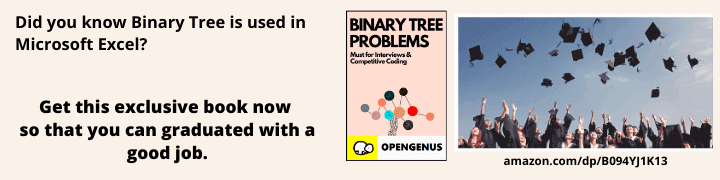
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes
The Iterable interface (java.lang.Iterable) is the root interface of the Java collection classes. The Collection interface extends Iterable interface, so all subtypes of Collection implement the Iterable interface.
This interface stands to represent data-structures whose value can be traversed one by one. This is an important property. Consider the scenaio where you have access to vast amount of data stored in two data-stores S1 and S2.
S1 is iterable while S2 is not. S1 gives you the power to explore the entire universe of data provide you have enough time and energy resources to do so. At the same time, S2 does not provide any means of exploring its contents if you have no knowledge of what to expect.
Depending on a situation, both iterable and not iterable data structures are useful in our Universe.
A class that implements the Iterable can be used with the for-loop. For instance:
List list = new ArrayList();
for(Object obj : list)
{
// You can do anything with your obj
}
Methods
The Iterable interface has only one method (iterator() method):
public interface Iterable<T>
{
public Iterator<T> iterator();
}
User defined classes with Iterable interface
It is possible to use user-defined collection type classes with the for-loop. To do so, the class must implement the java.lang.Iterable
public class MyCollection<E> implements Iterable<E>
{
public Iterator<E> iterator()
{
return new MyIterator<E>();
}
}
Implementation
The implementation of the MyIterator class:
public class MyIterator <T> implements Iterator<T>
{
public boolean hasNext()
{
// You have the power to craft its content
}
public T next()
{
// You can give any power to this function
}
public void remove()
{
// You are the creator of this section
}
}
Usage
Instance of usage:
public static void main(String[] args)
{
MyCollection<String> stringCollection = new MyCollection<String>();
for(String string : stringCollection)
{
// Manage your string carefully
}
}