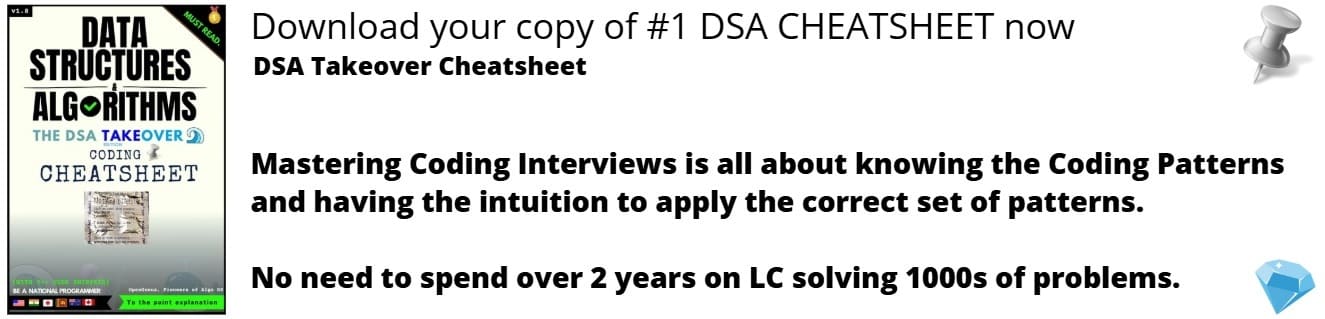
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the System Design for Parking Lot in depth presenting the system requirements, technology stack, estimation of requirements, UML Class diagrams and much more.
Introduction to System Design
System design is the process of designing the elements of a system such as the architecture, modules and components, the different interfaces (UI/UX) of those components so as to meet the end-user requirements and expectations. Major tasks performed during the system design process are initialising design definition, establishing design characteristics, assessing all possible alternatives and managing the design.
Steps in System Design
The above 6 steps are the major steps that is followed for almost any system design.
Now, let us see each step briefly.
Requirement Specification:
For any project, narrowing down to a specific goal and targets is very important so that things do not get complicated later on. Requirements are basically of two types:
- Functional
- Non-Functional requirements
Estimation of Requirements:
Now that the system requirements are planned, the next step is to estimate the quantity or the amount of resources needed. This can include estimating the amount of storage needed, computing resources required, etc.
Data Flow:
This step involves identifying the type of data being handled and the type of database to use(Relational DB, NoSQL DB,etc.).
High level Component Design:
In this step, the components of the system are planned. In this step, the main components of the system and their relations are sketched.
Detailed Design:
Now that the major or the core components are decided, each component is analysed in detail. In this stage, all the different approaches to solve a problem are considered and analysis is done to find out the best approach.
The detailed design may also include:
- User interfaces
- Data and control interaction between units
- Algorithms and data structures, etc.
Identify and Resolve Bottlenecks:
The final step of system design is to identfy bottlenecks(A bottleneck is any point of congestion in a project that causes delays in the workflow. Bottlenecks in project management reduce the pace of the project due to limited capacity.) in the system and resolve them. Bottlenecks can include data, storage, availability, network traffic, etc.
Understanding the problem (Parking lot system)
The parking lot system is a set of essential tools and processes that makes the parking lot function much faster and be more efficient. The components of the parking lot system may differ depending on the parking lot, but generally, the system includes core functions like giving the customer a ticket on entering and generate the bill or price on exit.In this case, we are considering two users: the customer and the admin(or, the owner of the parking lot). The customer can check availability of parking slot and can get a ticket after parking the vehicle. The admin has to set the parking rates and also generate a bill once the parked vehicle is taken.
System Requirements
The requirements for a parking lot system provide a complete description of the system behavior and are based on the business expectations. The key requirements that need to be offered by the parking lot system can be classified into functional and non-functional requirements.
Functional Requirements:
Functional Requirements describe the service that the system must offer. In the case of Parking lot system, there are two access levels: Admin Mode, and Customer Mode:
- Customer:
- Register at the parking lot
- Check parking slot availability
- Get the ticket
- Update parking slot reservation
- Update parking status
- Admin:
- Set parking rates
- Provide billing data
- Manage the system and parking data
- Analyse the parking data
- Display bill amount
- Generate a receipt
The following use case diagram provides an overview of the basic services:
The following class diagram also provides an overview of how the classes will be structured and the relations between the classes:
Non-Functional Requirements:
For a parking lot system, the most important non-functional requirements include security, performance and availability.
Security:
The data obtained from the customers must be encrypted and stored securely. The system also must employ firewall software as a defense against network attacks to avoid data leakage. As the database contains the details of the customer's vehicles, Unauthorized access to the data should not be allowed.
Performance:
The system must effectively utilize the hardware resources to maximise efficiency. The system is expected to perform efficiently so that the customers do not wait long at the parking lot.
Availability:
The system is expected to be available during general working hours of the office and schools (usually 6:00 AM to 10:00 PM). It is even more effeective if it is 24x7 as there are some customers who may need to park their vehicle in the night hours.
Estimation of Requirements
One of the important points of the system design is to know about the scale of the system. If the measurements of the system are very large in number, then they are high scale systems. Parking lot system is a high scale system as many cars come regularly and the data is updated regularly.
As there is nothing to compute except for the check-in time, more investment need not be done on the computing resources. Even for the storage, it is not necessary to store the details of the vehicle once the vehicle is checked out. Thus, the amount of storage required depends directly on the parking lot capacity as at any point of time, the database contains only the details of the vehicles that are in the parking lot at that time. Assuming the parking lot is very large and has a vehicle capacity of a crore vehicles(10000000), the maximum storage needed will 1.5 GB as an average of 150 Bytes of data is needed to store the details of a vehicle.
Data Flow
As all the required details are mandatory, Relational DataBases can be used and the data can be structed in rows and columns. SQL-based database like Microsoft SQL Server or Oracle RDBMS can be used for the parking lot system. The entities for each insert query are: Vehicle number, Parking-lot slot and the Check-in Time.
High Level Component Design
After analysing and deciding the requirements and the data flow, the next step is to describe the system architecture on a higher level.
Monolithic Architecture
The traditional way to implement parking lot system is with a monolithic architecture, where all features of a software reside in a single repository or code base. If any code updates are required, then the developer has to use the same code base, make the required changes and then re-deploy the updated code. So even if a single change is required, the whole code base is updated and re-deployed.
The monolithic architecture is the easiest to implement, but it is hard to maintain in the long run, and it is very hard to add new features or update the old ones. But, as there will be lesser software updates for a parking lot system and the basic functionalities remain the same, we can go for monolithic architecture.
Detailed Design
Now that the architecture is also decided, the next step is to analyse various methods of implementations and choose the best among those. In short, the tech stack and the algorithms for problem solving are decided in this stage.
The application components for a parking lot can be coded in any suitable object oriented programming language like Python or Java. Object oriented programming languages are preferred for this system as concepts like classes, objects, Inheritance, etc. are applied in this design.
Tech Stack:
- For any application, we should design both the front-end and the back-end for it to be complete and fully functional.
- For the front-end (User Interface) of the application, we can either make use of the GUI packages like turtle(python) or develop a portal(website) using Web frameworks like React, Angular, Vue, etc.
- For the backend(logics and functionalities), we can either use Python or Java.
- Java can be used in the case of larger parking slots as Python is less scalable in terms of performance and execution speed for larger scales.
- Python can be used for smaller parking lots as it is also an Object oriented programming language and it is developer-friendly(easier to code).
- As we make use of servers, web-servers like Apache, Microsoft Internet Information Services (IIS) or Nginx can be used.
- The maximum load at any given point in the system will be dependent on the capacity of the parking lot and the number of incoming vehicles for parking (check-in) or check-out, Load balancers and CDNs (Content Delivery Networks) are not needed.
A sample implementation of the various classes for a small parking slot using Python is given below.
Python Sample code:
# class for vehicle size
class v_size:
bike = 1
car = 2
class Vehicle:
def __init__(self):
self.occupied_spots = []
self.capacity = 10000 # any number of spots
def get_capacity(self):
return self.capacity # return number of spots
def park_in_spot(self, spot):
self.occupied_spots.append(spot) # add spot to occupied spots
def clear_spots(self):
for spot in self.occupied_spots:
spot.remove_vehicle() # remove vehicle from spot
class bike(Vehicle):
def __init__(self):
Vehicle.__init__(self)
self.size = v_size.bike # set size to bike
def can_fit_in_spot(self, spot):
return True
class car(Vehicle):
def __init__(self):
Vehicle.__init__(self)
self.size = v_size.car # set size to car
def can_fit_in_spot(self, spot):
return spot.get_size() == v_size.car # return true if spot is car size
class ParkingSpot:
def __init__(self, n, sz):
self.spot_number = n
self.spot_size = sz
self.vehicle = None
def is_available(self):
return self.vehicle == None # return true if spot is empty
def can_fit_vehicle(self, vehicle):
return self.is_available() and vehicle.can_fit_in_spot(self) # return true if spot is empty and vehicle can fit in spot
def park(self, v):
if not self.can_fit_vehicle(v):
return False # return false if vehicle can't fit in spot
self.vehicle = v
self.vehicle.park_in_spot(self) # park vehicle in spot
return True
def remove_vehicle(self):
self.level.spot_freed() # remove vehicle from spot
self.vehicle = None # set vehicle to none
def get_spot_number(self):
return self.spot_number # return spot number
def get_size(self):
return self.spot_size # return spot size
class ParkingLot:
def __init__(self, per_row):
self.spots_per_row = 1000
self.number_spots = 0
self.available_spots = 0
self.spots = []
def checkIn(self, vehicle):
if self.get_available_spots() < vehicle.get_spots_needed():
return False
# No space to park the vehicle
spot_num = self.find_available_spots(vehicle) # find available spot
if spot_num < 0:
return False # no available spots
return self.park_starting_at_spot(spot_num, vehicle) # park vehicle in spot
def find_available_spots(self, vehicle):
spots_found = 0
for i in range(len(self.spots)):
spot = self.spots[i]
if spot.can_fit_vehicle(vehicle):
spots_found += 1
else:
spots_found = 0
return -1
def park_starting_at_spot(self, spot_num, vehicle):
vehicle.clear_spots()
s = True
for i in range(spot_num, spot_num + vehicle.get_spots_needed()):
s = s and self.spots[i].park(vehicle) # park vehicle in spot
self.available_spots -= vehicle.get_spots_needed()
return s
def spot_freed(self):
self.available_spots += 1
def get_available_spots(self):
return self.available_spots
Identify and Resolve Bottlenecks
This is the last step in the system design process and this is where we identify the possible challenges on implementing this design.
One major bottleneck is that this system has a Single Point Failure if there is only one admin system. If the singular admin system faces an issue and fails to update the check-in or check-out of a vehicle, then it can lead to unwanted chaos. This can be fixed by having multiple admin systems so that if one fails, there is an alternative.
With this article at OpenGenus, you must have got a strong idea about the System Design of the Parking Lot System.