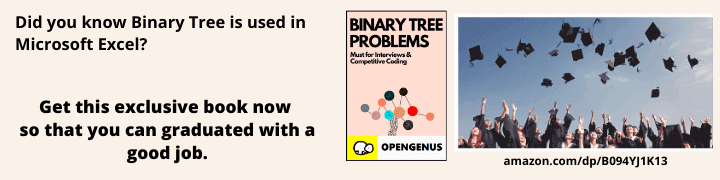
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to use lambda for Minimum() in Python with and without using the min() built-in function. We have explained the concepts using Python code snippets.
Table of contents:
- Python Min Lambda without built-in function
- Lambda in Min() built-in function
- Concluding Note
Go through this article where we find the maximum element using lambda in Python. The overall concept will be same and you can easily leverage it for minimum using lambda in Python.
Python Min Lambda without built-in function
In this following Python code snippet, we have explored how to use Lambda to find the minimum element among two elements.
f = lambda a, b: a if a<b else b
minimum = f(90, 95)
print(minimum)
Output:
90
This does not allow us to find the minimum element in a list but it is possible using lambda.
The idea is that the minimum element in a list of 1 element is the first element. So, we use the lambda recursively to compare the first element and minimum element of the rest of the list.
Following Python code snippet implements this idea of using Lambda to find the minimum element in a list:
f = lambda l: l[0] if len(l) == 1 else l[0] if l[0] < f(l[1:]) else f(l[1:])
list = [59, 47, 7, 17, 45]
minimum = f(list)
print(minimum)
Output:
7
As an alternative, one can use reduce() function in Lambda to find the minimum element in a list:
from functools import reduce
list = [59, 47, 7, 17, 45]
minimum = reduce(lambda x, y: x if x < y else y, list)
print(minimum)
Output:
7
This demonstrates the complete idea of how to use Lambda purely to find the minimum element in a list.
Lambda in Min() built-in function
Python comes with it own min() built-in function which can be used to find the minimum element in a list. In some cases, you need to find the minimum in a custom way. In this case, lambda is used to set the key attribute of min() function.
Consider this case of list of numbers which are represented as strings. In this case, min() will consider the elements as string and compare accordingly.
list = ["59", "47", "7", "17", "45"]
minimum = min(list)
print(minimum)
Output:
17
If you want the min() function to consider the elements are number, one can use lambda in key attribute of min() to support this custom functionality.
The following Python code snippet demonstrates the approach:
list = ["59", "47", "7", "17", "45"]
minimum = min(list, key=lambda x:int(x))
print(minimum)
Output:
7
The output is now correct. Similarly, one can use lambda in the key attribute of min() to find the minimum in any custom way in a list of tuples or custom objects.
Concluding Note
With this article at OpenGenus, you must have the complete idea of how to use lambda to find the minimum element in a list. Lambda in a very powerful approach in Python which you should definitely use whereever possible.