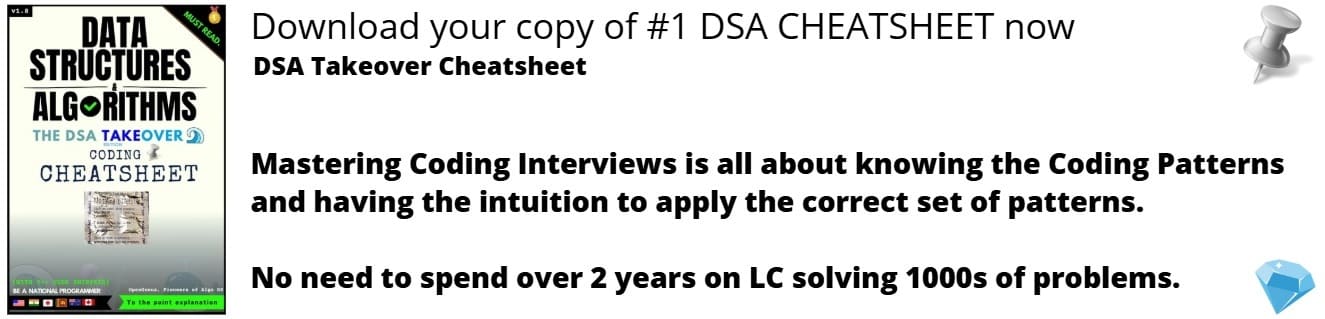
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
One of the most important concepts in object-oriented programming is inheritance. Inheritance allows us to define a class or a structure (struct) in terms of another class, which makes it easier to create and maintain an application. In doing so, the effect of reusing code functions and improving execution efficiency is also achieved.
Table of Content:
- Introduction
- Struct inheritance
- Multiple Inheritance in struct
- Structure Inheritance Vs Class Inheritance
- Conclusion
INTRODUCTION
What is inheritance?
Inheritance is an important mechanism in the C++ language. This mechanism automatically provides one class with operations and data structures from another class, which allows programmers to define components that are not in existing classes in new classes.
When creating a class, you don't need to rewrite new data members and member functions, you only need to specify that the new class inherits the members of an existing class. This existing class is called the base class , and the newly created class is called the derived class .
Inheritance represents there is a relationship. For example, a mammal is an animal, a dog is a mammal, therefore, a dog is an animal, and so on.
Can a struct be inherited in C++?
Yes, struct can also be inherited in C++. As simple as that.
So, what's the difference between inheriting a struct and a class?
Simply put, struct is exactly like class except the default accessibility for struct is public on the other hand the default accessibility for class is private.
Struct inheritance
Struct Inheritance can be simply defined as a struct inheriting from another struct.
It is almost similar to class inheritance the most essential difference being access control.
By default struct is public, class is private.
Basic Syntax :
struct A
{
int a;
};
struct B : A
{
int b;
};
Concept Explained :
The above is a very simple example of inheritance. At this time, B is a public inheritance of A. If you change the above struct to class, then B will become a private inheritance of A. This is the default inherited access. So when we usually write class inheritance, we usually write like this:
struct B : public A
It is to indicate that it is public inheritance instead of the default private inheritance.
This also means that struct can also inherit from class.
Implemented Code:
#include <iostream>
using namespace std;
struct baseStruct
{
void display()
{
cout<<"This is a base struct."<<endl;
}
};
struct subStruct:baseStruct
{
void show(){
cout<<"This is a sub struct."<<endl;
}
};
int main()
{
baseStruct s1;
s1.display();
subStruct s2;
s1.display();
s2.show();
return 0;
}
Output:
This is a base struct.
This is a base struct.
This is a sub struct.
Explanation:
In the above program we have created a base struct and a sub struct. the sub struct inherits from base struct.
So when in main function we create an object of sub struct it can use the functions of sub struct as well as base struct.
Note : Base struct cannot use the function of sub struct. Reason, it is only the sub struct that inherits.
Multiple Inheritance in struct
Consider the following case of multiple inheritance:
struct A
{
char a;
};
struct B
{
int b;
};
struct C : A, B
{
long c;
};
Explanation:
In the above skeleton program struct C inherits form both struct A and B.
Structure Inheritance Vs Class Inheritance
Structure Inheritance | Class Inheritance | |
---|---|---|
The default data access control of struct is public. | The default data access control of class is private | |
Everything in struct is publicly inherited, for single / multilevel / hierarchical inheritance, but not hybrid and multiple inheritance. | For class it is private for all types of inheritances. | |
struct is not used to define template parameters. | class is also used to define template parameters. | |
struct is less secure. | class is more secured than struct. |
The following two programs perfectly shows difference between Structure Inheritance and Class Inheritance.
Program 1: Using Class Inheritance
#include <iostream>
using namespace std;
class parent {
public:
int x=10;
};
class child : parent {
void display(){
cout<<x;
}
};
int main()
{
child c;
c.display();
return 0;
}
Output 1:
error: 'int parent::x' is inaccessible within this context
Program 2: Using Struct Inheritance
#include <iostream>
using namespace std;
struct parent {
public:
int x=10;
};
struct child : parent {
void display(){
cout<<x;
}
};
int main()
{
child c;
c.display();
return 0;
}
Output 2:
10
Explanation:
We understand the first program gives us an error because the child class object is unable to access the display function because the basic access specifier of a class is private.
On the other hand the child struct object is able to inherit the display function of the parent class because the basic access control is set private.
Conclusion
In this article at OpenGenus, we learnt about struct inheritance in C++. Its basic implementation and how it is different from class inheritance.