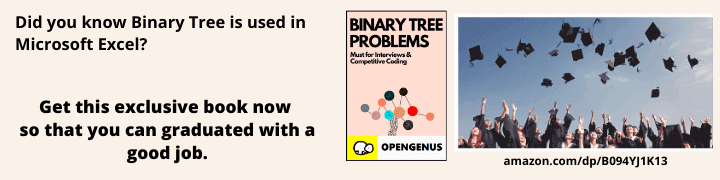
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the problem of setting an array of int to 1 using memset in C++ and the solution for it.
Table of contents:
- Problem with memset
- Solution: Memset array to 1 in C++
Problem with memset
The problem is that memset sets the given value to each byte while an array of int or other datatype have multiple bytes. Consider the case of array of integers (int). Size of int is 4 bytes.
So, if we memset an array of int to 1 using the following code line:
memset(array, 1, size);
1 will be set to each byte. So, an int in the array will be like:
00000001 00000001 00000001 00000001
This binary representation results in the int value of (2^0 + 2^8 + 2^16 + 2^24) = 16843009. Hence, the memset is initializing the array of ints to 16843009. This is an issue which we have solved in this OpenGenus article.
Solution: Memset array to 1 in C++
The solution to initialize an array of int to 1 using memset is as follows:
- Create an array of uint8_t datatype
- Initialize the array of uint8_t to 1 using memset
- Cast the uint8_t array to int array
uint8_t* array1 = (uint8_t *) malloc(size);
memset(array1, 1, size);
int* array2 = (int *) array1;
The array "array2" which is of datatype int will be initialize to 1 using memset.
Note: Performance-wise, this is not a recommended solution as it involves casting. Still, this will perform better than looping through an int array and setting each value to 1.
With this article at OpenGenus, you must have the complete idea of how to use memset to initialize an array of int to 1.