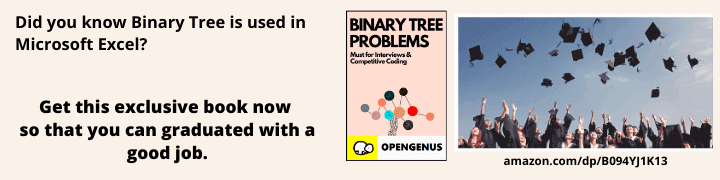
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the reason behind the Python error "<lambda>()
takes 1 positional argument but 2 were given" and have presented multiple ways in which this error can be fixed.
Table of contents:
- Understanding the error
- Fix 1: Basic case
- Fix 2: Error with reduce()
- Concluding Note
Understanding the error
While running a Python code which uses lambda you may encounter the following error:
Traceback (most recent call last):
File "opengenus.py", line 4, in <module>
a = reduce(f, list)
TypeError: <lambda>() takes 1 positional argument but 2 were given
The problem is that the lambda defined in the code takes 1 input parameter but during the invocation of the lambda function 2 input parameters are passed and hence, the error.
The fix will involve:
- Updating the lambda to take 2 inputs
- OR, update the way lambda is called so correct number of input arguments are passed.
There could be other variants of this error when there is a mismatch between the number of parameters expected by the lambda and the number of parameters passed to the lambda. For example:
TypeError: <lambda>() takes 1 positional argument but 2 were given
TypeError: <lambda>() takes 1 positional argument but 2 were given
We will explore how to fix this error.
Fix 1: Basic case
Consider the following Python code which defined a lambda that takes 1 input and adds one to it but while calling the lambda, we are passing two input values. This is the case where the error will come.
f = lambda x: x+1
a = f(4, 3)
print(a)
Run it as:
python code.py
Error:
Traceback (most recent call last):
File "opengenus.py", line 4, in <module>
a = reduce(f, list)
TypeError: <lambda>() takes 1 positional argument but 2 were given
The fix is simple. Call the lambda with one element at a time as the lambda is designed to take 1 input parameter. In some code, lambda can take 2 or more input parameters as well.
The fixed Python code is as follows:
f = lambda x: x+1
a = f(4)
print(a)
Output:
5
Similarly, make sure that the lambda is not called with less number of input parameters than expected by the lambda.
Fix 2: Error with reduce()
One case when the error may come is during the use of reduce(). Reduce() built-in function is frequently used to call a lambda function on repeatedly on each element of an iterable.
Consider the following code:
from functools import reduce
list = [91, 12, 63, 5]
f = lambda x: print(x)
a = reduce(f, list)
Run it as:
python code.py
Error:
Traceback (most recent call last):
File "opengenus.py", line 4, in <module>
a = reduce(f, list)
TypeError: <lambda>() takes 1 positional argument but 2 were given
This issue is reduce() invokes the lambda with two parameters and hence, a fix will be to update the lambda to take 2 input parameters. Consider the following Python code which fixes the issue:
from functools import reduce
list = [91, 12, 63, 5]
f = lambda x, y: print(x, y)
a = reduce(f, list)
Output:
91 12
None 63
None 5
Concluding Note
With this article at OpenGenus, you must have the complete idea of how to fix this error of <lambda>()
takes 1 positional argument but 2 were given.
Always check if the definition of a function and if you are calling the function as expected.