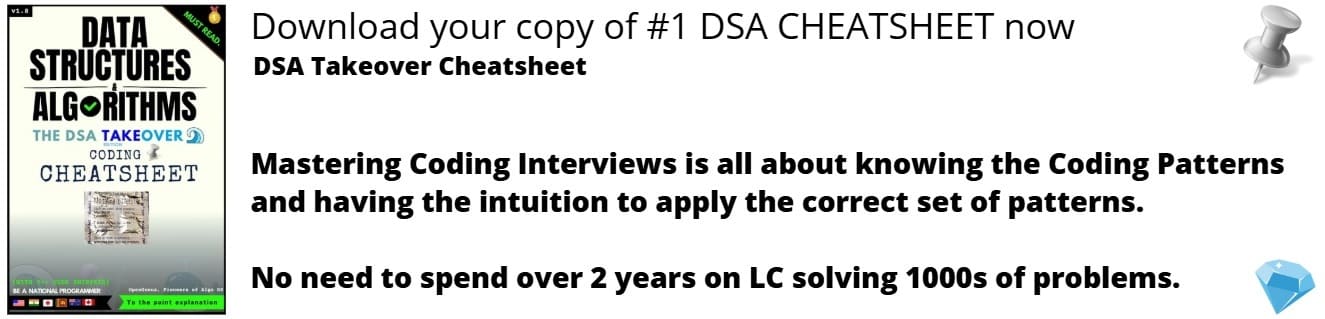
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored different ways of converting vector to string in C++.
Table of contents:
- Using string constructor
- Using String Stream
- Index-based for-loop + String Stream
- Using std:: accumulate
- Using std:: copy
- Using Boost
1. Using string constructor
If the given vector is of type char , we can use the range constructor to construct a string object by copying the specified range of characters to it. Here is an example:
#include<bits/stdc++.h>
using namespace std;
int main()
{ vector<char> vect{'P','Q','R','S'};
string str(vect.begin(),vect.end());
cout<<str<<endl;
return 0;
}
Output:
2. Using String Stream
Another way to convert a vector to string is using the string stream the following c++ program show its implementation :
#include <iostream>
#include <vector>
#include <sstream>
using namespace std;
int main()
{
vector<int> vec { 1 , 2 , 3 , 4 , 5 , 6 , 11 , 23 , -43};
stringstream ss;
for(auto it =vec.begin();it!=vec.end();it++)
{ if(it != vec.begin())
{ ss<<" ";
}
ss<< *it;
}
cout<<ss.str()<<endl;
return 0;
}
Output:
3. Index-based for-loop + String Stream
#include <iostream>
#include <vector>
#include <sstream>
using namespace std;
int main()
{
vector<int> vec { 1 , 2 , 3 , 4 , 5 , 6 , 11 , 23 , -43};
stringstream ss;
for(int i =0;i<vec.size();i++)
{ if(i != 0)
{ ss<<", ";
}
ss<< vec[i];
}
cout<<ss.str()<<endl;
return 0;
}
Output:
4. Using std:: accumulate
Another option to convert a vector to a string is using the standard function std::accumulate, defined in the header numeric. accumulate function usually used for computing sum of given initial values and elements in given range.
We can alter its default operation (sum) and use custom binary predicate to convert given vector to string .
#include <iostream>
#include <vector>
#include <numeric>
using namespace std;
template<typename T>
string join(vector<T> const &vec,string delim)
{
if (vec.empty()) {
return string();
}
return accumulate(vec.begin() + 1, vec.end(),
to_string(vec[0]),
[](const string& a, T b){
return a + ", " + to_string(b);
});
}
int main()
{
vector<int> vec { 1 , 2 , 3 , 4 , 5 , 6 , 11 , 23 , -43 };
string delim = ", ";
string s = join(vec, delim);
cout << s << endl;
return 0;
}
Output:
5. Using std:: copy
Another alternative is the use of std::copy and the ostream_iterator class:
#include<iostream>
#include <iterator>
#include <sstream>
#include <algorithm>
#include <vector>
using namespace std;
int main(){
vector<int> vec { 1 , 2 , 3 , 4 , 5 , 6 , 11 , 23 , -43 };
ostringstream stream;
if(!vec.empty()){
copy(vec.begin(), vec.end()-1, ostream_iterator<int>(stream,", "));
stream << vec.back();
}
cout<<stream.str()<<endl;
return 0;
}
Output:
6. Using Boost
Using the boost::algorithm::join algorithm. It joins all elements in the specified list into a string, where segments are concatenated by a given separator. With Boost and C++11 this could be achieved like this:
#include <bits/stdc++.h>
using namespace std;
int main()
{
vector<int> vec { 1 , 2 , 3 , 4 , 5 , 6 , 11 , 23 , -43 };
string str = ", ";
auto to_string = static_cast<string(*)(int)>(to_string);
cout << boost::algorithm::join(vec | boost::adaptors::transformed(to_string), str);
return 0;
}
note:
for using boost libraries and its function please visit this page and explore boost installation and uses.
With this article at OpenGenus, you must have the complete idea of converting a vector to a string in C++.