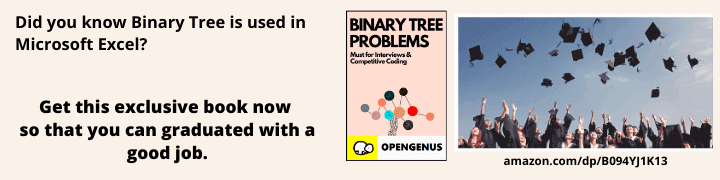
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Definiton of Standard, Mean Deviation and Variance
- Standard Deviation
- Mean Deviation
- Variance
- Java program
- Explanation
In this article at OpenGenus, we will learn to calculate the standard deviation, mean deviation and variance in Java.
Definiton of Standard, Mean Deviation and Variance
Standard Deviaton
A statistical measurement called standard deviation shows how far a set of data is from the mean or average value. It is calculated by taking the square root of the variance, which is the sum of the squared deviations between each data point and the mean. A higher standard deviation implies that the data is more dispersed from the mean, whereas a lower standard deviation suggests that the data is closely concentrated around the mean. The variability or dispersion of a dataset is frequently measured using the standard deviation in disciplines like economics, science, and social sciences.
Standard deviation = square root of ∑(Xi - ų)^2 / N
where,
Xi = each element of the array
ų = mean of the elements of the array
N = Number of elements
∑ = Sum of the each element
Mean Deviation
A dataset's mean deviation, which is a statistical measure, shows the average deviation or difference between each data point and its mean or average value. The absolute differences between each data point and the mean are then averaged out to determine the value. In contrast to standard deviation, which squares the deviations, mean deviation equally weights each data point. Data that deviates more from the mean tends to be more dispersed, whereas data that deviates less from the mean tends to be more tightly grouped. To gauge a dataset's variability or dispersion, mean deviation is frequently used in the domains of statistics, economics, and finance.
Mean Deviation (M.D) = ∑∣X – X̄∣ / N
Where,
∑ – Sum of each element
x – each element of the array
X̄ – Mean of the elemnts of the array
N – Number of elements
Variance
Variance is a statistical measure that indicates how much each data point deviates from the dataset's mean or average value. It is determined by averaging the squared deviations between each data point and the mean. Data that is more dispersed from the mean is indicated by a larger variance, whereas data that is more tightly packed around the mean is indicated by a smaller variance. Engineering, physics, and other disciplines employ variance to quantify a dataset's variability or dispersion.
The Formula for variance is written as
Variance = ∑ (xi – x̄)^2/N
where,
x̄ -> mean of data set(In this case the elemnts of the array).
∑ (xi – x̄)^2 -> sum of squares of difference of each element in the array,
N -> the total number of elements in the array .
Java Program
In this program, we will calculate the mean, variance, and standard deviation of real numbers for the given number of floating-point values.
//Java program to calculate the standard deviation, mean deviation and variance
public class Calculation{
public static void main(String[] args){
double[] arr = new double[] {14.5, 16.5, 17.2, 15.95, 17.89};
double sum = 0;
double mean = 0;
double variance = 0;
double standardDeviation = 0;
double meanDeviation = 0;
int i = 0;
for(i=0;i<5;i++){
sum = sum + arr[i];
}
mean = sum/5;
//summation for standard deviation
double sumSd = 0;
for(i=0; i<5; i++){
sumSd = sumSd + Math.pow((arr[i]-mean),2);
}
variance = sumSd/5;
standardDeviation = Math.sqrt(variance);
//summation for mean deviation
double sumMd = 0;
for(i=0; i<5; i++){
sumMd = sumMd + (Math.abs(arr[i]-mean));//to ignore the negative number
}
meanDeviation = sumMd/5;
System.out.printf("Standard Deviation: %.2f\n",standardDeviation);
System.out.printf("Mean Deviation: %.2f\n ",meanDeviation);
System.out.printf("Variance: %.2f\n",variance);
}
}
Output
Standard Deviation: 1.16
Mean Deviation: 0.95
Variance: 1.34
Explanation
- First, create a class called Calculate
- Then create the main method and then in the main method create an object of the above class and call it using the object.
- Then declare an array in this class with the values given in the above example.
- Now, to iterate through the array, we need to find the size of the array.
- Now, use the for-loop and iterate through this array and increment it by 1 as we need to print all the elements of the array.
- Then, again use the for-loop and iterate through the array to calculate the sum of the elements of the array.
- After that, the mean will be calculated by mean = sum / 5, where 5 is the number of elements in the array(N).
- The variable containing the sum for standard deviation is initialized
- Variance is calculated by dividing the sum from the standard deviation by the number of elements in the array
- Now, the standard deviation and variance will be calculated with the help of mean, which is done by iterating through the for-loop created and with the help of Mathematical predefined methods like Math.pow and Math.sqrt.
- The variable containing the sum for Mean deviation is initialized
- Now, the mean deviation will be calculated with the help of mean, which is done by iterating through for-loop again and with the help of Mathematical predefined methods like Math.abs (This is because the negative(-)sign of values during the subtraction of the mean from each element of the array is to be ignored).
- After that, the value will be returned by that class and then print.(printf is used to format the floating point numbers)
With this article at OpenGenus, you must have the complete idea of how to implement a Java program to compute Standard Deviation, Mean Deviation and Variance.