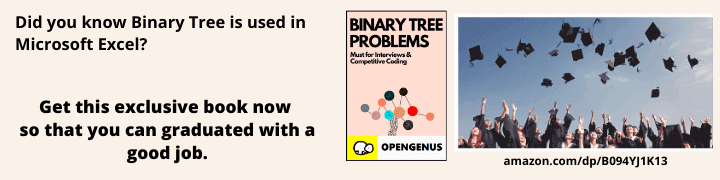
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes | Coding time: 20 minutes
Linked List is an efficient data structure to store data. You can learn about the basics of Linked List data structure in this wonderful post. In this session, we will explore the search operation in a Linked List.
As we discussed previously, any data structure that stores data has three basic operations:
- Insertion: To insert data into the data structure
- Deletion: To delete data from the data structure
- Search: To search for a data in the data structure
In this leason, we will explore the search operation in a Linked List in depth.
The structure of a node in a Linked List is as follows:
The structure of a node in a doubly Linked List is as follows:
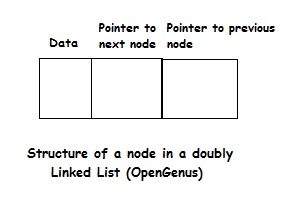
How to search an element in a Linked List?
To search an element in a Linked List, we need to traverse the entire Linked List and compare each node with the data to be search and continue until a match is found. We need to begin the search process from the first node as random access is not possible in a Linked List.
Pseudocode to search an element iteratively:
1) Initialize a node pointer, current = head.
2) Do following while current is not NULL
a) current->key is equal to the key being searched return true.
b) current = current->next
3) Return false
Pseudocode to search an element recursively:
search(head, x)
1) If head is NULL, return false.
2) If head's key is same as x, return true;
2) Else return search(head->next, x)
Why is searching in a Linked List slower than Arrays?
Considering that Linear Search is used in a Linked List and an Array, searching is always slower in a Linked List due to locality of reference. This is due to the following facts:
- How Linked Lists are stored in memory?
- How memory is accessed by the operating system?
Linked Lists are stored randomly (scattered) in memory.
When a data from a particular memory location is required, the operating system fetches additional data from the memory locations that are adjacent to the original memory location. The key idea is that the next memory location to be fetched is likely to be fetched from a nearby location and this has the potential to reduce the memory fetch operations. This concept is known as locality of reference.
As Linked Lists are stored randomly, the next data to be fetched is in a far memory location and hence, locality of reference does not reduce the memory access operations.
As arrays are sequentially, locality of reference plays a great role in making search operations in array faster.
Is binary search possible in Linked List?
Yes, binary search is possible in a Linked List povided the data is sorted.
The problem is that random access is not possible in a Linked List. Hence, accessing the middle element in a Linked List takes liner time. Therefore, the binary search takes O(N) time complexity instead of O(log N) in case of an array.
As binary search has no performance advantage compared to linear search in case of a Linked List, linear search is preferred due to simple implementation and single traversal of the Linked List.
Pseudocode
Pseudocode of searching a node in a Linked List:
//Checks whether the value x is present in linked list
public boolean search(int x)
{
Node current = first; //Initialize current
while (current != null)
{
if (current.data == x)
return true; //data found
current = current.next;
}
return false; //data not found
}
Implementations
Implementation of search operation in a Linked List is as follows:
- Java
Java
import java.util.*;
class LinkedList<E> implements java.io.Serializable
{
private static class Node<E>
{
E item;
Node<E> next;
Node<E> prev;
Node(Node<E> prev, E element, Node<E> next)
{
this.item = element;
this.prev = prev;
this.next = next;
}
}
transient int size = 0;
transient Node<E> first;
transient Node<E> last;
// Creates an empty list
public LinkedList() {}
//Checks whether the value x is present in linked list
public boolean search(int x)
{
Node current = first; //Initialize current
while (current != null)
{
if (current.data == x)
return true; //data found
current = current.next;
}
return false; //data not found
}
// Return Node at index "index" O(N) time
public Node<E> node( int index)
{
if(index < (size >> 1))
{
Node<E> x = first;
for(int i=0;i<index; ++i)
x = x.next;
return x;
}
else
{
Node<E> x = last;
for(int i=size-1; i>index; --i)
x = x.prev;
return x;
}
}
// Print all elements in the LinkedList
public void printList()
{
Node<E> x = first;
for(int i=0;i<size; ++i)
{
System.out.println(x.item);
x = x.next;
}
}
private void LinkFirst(E e)
{
final Node<E> front = first;
final Node<E> newNode = new Node<>(null, e, front);
first = newNode;
if( front == null)
last = newNode;
else
front.prev = newNode;
++ size;
}
private void LinkLast(E e)
{
final Node<E> l = last;
final Node<E> newNode = new Node<>(l, e, null);
last = newNode;
if(l == null)
first = newNode;
else
l.next = newNode;
++ size;
}
// Inserts a node before a non null node succ
private void LinkBefore(E e, Node<E> succ)
{
Node<E> before = succ.prev;
Node<E> newNode = new Node<E>(before, e, succ);
succ.prev = newNode;
if(before == null)
{
first = newNode;
}
else
{
before.next = newNode;
}
++ size;
}
E unlinkFirst(Node<E> f)
{
final Node<E> next = f.next;
first = next;
final E element = f.item;
f.item = null;
f.next = null;
if(next == null)
last = null;
else
next.prev = null;
-- size;
return element;
}
E unlinkLast(Node<E> l)
{
final E element = l.item;
final Node<E> newLast = l.prev;
l.prev = null;
l.item = null;
last = newLast;
if(newLast == null)
first = null;
else
newLast.next = null;
-- size;
return element;
}
E unlink(Node<E> n)
{
final E element = n.item;
final Node<E> before = n.prev;
final Node<E> next = n.next;
if( before == null )
return unlinkFirst(n);
else if(next == null)
return unlinkLast(n);
else
{
n.item = null;
n.next = null;
n.prev = null;
before.next = next;
next.prev = before;
-- size;
}
return element;
}
void add(E e)
{
LinkLast(e);
}
void addAtEnd(E e)
{
LinkLast(e);
}
void addAtFront(E e)
{
LinkFirst(e);
}
}
public class Test_LinkedList
{
public static void main()
{
LinkedList<Integer> ll = new LinkedList<Integer>();
ll.add(10);
ll.add(9);
ll.add(10);
ll.add(100);
Node<Integer> nn = ll.node(3);
ll.unlink(nn);
ll.add(11);
ll.printList();
}
}
Complexity
- Worst case time complexity:
Θ(N)
- Average case time complexity:
Θ(N)
- Best case time complexity:
Θ(1)
- Space complexity:
Θ(1)