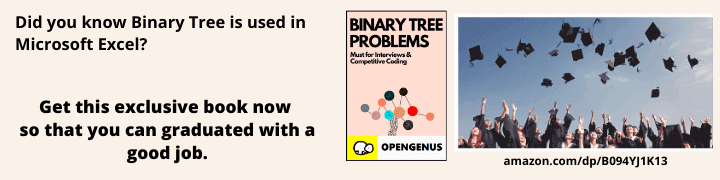
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about substr() function in C++ which is used to extract a sub-string from a given string.
What is a String?
A string is a sequence of characters which is used to alter or store text. In C++, string is a datatype which is used to store continuous characters and it ends when they encounter a space or a newline.
The C++ STL(Standard Template Library) provides a string class called 'std::string' that represents a string.
In C++, strings are zero-indexed, so the first character in the string has an index of 0.
Here is an example of how to use the 'std::string' class to declare and initialize a string.
#include <iostream>
#include <string>
using namespace std;
int main()
{
//Declare and Initialize a string
string str = "Welcome to the world of Programming";
//print the string
cout << "String: " << str << endl;
return 0;
}
Output of the above program:
String: Welcome to the world of Programming
C++ Substring substr()
A substring is a part of string and we use 'substr()' function for string slicing or exracting a substring from a string. The substr() function is defined in the string header file. The substr() function takes two arguments: the starting position of the substring and the number of characters which we want to extract from the given string i.e. length of substring. This substr() function does not change the original string and returns a newly created string object containing the substring. Following is the syntax of substr() function.
substr(position, length)
- position is the starting index of substring in the given string
- length is the number of characters in the substring
Note:
- If the position is equal to the length of the string, the function returns an empty string.
- If the length is not specified, the substring till the end is returned.
Example:
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "Welcome to the world of Programming";
//Extract the substring "world" from the string
string substrg = str.substr(15,5);
cout<<" Original string: "<<str<<endl;
cout<<" Extracted substring: "<<substrg<<endl;
return 0;
}
In the above example the 'substr' function is called on the string object 'str' with the arguments '15' and '5' which tells the 'substr' function to extract the substring starting at index '15' and it should have a length of '5' characters.
The output of this program will be:
Original string:: Welcome to the world of Programming
Extracted substring: world
Note: If we try to use the substr() function to extract a substring from the position which is outside the range of the original string, we will get an out of range error. This error indicates that we are trying to access a part of the string that does not exist and it will crash our program.
It is important to include the try and catch blocks to handle the out_of_range exception, otherwise the program will crash if the starting position or the length are out of range.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "Welcome to the world of Programming";
try{
//This will throw an out_of_range exception
//because the starting position is out of range
string substrg = str.substr(90,5);
cout << "Extracted substring: " << substrg << endl;
}
catch(const out_of_range& e)
{
cerr << "Error: " << e.what() << endl;
}
return 0;
}
Output
Error: basic_string::substr: __pos (which is 90) > this->size() (which is 35)
With this article at OpenGenus, you must have the complete idea of substr in C++.