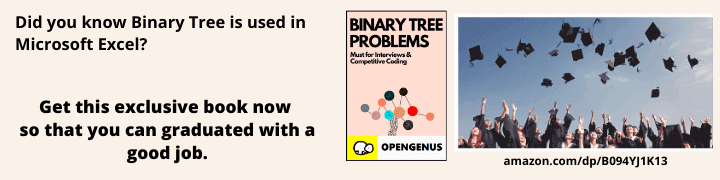
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will list important and unique project ideas that have algorithmic approaches behind them.
Table of contents:
The different Algorithm and Data Structure Project Ideas are:
- Snake Game
- Sudoku
- To-Do list
- Social Media Network
- Phonebook
- Library Management System
- Maze
- Music Playlist
- Calendar
- Student Grade Checker
- Flight Route Planner
- Spell Checker
- Web Crawler
- File Compression Tool
- Real-Time Trafic Analysis
- Shopping Cart App
- Word Frequency Counter
- Online Bookstore
- Decision Support System
- Banking System
- Tic-Tac-Toe
- Memory Matching Game
- Tower of Hanoi Puzzle
- Crossword Puzzle
- Hangman
- Real Estate Property Search
- Email Spam Filter
1) Snake Game
The Snake Game is a popular game where the user controls the snake's movement and can not crash into the wall or to itself. The snake is increasing in size by eating items on the game board.
We can implement the game using a grid for the game board and linked lists where the snake's head can be the head (first node) and the snake's tail can be the tail (last node). When the snake eats an item then we can add an extra node to the head or the tail and increase the size of the list by one.
- Project title: Snake Game
- Algorithms/DS involved: linked list
- GitHub link: https://github.com/skrishnan2001/Snake-Game
- Difficulty rating: 3
2) Sudoku
I like Sudoku games because it involves thinking. In this game, the user has to put a number between 1-9 in one of the cells, however, the same number can not appear twice in the same row, column, and 3x3 grid as well.
To implement this game, we can use a grid (2D array) for the game board and backtracking for the logic. By using this approach, we can explore different possible combinations of numbers until a valid solution is found.
- Project title: Sudoku
- Algorithms/DS involved: backtracking, 2D array
- GitHub link: https://github.com/nibble-4bits/Sudoku-Solver-Visualizer
- Difficulty rating: 5
3) To-Do List
This project idea is great for beginner developers because here, we can also implement CRUD (Read-Create-Update-Delete) operations as well.
One of the ways to create a to-do list is using a stack data structure. This data structure follows the LIFO (last in - first out) method, so when we add a new task in our list, it will be on the top of the older tasks. For example, when removing a task, let's say we have task 1 (bottom), 2 (middle) and 3 (top) and we want to remove task 2, then task 3 will now be on top of task 1, so the order of their addition will remain.
- Project title: To-Do list
- Algorithms/DS involved: stack
- GitHub link: https://github.com/virag-ky/To-Do-List
- Difficulty rating: 1
4) Social Media Network
If you want to create a social meda network project or something similar to this, then the best approach would be to use graphs. Each person would represent a node (vertex) and the relationships between them would be represented as edges. This relationship between them can be friendships, follows, likes, or comments.
- Project title: Social Media Network
- Algorithms/DS involved: graph
- GitHub link: https://github.com/mgmacias95/TwitterFriends
- Difficulty rating: 4
5) Phonebook
Creating a phonebook project is also beginner friendly. Here, we can use an array to store objects that hold the people's information, for example, MongoDB database is using a JSON-like format (like objects) so we can store the information in this format:
{
people: [
{
name: "John",
number: "40-234-456",
},
{
name: "Jane",
number: "30-123-234"
}
]
}
- Project title: Phonebook
- Algorithms/DS involved: array, object
- GitHub link: https://github.com/virag-ky/FullStackOpen-Part3
- Difficulty rating: 1
6) Library Management System
A library management system helps libraries manage and organize their resources (books, newspapers). To implement this type of project, we can use a hash table where we can represent the books and their information with key-value pairs. With this data structure, we can efficiently store and retreive the key-value pairs and reduce the time complexity compared to other data structures.
- Project title: Library Management System
- Algorithms/DS involved: hash table
- GitHub link: https://github.com/haroonrashid2210/Library-Management-System
- Difficulty rating: 3
7) Maze
There are many ways to create maze games, we can create a maze generator only (which just generates the maze) or we can create a fully functional maze game where we can control a sprite for example to navigate through the maze.
To create the maze itself we can use a graph and for the maze navigation (to find the shortest path from the entrance to the exit) we can use the breadth-first search (BFS) algorithm. While using this algorithm, we can keep track of the parent node of each visited node. This allows us to reconstruct the path from the exit back to the entrance once the destination is reached.
- Project title: Maze
- Algorithms/DS involved: graph, breadth-first search (BFS)
- GitHub link: https://github.com/btschneid/Pathfinding-and-Sorting-Visualizer
- Difficulty rating: 5
8) Music Playlist
To create a music playlist project we can use doubly linked lists. Each node would represent a music track. The data part of the node can represent the information of the track, for example, the title, artist, etc. The previous part of the node can represent the previous track and the next part of the node can represent the next track in the list.
- Project title: Music Playlist
- Algorithms/DS involved: doubly linked list
- GitHub link: https://github.com/snehaa2001/Music-playlist
- Difficulty rating: 3
9) Calendar
Building a calendar is another beginner friendly project. Here, we can store the days of the week and the months in arrays, and then create the 12 calendars using a for loop or a forEach() method and some DOM manipulation.
const daysOfWeek = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'];
const months = [
'January',
'February',
'March',
'April',
'May',
'June',
'July',
'August',
'September',
'October',
'November',
'December',
];
// Create 12 tables
months.forEach((month) => {
const div = document.createElement('div');
div.setAttribute('class', 'calendars');
div.innerHTML = ` <table>
<thead>
<tr>
<th class="year-display" colspan="7">
<p>
<span id=${month}-span class="month-span">${month}</span>
</p>
</th>
</tr>
<tr id=${month} class="weeksContainer"></tr>
</thead>
<tbody id=${month}-days class="daysContainer"></tbody>
</table>`;
mainContainer.appendChild(div);
// Create the days of the week
const weekRow = document.getElementById(`${month}`);
daysOfWeek.forEach((day) => {
const dayHeader = document.createElement('th');
dayHeader.textContent = day;
dayHeader.setAttribute('class', 'week');
weekRow.appendChild(dayHeader);
});
createDays(month);
setDays(month);
});
- Project title: Calendar
- Algorithms/DS involved: array
- GitHub link: https://github.com/virag-ky/calendar-in-js
- Difficulty rating: 1
10) Student Grade Checker
A student grade checker project could use a hash table to store and retrieve student grades efficiently. Since this data structure stores key-value pairs, the keys could be the student's name or ID and then the value could be the grades. We could also implement functions to insert or delete grades from the table.
- Project title: Student Grade Checker
- Algorithms/DS involved: hash table
- GitHub link: https://github.com/cameronroudebush/Student-Hash-Table-Database
- Difficulty rating: 3
11) Flight Route Planner
A flight route planner project determines the most efficient routes for flights between different airports. Using graphs in this project is really helpful, the airports could be represented as nodes and the flights between them could be represented as edges (the connections between the nodes).
- Project title: Flight Route Planner
- Algorithms/DS involved: graph
- GitHub link: https://github.com/neo4j-graph-examples/airport-routes
- Difficulty rating: 3
12) Spell Checker
A spell checker project helps identify and correct spelling mistakes in words. We can implement it with a data structure called, trie. This data structure is often used for efficient retrieval of words/strings. By using this data structure, we can efficiently store a dictionary of words and provide suggestions for misspelled words.
- Project title: Spell Checker
- Algorithms/DS involved: trie
- GitHub link: https://github.com/Asylumrunner/TrieSpellChecker
- Difficulty rating: 3
13) Web Crawler
Web crawlers explore the internet and gather information from websites. It starts with a URL then it follows the links from the page to visit other pages. Here, we can use a queue data structure to store the visited websites. The easiest algorithm to use would be breadth-first search so that the crawler visits all the links from the current website first before moving on to other websites.
- Project title: Web Crawler
- Algorithms/DS involved: queue, breadth-first search (BFS)
- GitHub link: https://github.com/sanmak/queue-web-crawler
- Difficulty rating: 5
14) File Comprassion Tool
A heap can be used to optimize the compression process. A heap is a data structure that allows efficient retrieval of the smallest/largest element in a collection. In this case, a heap can be used to store and manage frequency counts of characters in the input file.
- Project title: File Comprassion Tool
- Algorithms/DS involved: heap
- GitHub link: https://github.com/AnshulRanjan2004/File-Compression-Utility
- Difficulty rating: 5
15) Real-Time Traffic Analysis
With this project we can analyze and monitor traffic data in real time. The data can be collected from sensors, cameras, or GPS devices. Segment trees can be used to efficiently process and analyze traffic data. Once the data is collected and stored in the segment trees, we can make queries to retrieve information (for example, the average speed at a certain time interval).
- Project title: Real-Time Traffic Analysis
- Algorithms/DS involved: segment tree
- GitHub link: https://github.com/take-five/segment_tree
- Difficulty rating: 5
16) Shopping Cart App
It's quite easy to implement a shopping cart app with arrays. The shopping cart acts as a temporary container for the items that users wants to purchase.
- Project title: Shopping Cart App
- Algorithms/DS involved: array
- GitHub link: https://gist.github.com/zc0rp10/9bb609a19122a95c9d0bed64b7382b9c
- Difficulty rating: 1
17) Word Frequency Counter
With this project we can count the frequency of each word in a text. We can use a hash table to efficiently store and retreive the key-value pairs. First, we would split the words into tokens (for example, split it with whitespace), then we can iterate over them and for each token we can compute a hash value using a hash function. If the token already exists in the table, then we can increment the frequency value by one.
- Project title: Word Frequency Counter
- Algorithms/DS involved: hash table
- GitHub link: https://github.com/CodeRuben/Word-Frequency-Counter
- Difficulty rating: 2
18) Online Bookstore
Using a binary search tree (BST) for this bookstore app, we can efficiently handle operations like searching, inserting, deleting, and updating books. The books will be stored in a binary search tree based on a specific key (for example, book title).Each node could represent a book and contains information of the book (title, author, etc).
- Project title: Online Bookstore
- Algorithms/DS involved: binary search tree (BST)
- GitHub link: https://github.com/rajvipatel-223/Library-Management-System-Searching-catalogues-in-library-using-binary-search-tree
- Difficulty rating: 4
19) Decision Support System
A decision support system project uses decision tree algorithms. With decision trees we can organize the data in a tree-like structure where each node can represent a decision based on a feature, and each leaf node can represent a result.
- Project title: Decision Support System
- Algorithms/DS involved: tree
- GitHub link: https://github.com/milaan9/Python_Decision_Tree_and_Random_Forest
- Difficulty rating: 5
20) Banking System
In a banking system project we usually have customer accounts that need to be stored and managed. We can handle these accounts using linked lists. Here, each account can be represented with a node and the data property of the node would hold the information of the accounts (for example, name, account number, balance, etc).
- Project title: Banking System
- Algorithms/DS involved: linked list
- GitHub link: https://github.com/zea17/dragon-bank
- Difficulty rating: 2
21) Tic-Tac-Toe
To implement the Tic-Tac-Toe game board, we can use a 2D array data structure (matrix). Each of the cell in the matrix will represent a position on the game board. Typically the board is a 3x3 grid. There are 2 players, one with the X and one with the O. Using the matrix we can easily visualize and manipulate the state of the game board.
[ ['X', '-', '-'],
['-', 'O', '-'],
['-', '-', '-'] ]
- Project title: Tic-Tac-Toe
- Algorithms/DS involved: 2D array/matrix
- GitHub link: https://github.com/thomaspttn/Tic-Tac-Toe
- Difficulty rating: 3
22) Memory Matching Game
This is a fun game to play and one of the ways we can implement it is using linked lists. Each node would represent a card and they would have their information (the card value and a property that would indicate if the card has been flipped or not) stored in the node as well. When two cards are flipped, then we can check if they have the same values and if they have the same "flipped" property (flipped: true). If we found a match then we can remove them from the list.
- Project title: Memory Matching Game
- Algorithms/DS involved: linked list
- GitHub link: https://github.com/virag-ky/OpenGenus/blob/main/data-structures/memoryMatchingGame.js
- Difficulty rating: 2
23) Tower of Hanoi Puzzle
Tower of Hanoi is a puzzle game where we have to move one disk from a peg to another peg. We can use a stack to store the disks. Usually, there are 3 pegs and the third one is a helper peg, so we can easily move all the stack from the starting peg to the destination peg. We can only move one disk at a time and we can't place a larger disk on top of a smaller one. For the logic, we can use a recursive algorithm.
- Project title: Tower of Hanoi Puzzle
- Algorithms/DS involved: stack, recursion
- GitHub link: https://github.com/funoctis/Tower-of-Hanoi
- Difficulty rating: 3
24) Crossword Puzzle
We can represent the crossword puzzle with a 2D array (matrix). The puzzle is basically a grid which contains both horizontal and vertical lines. The players have to fill in the squares with letters following some instructions and the goal is to form full words.
+---+---+---+---+---+
| . | . | . | . | . |
+---+---+---+---+---+
| . | A | . | R | E |
+---+---+---+---+---+
| . | . | . | A | . |
+---+---+---+---+---+
| . | B | . | L | . |
+---+---+---+---+---+
| . | . | . | E | . |
+---+---+---+---+---+
- Project title: Crossword Puzzle
- Algorithms/DS involved: 2D array/matrix
- GitHub link: https://github.com/HartasCuerdas/xwHelper
- Difficulty rating: 2
25) Hangman
This is a popular game and one way to implement it is using linked lists. In this game one player thinks of a word and the other player tries to guess the word one letter a time. The word can be the list itself and each node would be each letter of the word. This data structure allows efficient insertion and deletion of nodes, so during the game it's suitable for dynamically managing the word.
- Project title: Hangman
- Algorithms/DS involved: linked list
- GitHub link: https://github.com/TAGC/Hangman/tree/master
- Difficulty rating: 2
26) Real Estate Property Search
By using R-tree indexing, a real estate property search project can efficiently handle spatial queries and provide users with relevant property listings based on their search criteria and geographic location. First, we can collect real estate property data like, location, price, area, number of bedrooms, etc, then we can build an R-tree index to organize the property data spatially. Each property will be represented by a spatial object, the R-tree will arrange these objects in a hierarchical structure, allowing for efficient spatial queries.
- Project title: Real Estate Property Search
- Algorithms/DS involved: R-tree
- GitHub link: https://github.com/StefanSalewski/RTree
- Difficulty rating: 5
27) Email Spam Filter
We can build an email spam filter project using Bloom filters to efficiently identify and filter out spam emails based on known spam patterns and characteristics. First, we can collect data of known spam email addresses, domains, or keywords that are commonly associated with spam emails. After that we can initialize a Bloom filter with an appropriate size and number of hash functions, then we add the known spam patterns from the data we collected into the Bloom filter. Each pattern is hashed by the selected hash functions and the corresponding bits in the Bloom filter are set to 1. When a new email arrives,the email address or the domain can be hashed using the same hash functions. Then, the filter will determine if any of the corresponding bits are set to 1, if all bits are set to 1, then the email is considered as a spam.
- Project title: Email Spam Filter
- Algorithms/DS involved: Bloom filter
- GitHub link: https://github.com/fareespatel/Spam-classification-using-Bloom-Filter
- Difficulty rating: 5
Conclusion
These were just a few project ideas and their implementation methods, of course, there are many ways to implement each of these projects by using different data structures, algorithms or programming languages.
Feel free to try out some of them if you want to practice DSA and you can go by difficulty levels, starting from number 1 which is the easiest.