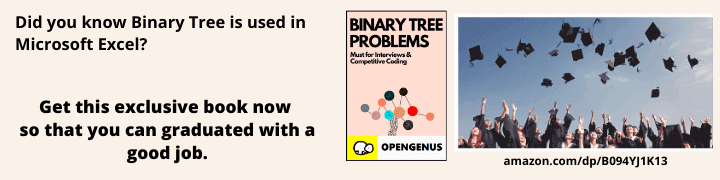
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to change time from 24 hours format (HH:MM) to 12 hours format (HH:MM + AM or PM) and implemented the approach in C Programming Language.
Table of contents:
- Problem Statement
- Approach to change 24 hours format to 12 hours format
- Implementation in C
- Output
Problem Statement
In this problem, we have to write a program in C programming language that takes a time in 24 hours format as input and gives the output time in 12 hours format.
For example, if the input time is 23:15 (HH:MM format), then output time will be 11:15 PM.
Similarly, if the input time is 10:49 (in 24 hours HH:MM format), the output time will be 10:49 AM.
Approach to change 24 hours format to 12 hours format
The approach/ steps to change the time format from 24 hours format to 12 hours format is as follows:
- The time is 24 hours format is of the form HH and MM where HH is hours from 00 to 24 and minutes MM is from 00 to 59.
- We assume the input time is a valid time.
- If HH is < 12, then the output is same as input with "am" appended to it.
- If HH is >= 12, then HH is modified as HH-12 and the new HH and original MM is the output with "pm" appended to it.
Implementation in C
Following is the implementation in C Programming Language:
#include<stdio.h>
int main()
{
int a,b,c;
printf("Enter a time in 24 hours format=");
scanf("%d%d",&a,&b);
if(a<12)
{
printf("%d:%dam",a,b);
}
else
{
c=a-12;
printf("%d:%dpm",c,b);
}
return 0;
}
Output
Following is the command to compile and run the program:
gcc opengenus.c
./a.out
Sample input is as follows:
21
34
The output of the above program is:
Enter a time in 24 hours format=9:34pm
Another sample input is as follows:
11
45
The output for the above input will be as follows:
Enter a time in 24 hours format=11:45am
With this article at OpenGenus, you must have the complete idea of how to change time from 24 hours format to 12 hours format and write a C program for it.