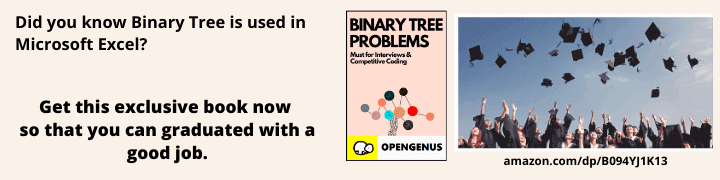
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will look at snprintf() function in C++ which is used to print strings in formatted way as a character buffer.
Table of contents:
- snprintf() function's use
- snprintf() prototype
- snprintf() parameters
- snprintf() return value
- Example on how snprintf() works
Use of snprintf() in C++
snprintf() function is basically used to write a formatted string to character string buffer.
Note: Unlike sprintf(), maximum number of characters that can be written to the buffer is specified in snprintf().
Prototype for snprintf()
The snprintf() function writes the string pointed by format to buffer. The maximum number of characters that can be written is (MAX_SIZE-1).
int snprintf( char* buffer, size_t MAX_SIZE, const char* format, ... );
After the characters are written, a terminating null character is added. If MAX_SIZE is equal to zero, nothing is written and buffer may be a null pointer.
It is defined in <cstdio>
header file.
snprintf() Parameters
-
buffer
Pointer to a buffer where the resulting string is stored. The buffer should have a size of at least MAX_SIZE characters. -
MAX_SIZE
Specifies the maximum number of bytes to be used in the buffer. The generated string has a length of at most MAX_SIZE-1, leaving space for the additional terminating null character. -
format
Pointer to a null terminated string that is written to the file stream. It consists of characters along with optional format specifiers starting with %. The format specifiers are replaced by the values of respective variables that follows the format string. -
... (additional arguments)
Depending on the format string, the function may expect a sequence of additional arguments, each containing a value to be used to replace a format specifier in the format string (or a pointer to a storage location, for MAX_SIZE).
There should be at least as many of these arguments as the number of values specified in the format specifiers. Additional arguments are ignored by the function.
Note: size_t is an unsigned integral type.
Format specifiers have following parts associated with them:
-
A leading % sign
-
Flags
: Modifies the conversion behavior. Following are types of flags:
'-' : Left justify the result within the field. By default it is right justified.
'+' : The sign of the result is attached to the beginning of the value, even for positive results.
'#' : An alternative form of the conversion is performed.
Space: If there is no sign, a space is attached to the beginning of the result.
'0' : It is used for integer and floating point number. Leading zeros are used to pad the numbers instead of space.
-
Width
: An optional * or integer value used to specify minimum width field. -
Length
: An optional length modifier that specifies the size of the argument. -
Precision
: An optional field consisting of a . followed by * or integer or nothing to specify the precision. -
Specifier
: A conversion format specifier. The available format specifiers are as follows:
Format specifier | Description |
---|---|
% | Prints % |
c | Writes a single character |
s | Writes a character string |
o | Converts an unsigned integer to octal representation |
d or i | Converts a signed integer to decimal representation |
X or x | Converts an unsigned integer to hexadecimal representation |
p | Writes an implementation defined character sequence defining a pointer. |
n | Returns the number of characters written so far by this call to the function. The result is written to the value pointed to by the argument |
A or a | Converts floating-point number to the hexadecimal exponent |
So the general format of format specifier is:
%[flags][width][.precision][length]specifier
- Other additional arguments specifying the data to be printed. They occur in a sequence according to the format specifier.
Return value of snprintf()
If successful, the snprintf() function returns number of characters that would have been written for sufficiently large buffer excluding the terminating null character. On failure it returns a negative value.
The output is considered to be written completely if and only if the returned value is nonnegative and less than MAX_SIZE.
Example
#include <cstdio>
#include <iostream>
using namespace std;
int main()
{
char buffer[100];
int returnValue, MAX_SIZE = 100;
char name[] = "Vedant";
int age = 19;
returnValue = snprintf(buffer, MAX_SIZE, "Hi, I am %s and I am %d years old. Nice to meet you.", name, age);
if (returnValue > 0 && returnValue < MAX_SIZE)
{
cout << buffer << endl;
cout << "Number of characters written = " << returnValue << endl;
}
else
cout << "Error writing to buffer" << endl;
return 0;
}
When you run the program, the output will be:
Output
Hi, I am Vedant and I am 19 years old. Nice to meet you.
Number of characters written = 56
Difference between snprintf() and sprintf()
sprintf() stores and converts the values if needed with the help of format parameter and the value is stored in bytes. This helps to identify the address easily in the function. A null character is placed in the end and hence it is necessary to make sure that enough space is allocated to accommodate the null character as well. Conversion types are also provided in the function to make it easy for users. The main difference between sprintf() and snprintf() is that in snprintf(), the buffer number to be specified in the function which is represented by ‘n’ in snprintf().
Note: sprintf( ) provides no bounds checking on the array pointed to by buf whereas in snprintf() maximum of num–1 characters will be stored into the array pointed to by buf. Also the array will be overrun if the output generated by sprintf( ) is greater than the array can hold which is not the case in snprintf().
At the time of concatenation, the functions are used differently in both sprintf and snprintf. For example, if we need to print ‘My name is Vedant’ in both sprintf and snprintf the method is different.
Sprintf example:
Sprintf (buf, “%s.%s”, buf, “My name “);
Sprintf (buf, “%s.%s”, buf, “is Vedant”);
Snprintf example:
Snprintf (buf, sizeof(buf), “%s”, “My name “);
Snprintf (buf+strlen(buf), sizeof(buf)-strlen(buf), “%s”, ” is Vedant”);
With this article at OpenGenus, you must have the complete idea of snprintf() function in C++.