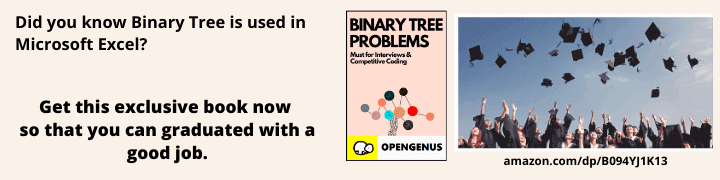
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to use lambda with max() built-in function in Python to find the maximum element in any iterable with custom objects. Additionally, we have presented how to implement max from scratch using lambda without using any built-in function.
Table of contents:
- Creating Max function using Lambda (without built-in function)
- max() built-in function in Python
- Need of Lambda in max()
- Using Lambda in max(): Examples
- Concluding Note
Let us dive into Python Max Lambda with Python code examples along the way.
Creating Max function using Lambda (without built-in function)
In this section, we will use the lambda function to create an in-built maximum function that will find the maximum element between two elements.
Check the following Python code:
f = lambda a, b: a if a>b else b
maximum = f(10, 14)
print(maximum)
In this, lambda takes 2 input numbers (a, b). The statement in lambda is a ternary operator which returns a if a is larger than b or else it returns b.
Output:
14
The output is as expected. The problem will be to take list as input and find the maximum element in it. The challenge is there can be only one statement in lambda and how can we iterate through a list in Python and keep track of the maximum element in one statement.
The solution is to:
- return the first element of the list if the length of the list is 1. If there is only one element, it is the largest element.
- If length of list is greater than 1, then use this lambda recursively.
- Compare the first element l[0] with the maximum element of the rest of the list that is fetched by recursively calling the lambda on l[1:].
- This allows us to fetch the maximum element of a list.
f = lambda l: l[0] if len(l) == 1 else l[0] if l[0] > f(l[1:]) else f(l[1:])
list = [19, 7, 17, 97, 5]
maximum = f(list)
print(maximum)
Output:
97
The output is as expected.
Another alternative will be to use the reduce function in lambda to find the maximum element:
from functools import reduce
list = [19, 7, 17, 97, 5]
maximum = reduce(lambda x, y: x if x >= y else y, list)
print(maximum)
Output:
97
The output is as expected.
This demonstrates how we can write our own lambda to find the maximum element without using the max() in-built function.
max() built-in function in Python
Following is the syntax of the built-in max function in Python:
max(iterable, key)
- Iterable: This is a list of objects whose max we need. This can be any iterable object in Python.
- Key: This is optional and can be used to control how elements in the list are compared to find the maximum element.
- The function returns an object from the iterable that is maximum.
Key can take 5 different things in Python:
- Built in function
- User defined function
- Lambda function / Anonymous function
- Itemgetter
- Attrgetter
In this article at OpenGenus, we have focused on how to use Lambda in Key attribute of max() to find the maximum element of any list in desired way.
Using lambda in max() brings all benefits of lambda along with keep the code short and clear compared to other alternatives like User defined function.
Following is an example of using max() without key attribute:
list = [19, 7, 17, 97, 5]
maximum = max(list)
print(maximum)
Output:
97
It works as expected but this is not the case always. We will see in the next section.
Need of Lambda in max()
Let us consider the case such that the list has numbers but as strings. If we use max function on it, max() will compare the elements as string and not as numbers and hence, the result will not be as expected.
Consider the following Python code using max() in-built function:
list = ["19", "7", "17", "29", "5"]
maximum = max(list)
print(maximum)
We will expect the answer to be 29 as we are considering the data as numbers. As the data is a list of string, max() will return 7.
Output:
7
The fix in this case is to use the key attribute in max() function to control how the elements are compared. We can use the lambda function for key attribute of max() function.
The code using lambda will be as follows:
list = ["19", "7", "17", "29", "5"]
maximum = max(list, key=lambda x:int(x))
print(maximum)
The output will be:
29
In this case, the lambda function is converting each element to its corresponding integer value using the int() in-built function in Python.
Using Lambda in max(): Examples
In this previous section, we saw a specific use case of lambda in the max() in-built function. There are several ways lambda can be used in max() such as:
- If elements in list are in string format but you want to compare them as integers, use lambda to convert each element to its corresponding integer value using int() as part of the key attribute of max().
maximum = max(list, key=lambda x:int(x))
- If there is a list of tuples and you want to find the maximum element based on the second element of the tuple, we can use lambda as follows:
list = [(1,'aditya'), (2,'ue'), (9,'ben'), (5,'ned')]
max(list, key = lambda x: x[1])
- Find maximum element in a list that has a mixture of string and integers by comparing string by converting into integer. By default, max() in-built function will give error in Python3 if applied on this list but on passing the correct lambda in key attribute it will work as expected.
list = ['1', '9', 100, 2]
max(list, key=lambda x: int(x))
- Similarly, you can use lambda in the key attribute of max() in-built function to support any custom object in Python.
Concluding Note
With this article at OpenGenus, you must have the complete idea of how to use lambda to find the maximum element and how to use lambda in the key attribute of max() in-built function in Python.
Lambda is a very feature in Python. Use it to find maximum element in any custom way.