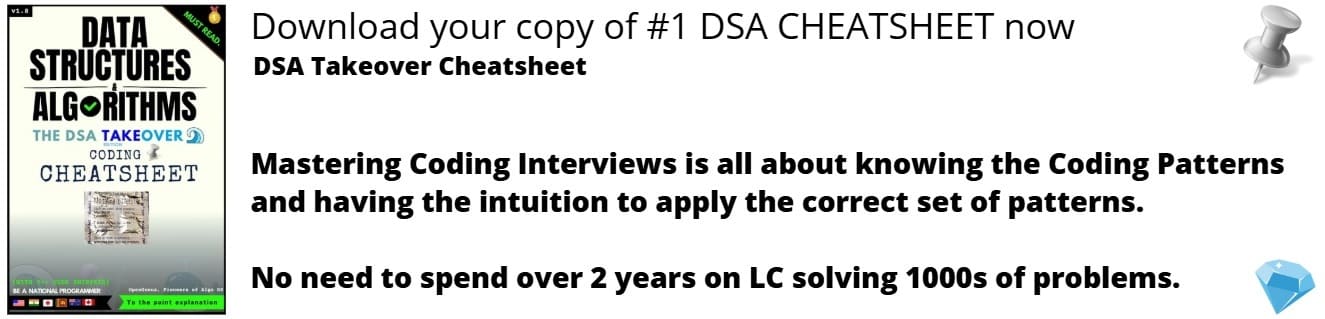
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the System Design of a scalable Payment Gateway such as PayPal and Stripe. We have presented functional, non-functional requirements, use case diagrams and much more.
Table of Contents
- What is a payment gateway
- Role of a payment gateway
- Functional and Non functional requirements
- Use case diagrams
- Implementation
- Examples of payment gateways
What is a payment gateway?
A payment gateway is a technology that allows merchants recieve online (card not present) credit or debit card payments from their customers. A payment gateway stands between the merchant and the customer.
Role of a payment gateway in online transactions
The payment gateway comes in handy in card-not-present transactions. The payment gateway performs card verification and authentication. It also securely passes the transaction information to the payment processor which then proceeds to send the transaction information to the issuig bank.
The issuing bank will either approve or decline the transaction and in either case, the response is sent back to the payment processor which if successfull will send the response to both the payment gateway and the acquiring bank.
Whereas if the transaction has been declined the payment processor will send the response back to the payment gateway which will forward the response to the merchant application. The payment processor stands between the merchant bank and the issuing bank.
Its quite clear a payment gateway is distinct from a payment processor but in certain Payment Service Providers (PSPs), you might find this as a single unit.
Functional and Non functional requirements
Functional requirements requirements describe the product capabilities or things that a product should do for its customers.
Non functional requirements describe the qualiy attributes, design and implementation constraints that a system must have.
Functional requirements
-
Accounts
1.1 The system will allow creation of merchant accounts.
1.2 The system will allow creation of customer accounts to capture card data
1.3 The system will allow users to log in with a username and password
1.4 The merchant shall be given a unique identifier to collect payments. -
Transactions
2.1 The system will allow customers to view previous payments.
2.2 The system will enable customers make payments
Non functional requirements
-
Perfomance
1.1 The system shall be scalable, reliable and available.
1.2 Payments should not be processed for more than 30 seconds. -
Security
2.1 The system shall be PCI DSS compliant. -
Operational
3.1 In cases of failed transactions, the system shall retry the transaction.
3.2 The system shall support around 1000 users.
3.3 The system shall be deployed as a SaaS.
Use Case
Use case Name: Make a payment
ID: PG-001
Actor: Customer
Description: This use case describes how a customer makes a payment to the merchant.
Trigger: The customer needs to pay for a merchant service or product.
Preconditions
- The transactions datastore is online.
- The customer is authenticated.
- The merchant application has integrated with the system.
Normal course
-
The system requests for the amount of money to be credited from the customer.
-
The system sends the transaction to the issuing bank.
-
If the payment request is authorized.
a. The system notifies the merchant acquiring bank.
b. The system notifies the customer and merchant application. -
If the transaction is declined.
a. The system notifies the merchant application. -
The system stores the issuing bank response in the transactions data store.
Post conditions
The transaction response is stored in the transactions datastore.
Technology stack
The technology stack defines the infrastructure our payment system will run on. The payment gateway system will be powered by python.
Due to the simplicity(Few features) of this system and the this being the initial development, we shall use the monolithic design.
Users of the system shall interact with the gateway via a REST API and as such, django-rest-framework shall be used for writing the views or routes and django-env shall be used for configuration.
MySQL shall be used for persistent storage and Apache shall be used to serve the application.
The payment process is an I/O bound process, and as such django-celery will be used to distribute the workload and preferably redis will be used as the message broker.
Implementation
Models
import abc
import datetime
class PaymentCard(abc.ABC):
card_no: int
class User(abc.ABC):
first_name: str
last_name: str
address: str
email: str
@abc.abstractmethod
def save():
raise NotImplementedError
class Visa(PaymentCard):
card_no: int
expiry_date: datetime.date
cvv: int
class MasterCard(PaymentCard):
pass
class AmericanExpress(PaymentCard):
pass
class AcquiringBank:
pass
class Customer(User):
card: PaymentCard
def save():
pass
class Merchant(User):
acquiring_bank: AcquiringBank
def save():
pass
class Payment(abc.ABC):
id: int
card: PaymentCard
amount: int
to: Merchant
Payment Gateway
from models import Payment, PaymentCard
class PaymentProcessor:
def authorize_payment(payment: Payment):
pass
class PaymentGateway:
def __init__(self, payment_processor: PaymentProcessor):
self.payment_processor = payment_processor
def validate_card(card: PaymentCard) -> bool:
# Check for expired cards, non existent cards
pass
def authorize_payment(self, payment: Payment):
self.validate_card(payment.card)
authorization_response = self.payment_processor.authorize_payment(payment)
return authorization_response
The system has two types of users ie Customers and Merchants. Customers are associated with a card that they use for payments and whereas the merchants are associated with an acquiring bank that will collect the merchant's payments.
The payment gateway class delegates the responsibility of processing payments to the payment processor, but before authorizing a payment, the payment gateway checks for the validity of the payment card.
6. Examples of payment gateways
The choice of the payment gateway for your business depends on your situation or needs.
- Paypal
Its simplicity and user friendliness has made it an excellent pick for users whose needs arent complex.
- Paypal is available in more than 200 countries.
- Paypal has a Purchase Protection that allows customers to request refunds incase of disatisfaction.
- Its quite easy to send money to your friends and family members at no costs.
- It fully protects the customer information through encryption and this lessens the workload for the merchants to meet the PCI DSS compliance obligation.
- Stripe
Stripe offers a host of features and this must be an excellent pick for developers. It offers customizable developer tools and allowing developers to create complex applications.
- Stripe supports a wide range of currencies though it is available in a few countries
- With all the customizable developer tools, it makes it a target for large companies however this makes it less user friendly for solo merchants.
With this article at OpenGenus, you must have the complete idea of Payment Gateway System Design.