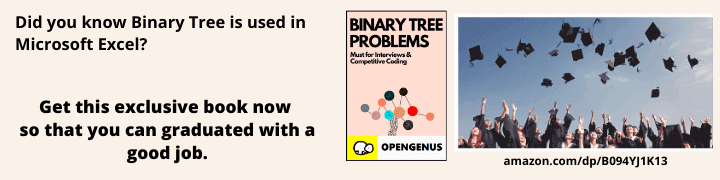
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the reason behind the error "Undefined reference to pthread_create" and presented two fixes in the compilation command to fix this compilation error.
Table of contents:
- Reason for the error
- Fix 1: pthread
- Fix 2: lpthread
In short, the fix is to use pthread flag in compilation:
gcc -pthread -o code code.c
Reason for the error
While compiling a C code using the function pthread_create(), you may face this compilation error:
code.c: In function βmainβ:
/tmp/cc8BMzwx.o: In function `main':
term.c:(.text+0x82): undefined reference to `pthread_create'
collect2: ld returned 1 exit status
This issue can come when you are not linking the pthread library during compilation.
Following is a sample C code where pthread_create is used and where the compilation error will be faced:
#include <pthread.h>
#include <stdio.h>
#define NUM_THREADS 64
void *run(void * id)
{
// ... Do computation
pthread_exit(NULL);
}
int main (int argc, char *argv[])
{
pthread_t threads[NUM_THREADS];
for(iter=0; iter < NUM_THREADS; ++iter){
pthread_create(&threads[t], NULL, run, (void *)iter);
}
pthread_exit(NULL);
}
Error if you compile using the command:
gcc -o code code.c
We will explore 2 fixes.
Fix 1: pthread
pthread option is used to add multi-threading support in C code with pthread library. It links both preprocessor and linker.
gcc -pthread -o code code.c
Fix 2: lpthread
Another fix is to add lpthread option to the compilation command. It adds pthread library in the linker. Depending on your system, this may fix the error while -pthread option is a complete solution.
gcc -o code code.c -lpthread
With these fixes in this article at OpenGenus, you must have the complete idea of how to fix this compilation error "Undefined reference to pthread_create".