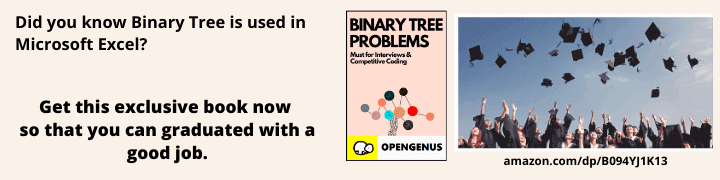
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to check if given number is even or odd and implemented the approach in C Programming Language.
Table of contents:
- Problem Statement
- Approach
- Implementation in C
- Output
- Optimized approach
Problem Statement
In this problem, we have to write a C program to take an integer as input and check if the given number is even or odd.
For example, if the input is 14, the program should give output that this is even number.
Similarly, if the input is 73, the program will give output that this is an odd number.
Approach
The approach to solve this problem is:
- Let the input integer is N.
- If the remainder of dividing N by 2 is 1, then N is odd.
- Else if the remainder of dividing N by 2 is 0, then N is even.
This is the most straight-forward approach.
Implementation in C
Following is the implementation of the above approach in C Programming Language:
#include<stdio.h>
int main()
{
int a,b;
printf("Enter a number=");
scanf("%d",&a);
b=a%2;
if(b==1)
{
printf("%d is odd",a);
}
else
{
printf("%d is even",a);
}
return 0;
}
Output
Following is the command to compile and run the above program:
gcc opengenus.c
./a.out
The output is as follows (enter a number like 35):
Enter a number=35
35 is odd
Optimized approach
When numbers are represented in binary format, the least significant bit is 1 if number is odd and it is 0 if it is even.
The implementation is as follows:
if(a & 1 == 0) // bitwise AND
printf("%d is even",a);
else
printf("%d is odd",a);
To understand bitwise operations, go through this article.
When we do a bitwise AND with 1, then only the least significant bit remains and all other bits are made 0. The least significant bit contributes 1 to the number and all other bits contribute powers of 2 starting from 2.
With this article at OpenGenus, you must have the complete idea of how to check if the given number is even or odd in C Programming Language.