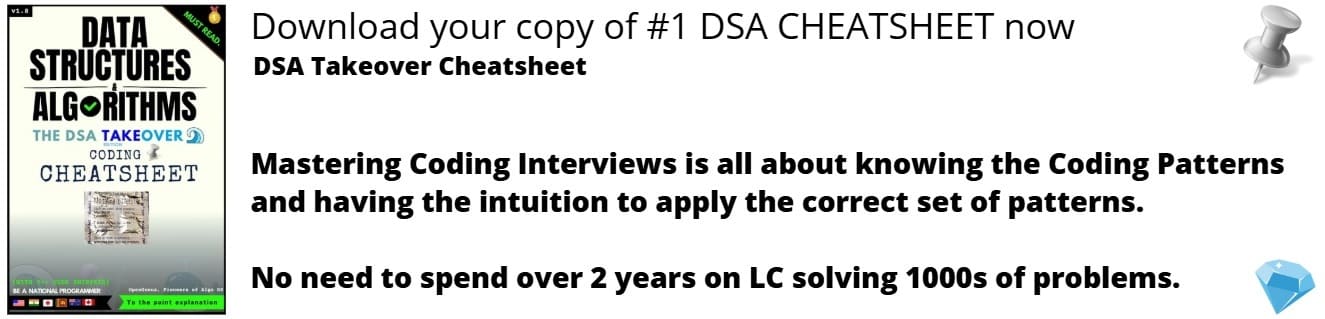
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will understand how to develop a Timer Console Application which simulates a countdown timer with a Progress Bar and a countdown timer display.
Introduction
- A Timer is a useful tool for measuring time intervals, counting down to events or deadlines, and managing time effectively.
- In the digital age, timers are ubiquitous and can be found in various forms, including wristwatches, smartphones, and desktop applications.
- In this article, we will focus on a console-based timer application that can be easily implemented in Java.
- This application displays a progress bar and a countdown timer in the console, providing a visual representation of the remaining time for a given timer value.
- The progress bar displays the elapsed time with green blocks and the remaining time with red blocks, while the countdown timer displays the remaining time in minutes and seconds, as well as the percentage of the elapsed time.
- This application is a useful tool for timing events, tasks, and activities, and can be easily customized to suit specific needs.
- In this article, we will explore the code of a sample Java program that implements a console-based timer application and explain its key components and functionalities in detail.
Approach
The TimerApp program has a simple design and consists of three main methods: main, getValidTimerValue, and startTimer.
- The main method is responsible for prompting the user for a valid timer value and starting the timer thread using the startTimer method.
- The getValidTimerValue method ensures that the user enters a valid timer value and returns it to the main method.
- The startTimer method updates the progress bar and countdown timer display in the console.
- The progress bar is constructed using green and red blocks to represent the elapsed and remaining time, respectively.
- The countdown timer display is formatted to show the remaining time in minutes and seconds, as well as the percentage of the elapsed time.
- The startTimer method runs in a separate thread, allowing the main thread to continue executing and accepting user input.
- The TimerAppv2 program uses Java's Thread class and sleep method to implement the timer functionality.
- The program also uses Java's Scanner class to read user input from the console and handle input validation errors.
- The program demonstrates the use of Java's string formatting and StringBuilder classes to construct the progress bar and countdown timer display.
- The program's design and implementation can be easily customized and extended to include additional features, such as audio alerts or customizable progress bar colors.
Implementation
The TimerApp program is a console-based timer application written in Java. Its implementation can be broken down into three main parts: user input validation, timer thread creation, and console output display.
1.User Input Validation
private static int getValidTimerValue() {
int timer = 0;
while (true) {
System.out.print("Enter timer value in seconds: ");
try {
timer = sc.nextInt();
if (timer > 0) {
break;
} else {
System.out.println("Timer value must be greater than 0.");
}
} catch (Exception e) {
System.out.println("Invalid input. Please enter a valid timer value.");
sc.nextLine();
}
}
sc.close();
return timer;
}
- The getValidTimerValue method is responsible for validating user input and returning a valid timer value.
- This method uses a while loop to repeatedly prompt the user to enter a timer value until a valid input is provided.
- The nextInt method of the Scanner class is used to read the user's input as an integer value, and an exception is caught if the input is not an integer.
- If the input is less than or equal to zero, an error message is displayed, and the loop continues. If the input is valid, the method returns the input value.
2.Timer Thread Creation
private static Thread startTimer(int timer) {
Thread thread = new Thread(() -> {
long startTime = System.currentTimeMillis();
long elapsedTime = 0;
while (elapsedTime < timer * 1000) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
break;
}
elapsedTime = System.currentTimeMillis() - startTime;
float progress = (float) elapsedTime / (timer * 1000);
String progressBar = getProgressBar(progress);
String countdownTimer = getCountdownTimer(timer, elapsedTime);
System.out.print("\r" + progressBar + " " + countdownTimer);
}
});
thread.start();
return thread;
}
- The startTimer method takes an integer timer value as input and returns a new thread object that is started to run.
- Inside the thread's run method, the current time is stored in startTime, and the time elapsed is stored in elapsedTime.
- The loop continues until the elapsed time is less than the timer value multiplied by 1000 (to convert to milliseconds).
- Inside the loop, the thread is paused for 100 milliseconds using Thread.sleep(). After the pause, the elapsed time is recalculated by subtracting the start time from the current time.
- A progress value is calculated as a percentage of elapsed time to the total timer value.
3. Console Output Display
private static String getProgressBar(float progress) {
StringBuilder sb = new StringBuilder();
sb.append("[");
int completed = (int) ((1 - progress) * PROGRESS_BAR_WIDTH);
int remaining = PROGRESS_BAR_WIDTH - completed;
for (int i = 0; i < completed; i++) {
sb.append("\033[32mâ–ˆ\033[0m");
}
for (int i = 0; i < remaining; i++) {
sb.append("\033[31mâ–ˆ\033[0m");
}
sb.append("]");
return sb.toString();
}
private static String getCountdownTimer(int timer, long elapsedTime) {
long remainingTime = timer * 1000 - elapsedTime;
float remainingPercentage = (float) remainingTime / (timer * 1000) * 100;
remainingPercentage = Math.round(remainingPercentage * 100) / 100f;
remainingTime = remainingTime / 1000 + 1;
return String.format("%02d:%02d (%.1f%%)", remainingTime / 60, remainingTime % 60, remainingPercentage);
}
- The progress bar is updated using the getProgressBar method, which constructs a string of green and red blocks to represent the elapsed and remaining time, respectively.
- The countdown timer display is updated using the getCountdownTimer method, which formats the remaining time as minutes and seconds and calculates the percentage of the elapsed time.
- The timer thread updates the progress bar and countdown timer display in the console using the System.out.print method.
- The progress bar and countdown timer are displayed on a single line using the \r escape sequence, which moves the cursor to the beginning of the current line.
- The progress bar and countdown timer are concatenated using the + operator, and the resulting string is printed to the console.
Code Highlights
Here are some key code snippets from the TimerAppv2 program:
int timer = getValidTimerValue();
- reads the timer value from the user
Thread timerThread = startTimer(timer);
- creates and starts the timer thread
while (elapsedTime < timer * 1000) { ... }
- implements the timer loop
System.out.print("\r" + progressBar + " " + countdownTimer);
- updates the console output
Code
import java.util.Scanner;
public class TimerApp {
private static final int PROGRESS_BAR_WIDTH = 50;
// Create Scanner object for user input
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) throws InterruptedException {
// Get timer value from user input
int timer = getValidTimerValue();
// Start timer
System.out.print("\nStarting timer... ");
Thread timerThread = startTimer(timer);
timerThread.join();
// Display timer finished message
System.out.println("\nTimer finished!");
}
// Get a valid timer value from user input
private static int getValidTimerValue() {
int timer = 0;
while (true) {
System.out.print("Enter timer value in seconds: ");
try {
timer = sc.nextInt();
if (timer > 0) {
break;
} else {
System.out.println("Timer value must be greater than 0.");
}
} catch (Exception e) {
System.out.println("Invalid input. Please enter a valid timer value.");
sc.nextLine();
}
}
sc.close();
return timer;
}
// Start the timer in a new thread
private static Thread startTimer(int timer) {
Thread thread = new Thread(() -> {
long startTime = System.currentTimeMillis();
long elapsedTime = 0;
while (elapsedTime < timer * 1000) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
break;
}
// Calculate progress and display progress bar and countdown timer
elapsedTime = System.currentTimeMillis() - startTime;
float progress = (float) elapsedTime / (timer * 1000);
String progressBar = getProgressBar(progress);
String countdownTimer = getCountdownTimer(timer, elapsedTime);
System.out.print("\r" + progressBar + " " + countdownTimer);
}
});
// Start the thread and return it
thread.start();
return thread;
}
// Get progress bar string based on current progress
private static String getProgressBar(float progress) {
StringBuilder sb = new StringBuilder();
sb.append("[");
int completed = (int) ((1 - progress) * PROGRESS_BAR_WIDTH);
int remaining = PROGRESS_BAR_WIDTH - completed;
for (int i = 0; i < completed; i++) {
sb.append("\033[32mâ–ˆ\033[0m"); // green block
}
for (int i = 0; i < remaining; i++) {
sb.append("\033[31mâ–ˆ\033[0m"); // red block
}
sb.append("]");
return sb.toString();
}
// Get countdown timer string based on remaining time
private static String getCountdownTimer(int timer, long elapsedTime) {
long remainingTime = timer * 1000 - elapsedTime;
float remainingPercentage = (float) remainingTime / (timer * 1000) * 100;
remainingPercentage = Math.round(remainingPercentage * 100) / 100f;
remainingTime = remainingTime / 1000 + 1;
return String.format("%02d:%02d (%.1f%%)", remainingTime / 60, remainingTime % 60, remainingPercentage);
}
}
- The above code is implementation of Timer Console Application in JAVA.
- Look at the example output
main() method
- The main method is the entry point of the application.
- It calls the getValidTimerValue method to get a valid timer value from the user.
- The ""Starting timer... " message is displayed to indicate that the timer has started.
- The startTimer method is called with the timer value to start the timer.
- The timerThread.join() method is used to wait for the timer thread to finish before proceeding.
- Finally, the "Timer finished!" message is displayed to indicate that the timer has completed.
Conclusion
- In conclusion of this article at OpenGenus, we have developed a timer console application that allows users to set a timer and see the progress of the timer using a progress bar and countdown timer.
- We have used Java and multi-threading to achieve this functionality.
- The application also includes error handling for invalid user input.
- This project can be further improved by adding additional features such as audio alerts upon timer completion or the ability to run multiple timers simultaneously.
- Overall, this timer console application provides a simple and convenient way for users to keep track of their time.