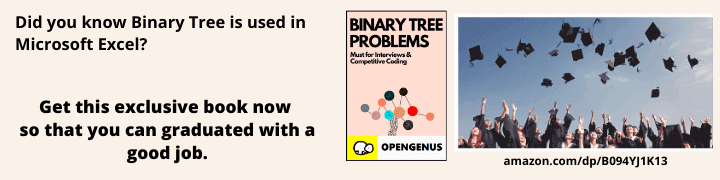
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
- Introduction
- Maps
- Sets
- How to Create and Use a Map of Sets
- Performance Analysis
- Useful Applications
- Questions
Reading time: 10 minutes
Introduction
In the C++ STL (Standard Library), the means to use a variety of containers are provided to arrange efficient methods of managing and manipulating data. Within this collection of supported containers, there are two we will focus on today:
- maps
- sets
Let us discuss how these two powerful containers can be combined and utilized to create a data structure known as a map of sets in C++ STL.
Maps
To utilize maps in C++, you must include the following at the beginning of your program:
#include <map>
A map in C++ is a container that stores key-value pairs, which contain a key and its corresponding value. In C++, key-value pairs look like:
key: value
The key acts as an identifier, therefore all keys in a map must be unique. They are sorted to allow for quick and efficient retrieval of data in an algorithm. Data values associated with keys can be of the following data types:
- int
- float
- long
- string
- bool
- user-defined types
- nested key-value pairs
Maps offer an extremely flexible container that can utilize an endless variety of data types.
Sets
To utilize sets in C++, you must include the following at the beginning of your program:
#include <set>
A set in C++ is a container that stores a collection of unique elements sorted in ascending order. Adding a duplicate entity to a set does not change the contents. As long as a data type in C++ has a defined comparison operator, either user-defined or provided by the language, it can be used in a set. This is because the program must have the ability to sort the elements. Without the comparison operator, it loses the ability to do so and would not be able to sort correctly.
Because of their unique sorting order, sets are perfect for algorithms that desire quick and efficient retrieval.
How to Create and Use a Map of Sets
To successfully use a map of sets in C++, you must first correctly define it. Here is the syntax:
#include <map>
#include <set>
std:: map<keyType, std:: set<valueType>> mapOfSets;
Depending on the data type, keyType and valueType should be replaced accordingly. You would also replace mapOfSets with the desired name of the map of sets you'd like to create.
Once you've correctly created a map of sets, it's time to insert the data into the map. Below is an example code that displays the method of inserting elements into the map:
#include <map>
#include <set>
int main() {
std::map<std::string, std::set<int>> mapOfSets;
mapOfSets["hello"].insert(10);
return 0;
}
Similarly, you can remove a key-value pair from the map of sets using the following:
mapOfSets.erase("hello");
To solely erase the value in the pair:
mapOfSets["hello"].erase(10);
Several operations can be performed on a map of sets such as finding an element or iterating over the collection. To find a specific value in the map of sets, you would use the following:
auto iterator = mapOfSets.find("hello");
The above code stores an iterator that points to the element in the map corresponding to the key "hello." One of its uses includes confirming whether or not a value exists.
Performance Analysis
Let's analyze the time complexity of several operations that can be used on a map of sets:
- insert(): O(logn)
- erase(): O(logn)
- find(): O(logn)
- iteration: O(n), where n is the number of key-value pairs in the map
Useful Applications
- Time Schedule: A map of sets can be a valuable tool when creating a schedule that consists of scheduled events with their corresponding date and time. These events will be organized correctly based on their scheduled time due to the properties of a map. In addition, an overlap of scheduling can be avoided with a quick check if a scheduled event already exists.
- Graph Algorithms: Each vertex and edge can be represented by the map and sets respectively, allowing for quick and easy management of graph data. Since each vertex is associated with a unique key, this grants the user swift access to the data point. Because each set in the map contains all of the neighboring vertices of a given vertex, this creates an adjacency list.