach 3: ostringstream
ostringstream is a builder where each element from the uint8_t array is appended one by one. Following it, calling the str() method of ostringstream returns the string.
uint8_t* input = {12, 1, 65, 90};
std::ostringstream convert;
for (int i = 0; i < 4; i++) {
convert << (int)input[i];
}
std::string key_string = convert.str();
Following is the complete C++ code using ostringstream:
#include <stdlib.h>
int main() {
uint8_t* input = {12, 1, 65, 90};
std::ostringstream convert;
for (int i = 0; i < 4; i++) {
convert << (int)input[i];
}
std::string key_string = convert.str();
return 0;
}
With this article at OpenGenus, you must have the complete idea of how to convert array of uint8_t (uint8_t*) to string in C++.