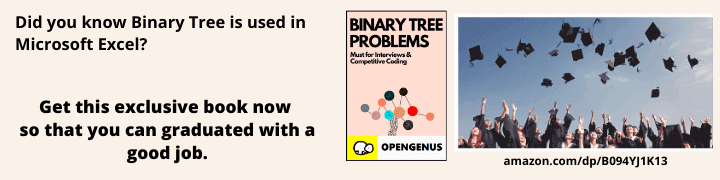
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes
Java LinkedList is an implementation of the List and Deque interfaces. It is one of the frequently used List implementation class.
It extends AbstractSequentialList and implements List and Deque interfaces. It is an ordered collection and supports duplicate elements. It stores elements in Insertion order. It supports adding null elements. It supports index based operations.
Properties
Properties of LinkedList in Java Collection Framework is as follows:
- Java LinkedList interface is a member of the Java Collections Framework.
- It is an implementation of the List and Deque interfaces.
- Internally, it is an implemented using Doubly Linked List Data Structure.
- It supports duplicate elements.
- It stores or maintains itβs elements in Insertion order.
- We can add any number of null elements.
- It is not synchronised that means it is not Thread safe.
- We can create a synchronised LinkedList using Collections.synchronizedList() method.
- In Java applications, we can use it as a List, stack or queue.
- It does not implement RandomAccess interface. So we can access elements in sequential order only. It does not support accessing elements randomly.
- When we try to access an element from a LinkedList, searching that element starts from the beginning or end of the LinkedList based on where that elements is available.
- We can use ListIterator to iterate LinkedList elements.
- From Java SE 8 on-wards, we can convert a LinkedList into a Stream and vice-versa.
- Java SE 9 is going to add couple of factory methods to create an Immutable LinkedList.
Java LinkedList Methods
Some of the useful methods of Linked List is as follows:
-
int size(): to get the number of elements in the list.
-
boolean isEmpty(): to check if list is empty or not.
-
boolean contains(Object o): Returns true if this list contains the specified element.
-
Iterator iterator(): Returns an iterator over the elements in this list in proper sequence.
-
Object[] toArray(): Returns an array containing all of the elements in this list in proper sequence.
-
boolean add(E e): Appends the specified element to the end of this list.
-
boolean remove(Object o): Removes the first occurrence of the specified element from this list.
-
boolean retainAll(Collection c): Retains only the elements in this list that are contained in the specified collection.
-
void clear(): Removes all the elements from the list.
-
E get(int index): Returns the element at the specified position in the list.
-
E set(int index, E element): Replaces the element at the specified position in the list with the specified element.
-
ListIterator listIterator(): Returns a list iterator over the elements in the list.
-
List subList(int fromIndex, int toIndex): Returns a view of the portion of this list between the specified fromIndex, inclusive, and toIndex, exclusive. The returned list is backed by this list, so non-structural changes in the returned list are reflected in this list, and vice-versa.
-
void addFirst(E e): Inserts the specified element at the beginning of this list.
-
void addLast(E e)**==: Inserts the specified element at the end of this list.
-
E getFirst(): Retrieves, but does not remove, the first element of this list. This method differs from peekFirst only in that it throws an exception if this list is empty.
-
E getLast(): Retrieves, but does not remove, the last element of this list. This method differs from peekLast only in that it throws an exception if this list is empty.
-
E remvoeFirst(): Removes and returns the first element from this list.
-
E removeLast(): Removes and returns the last element from this list.
-
boolean offerFirst(E e): Inserts the specified element at the front of this list.
-
boolean offerLast(E e): Inserts the specified element at the end of this list.
-
E pollFirst(): Retrieves and removes the first element of this list, or returns null if this list is empty.
-
E pollLast(): Retrieves and removes the last element of this list, or returns null if this list is empty.
-
E peekFirst(): Retrieves, but does not remove, the first element of this list, or returns null if this list is empty.
-
E peekLast(): Retrieves, but does not remove, the last element of this list, or returns null if this list is empty.
Implementations
Simple usage example of LinkedList class in Java:
import java.util.LinkedList;
import java.util.List;
public class LinkedListDemo
{
public static void main(String[] args)
{
List data = new LinkedList();
data.add("1");
data.add(2);
data.add(3);
data.add("4");
System.out.println("LinkedList content: " + data);
System.out.println("LinkedList size: " + data.size());
}
}
With generics:
import java.util.LinkedList;
import java.util.List;
public class LinkedListGenericsDemo
{
public static void main(String[] args)
{
List data = new LinkedList<>();
data.add("OpenGenus");
data.add("is");
data.add("for");
data.add("you");
System.out.println("LinkedList content: " + data);
System.out.println("LinkedList size: " + data.size());
}
}