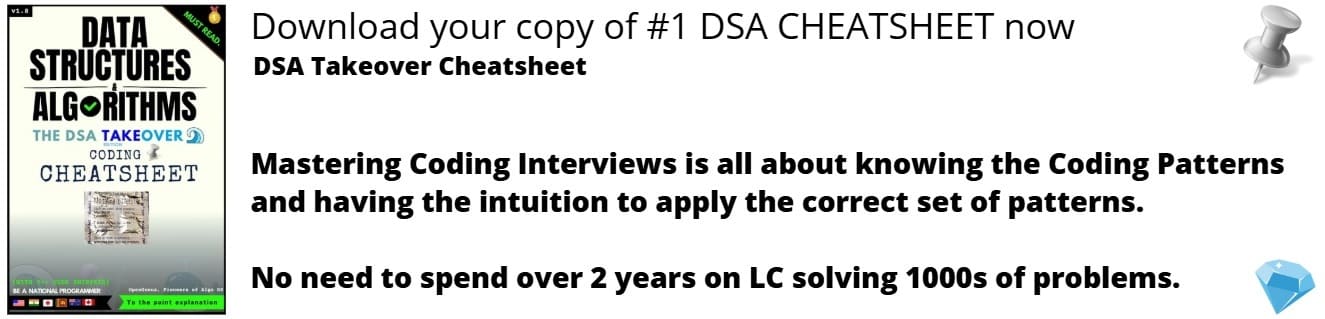
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we explore how we can use K6 to perform various kinds of testing on web servers specifically Load, Spike and Stress Testing Web Servers with K6.
Table of contents:
- Introduction to K6
- Load Testing with K6
- Spike Testing with K6
- Stress Testing with K6
- Circumstances to consider when classifying the performance of your web server
Let us get started with Load Testing, Spike Testing, and Stress Testing Web Servers with K6.
Introduction to K6
K6 is an open source testing tool for making web server performance tests.
Working: K6 works by creating a bunch of virtual users, which run javascript. They send out requests to the web server and record the responses which are dumped as outputs on the console. These can be configured from the js scripts. They are completely configurable and customizable when handling requests. Different endpoints can be tested, the virtual users code can make HTTP requests.
Install K6 on MacOS
brew install k6
Install K6 on Windows
choco install k6
Load Testing with K6
Load testing is primarily concerned with accessing the current performance of your system in terms of concurrent users or requests per second.
Aim for Load Tests
- Asssess the current performance of your typical and peak load.
- Make sure you are continuously meeting teh performance standards as you make changes to your system.
Loading Testing Script with K6
This code sample will allow requests to move from 1 to 100 requests over 5 mins, stay at 100 requests for 10 mins and finally ramp-down to 0 users.
import http from 'k6/http';
export let options={
stages:[
{duration:'5m', target:100},//move from 1 to 100 requests over 5 mins
{duration:'10m', target:100},//stay at 100 requests for 10 mins
{duration:'5m', target:0},// ramp-down to 0 users
]
}
export default function () {
var url = "http://localhost:4000/api/v1/auth/login";
var payload = JSON.stringify({
email: 'admin@gmail.com',
password: '123456',
});
var params = {
headers: {
'Content-Type': 'application/json',
},
};
http.post(url, payload, params);
}
Spike Testing with K6
Spike testing is a variation of stress testing instead of increasing the load gradually, it spikes to extreme load over a very short period of time.
Spike Testing Script with K6
The sample of code below will allow moderate requests of 100 to 500 requests and later spike to 1000 requests at 4 mins where it will continue at 1000 requests for a period of 8 mins before ramping down to 0 requests.
import http from 'k6/http';
export let options={
stages:[
{duration:'1m', target:100},
{duration:'4m', target:50},
{duration:'4m', target:1000},//spike the request to 1000 at 4 mins
{duration:'8m', target:1000},// stay at 1000 request for 8 mins
{duration:'2m', target:200},
{duration:'4m', target:0},
]
}
export default function () {
var url = "http://localhost:4000/api/v1/auth/login";
var payload = JSON.stringify({
email: 'admin@gmail.com',
password: '123456',
});
var params = {
headers: {
'Content-Type': 'application/json',
},
};
http.post(url, payload, params);
}
Stress Testing with K6
Stress testing is a type of load testing used to determine the limits of system. The purpose of this test is used to determine the stability and reliability of the system under extreme conditions.
Aim for Stress Testing
- Determin how the system works under extreme conditions.
- Determine what is your maximum capacity of your system in terms of users or thorughput.
- Determine the breaking point of your system and it's failure mode.
Spike Testing Script with K6
The sample of code demonstrating spike test will be sending normal request load for a while. It will then send 1000 request load which is the assumed breaking point for the webserver. Later at 2 mins the script will send 2000 requests load to go beyond the breaking point. It then finally scales down to 0 requests for the web server to recover.
import http from 'k6/http';
export let options={
stages:[
{duration:'2m', target:10},
{duration:'4m', target:50},//normal request load
{duration:'4m', target:1000},// request load around the breaking point
{duration:'4m', target:1000},
{duration:'2m', target:2000},// request load beyond the breaking point
{duration:'4m', target:0},//scale down to 0 requests for recovery stage
]
}
export default function () {
var url = "http://localhost:4000/api/v1/auth/login";
var payload = JSON.stringify({
email: 'admin@gmail.com',
password: '123456',
});
var params = {
headers: {
'Content-Type': 'application/json',
},
};
http.post(url, payload, params);
}
Circumstances to consider when classifying the performance of your web server
You can consider a web server to be Poor, Bad, Excellent, and Good with the following test reports.
Poor: System produces errors during the surge of traffic but recovers to normal after the traffic.
Bad: System crashes and does not recover after the traffic has subsided.
Execellent: System performance is not degraded during the surge of traffic.
Good:Response is slower but traffic doesn't produce any errors and all requests are handled.
With this article at OpenGenus, you must have the complete idea of Load Testing, Spike Testing, and Stress Testing Web Servers with K6.