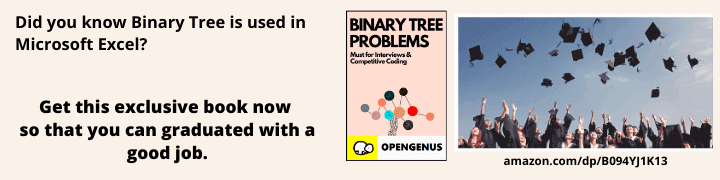
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Amazon Hub Locker Service is a convenient and secure delivery option that allows customers to pick up their packages from self-service lockers located in various public places. Some of these place include shopping centers, convenience stores, and apartment buildings. In this article at OpenGenus, we will take a look on the key design features of the Amazon Hub Locker Service.
Note: Amazon and the Amazon logo are trademarks of Amazon.com, Inc. or its affiliates.
Table of Contents
- How Amazon Hub Lockers work
- Functional requirements
- Non-functional requirements
- Software requirements
- System architecture
- Backend design
- Database design
- Security
- UI design
How Amazon Hub Lockers work
Amazon Hub Locker Service is a secure, self-service delivery option that allows customers to pick up their packages from lockers located in various public places. The service is designed to provide a convenient, secure, and flexible delivery option for customers who are unable to receive packages at their homes or workplaces.
Functional Requirements
To be functional, the locker should at least have the following features:
- Locker Management: The system should allow the management of lockers, including adding new lockers, removing lockers, and assigning lockers to specific locations.
- Package Management: The system should allow the management of packages, including scanning and storing package information, notifying customers of package availability, and tracking package delivery.
- User Management: The system should allow the management of user information, including user registration, user authentication, and user authorization.
- Payment Processing: The system should allow payment processing for package storage and late fees.
Non-functional Requirements
- Usability: The system should provide an interactive, user-friendly interface that is easily understandable for all users.
- Availability: The system should be available 24/7, with minimal downtime for maintenance and updates.
- Dependability: The system should provide consistent performance, with easy tracking of package records and updating of package status.
- Maintainability: The software should be easily maintainable, with minimal downtime for updates and bug fixes.
- Security: Only authorized users must be able to access the system and view and modify package data. The system should also be secure against hacking attempts and unauthorized access.
Software Requirements
- A server running Windows Server/Linus OS
- A backend language such as Java or Python to process orders and functionality
- Front-end frameworks, like Angular/React/Vue for the user interface
- Relational DBMS such SQL
System Architecture
The Amazon Hub Locker Service followers a simple 3-tier client/server architecture. The client can use web browsers or mobile applications to access the system (locker information and package status) through the Internet using the HTTPS protocol.
The middle tier, which includes the server, presents the website to the user and controls the business logic. It controls the interactions between the application and the user. The server also sends package information to the locker hardware, which unlocks the locker containing the package for the customer. Common web server technology used here can be Apache, Nginx, etc.
The data tier maintains the application's data such as locker data, package data, and user data. It stores these data in a relational database management system (RDBMS) like PostgreSQL. The client is able to retrieve their many points of data from this system, as well as make modifications to the data.
Backend Design
Here is some very basic code on a general design of the locker system in a Python backend:
class Locker:
def __init__(self, locker_id, location, size):
self.locker_id = locker_id
self.location = location
self.size = size
self.is_available = True
self.package = None
def set_package(self, package):
if self.is_available:
self.package = package
self.is_available = False
else:
print("Locker is not available")
def remove_package(self):
if not self.is_available:
package = self.package
self.package = None
self.is_available = True
return package
else:
print("Locker is already empty")
In this class, a locker has various properties such as a locker number, location of the locker, if the locker is available, and size of the locker.
Then, there are two simple functions outlining the basic functionality of the lockers - one to set the package into the locker and close it, and another to remove the package and make it empty again.
class Customer:
def __init__(self, name, email, phone_number):
self.name = name
self.email = email
self.phone_number = phone_number
self.packages = []
def add_package(self, package):
self.packages.append(package)
def remove_package(self, package):
if package in self.packages:
self.packages.remove(package)
def get_package_status(self, package_id):
for package in self.packages:
if package.id == package_id:
return package.status
return "Package not found"
In this class, a customer has various properties such as a name, email, phone number, and a list of packages.
There are functions to add a package, remove a package, and also get the status of a package.
class Package:
def __init__(self, tracking_id, weight, dimensions, recipient):
self.tracking_id = tracking_id
self.weight = weight
self.dimensions = dimensions
self.recipient = recipient
self.delivery_status = "In transit"
self.pickup_status = "Not yet picked up"
self.pickup_hub = None
def get_tracking_id(self):
return self.tracking_id
def get_weight(self):
return self.weight
def get_dimensions(self):
return self.dimensions
def get_recipient(self):
return self.recipient
def get_delivery_status(self):
return self.delivery_status
def get_pickup_status(self):
return self.pickup_status
def set_pickup_status(self, status):
self.pickup_status = status
def set_delivery_status(self, status):
self.delivery_status = status
def set_pickup_hub(self, hub):
self.pickup_hub = hub
Finally, there is a package class that stores a tracking id, weight, dimensions, recipient, delivery states, pickup status, and pickup hub location for a specific package.
The functions for this class are just a couple simple getters and setters
Of course, this is a very simple model and the actual code for an Amazon Hub Locker would be much more complex and extensive. For example, you could define a lot of other functions and data for the customers, packages, and hub locations, and you could implement a lot of other functionality for the lockers.
Database Design
For storing the data of the locker, here is a low-level design of the database using SQL:
CREATE TABLE lockers (
locker_id INT PRIMARY KEY,
location VARCHAR(255),
status VARCHAR(255)
);
CREATE TABLE packages (
package_id INT PRIMARY KEY,
locker_id INT,
delivery_status VARCHAR(255),
FOREIGN KEY (locker_id) REFERENCES lockers(locker_id)
);
CREATE TABLE customers (
customer_id INT PRIMARY KEY,
locker_id INT,
FOREIGN KEY (locker_id) REFERENCES lockers(locker_id)
);
Security
In order to maintain security and ensure that only the right customer takes a package from the Amazon Hub Locker system, you could possibly implement the following measures:
- Authentication: a customer should be able autheticate themselves with a username/password at the locker or inside the app when they arrive at the locker location.
- Verification: Before and after a customer picks up their package, they can scan a specific QR code or provide biometric data to their app.
- Encryption: Data should be stored safely and must have security measures to access in order to make sure that all customer data is secure.
- Security audits: A system should keep a record of all activities of the locker system to identify breaches, have a history of breaches, and maintain the security of their system.
UI Design
The system should have a user interface for an separate app for customers and delivery drivers. On the customer side, the app should allow them to see what locker their package is stored in and let them open the locker when they want to retrieve it. On the delivery side, an application can be useful to know which lockers to put packages in and confirm the delivery of these packages into the lockers.
Thus, the UI for a customer should at least have information about their package, as well as buttons and options affecting their order and package within the locker. The UI for a delivery driver and include diagrams of the locker locations and buttons to confirm various deliveries.
Conclusion
In conclusion of this article at OpenGenus, the low-level design for the Amazon Hub Locker service includes several hardware and software components. Essentially, there will be a physical locker location that uses locks and sensors in conjunction with the software, which consists of a backend server to manage package, locker, and customer information, a database to store the data, and a user interface for customers and delivery drivers. With this design, Amazon can provide a more secure and convenient way for peope to pick up packages at their own discretion.