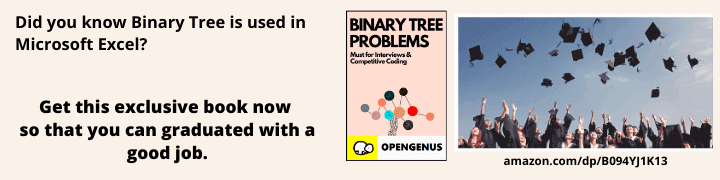
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 15 minutes
Array is a container in C++ STL which are used to store homogeneous (same) type of data and provides several useful functionalities over it. Arrays in STL provides the static implementation of arrays that is the size of array does not increase once created. To understand Dynamic Array, see this. In order to use array, the array library must be included.
#include <array>
Syntax of array :
array<object_type, array_size> array_name;
Example:
array<int, 10> opengenus;
Initialization of Array:
#include <array>
int main()
{
//It will create an empty integer array of size 3
array<int, 3> numbers;
//It will create an integer array of size 3 having elements 1,2 & 3
array<int, 3> num = {1, 2, 3};
return 0;
}
Different functions of array
Following are the major functions supported by array container:
- Accessing Array
a) at() function
b) front() function
c) back() function
d) data() function
e) operator [] - Modifying Array
a) swap() function
b) beign() and end() function
c) cbeign() and cend() function
d) crbeign() and crend() function
e) rbeign() and rend() function - Miscellaneous
a) size() function
b) max_size() function
c) fill() function
d) empty() function
Functions Supported By Array
1. at() function
at() function in array container returns the value stored at given position or index.
#include <iostream>
#include <array>
using namespace std;
int main(){
//It will create an integer array of size 3 having elements 1,2 & 3
array<int, 3> num = {1, 2, 3};
cout<<num.at(0); // Prints 1
cout<<num.at(2); // Prints 3
return 0;
}
2. front() function
front() function in array returns the value stored at first position in array. It is used to fetch the first element from array.
#include <iostream>
#include <array>
using namespace std;
int main(){
//It will create an integer array of size 3 having elements 1,2 & 3
array<int, 3> num = {1, 2, 3};
cout<<num.front(); // Prints 1
return 0;
}
3. back() function
back() function in array container returns the value stored at last position in array. It is used to fetch the last element from array.
#include <iostream>
#include <array>
using namespace std;
int main(){
//It will create an integer array of size 3 having elements 1,2 & 3
array<int, 3> num = {1, 2, 3};
cout<<num.back(); // Prints 3
return 0;
}
4. fill() function
fill() function in array fill the array with a particular value.
#include <iostream>
#include <array>
using namespace std;
int main()
{
//It will create an empty integer array of size 3
array<int, 3> num;
num.fill(10); //fill value 10 in array at every position
for(int i=0;i<3;i++)
cout<<num.at(i)<<" ";
return 0;
}
Output
10 10 10
5. empty() function
empty() function of array container returns true if array is empty otherwise returns false.
#include <array>
#include <iostream>
using namespace std;
int main(){
array<int, 0> num;
if(num.empty())
cout<<"Array is Empty";
else
cout<<"Array is Not Empty";
return 0;
}
Output
Array is Empty
6. max_size() function
max_size() function of array container returns the maximum number of elements the container is able to hold.
#include <array>
#include <iostream>
using namespace std;
int main(){
array<int, 10> num;
cout<<"Array can store upto "<<num.max_size()<<" elements";
return 0;
}
Output
Array can store upto 10 elements
7. begin() and end() function
array::begin() returns the iterator to the begining or iterator to the first element of the array
array::end() returns the iterator to the ending of array or iterator to the last element of array
#include<iostream>
using namespace std;
int main(){
array<int,5> numberes{10,20,30,40,50};
for(auto it = numbers.begin();it!=numbers.end();it++)
cout<< *it <<" ";
return 0;
}
output
10 20 30 40 50
8. cbegin() and cend() function
array::cbegin() returns the const_iterator to the begining or the first element of array . it points to the constant content . it can not be used to modify the elements it points to
array::cend() returns the const_iterator to the ending or the last element of array
#include <iostream>
#include <array>
using namespace std;
int main ()
{
array<int,5> myarray = { 2, 16, 77, 34, 50 };
for ( auto it = myarray.cbegin(); it != myarray.cend(); ++it )
cout << ' ' << *it; // cannot modify *it
return 0;
}
output
2 16 77 34 50
8. crbegin() and crend() function
array::crbegin() returns the const_reverse_iterator to the begining or the first element of array . A const_reverse_iterator is an iterator that points to const content and iterates in reverse order.
array::crend() returns the const_reverse_iterator to the ending or the last element of array .
#include <iostream>
#include <array>
using namespace std;
int main ()
{
array<int,6> myarray = {10, 20, 30, 40, 50, 60} ;
for(auto rit=myarray.crbegin() ; rit < myarray.crend(); ++rit )
cout << ' ' << *rit; // cannot modify *rit
return 0;
}
output
60 50 40 30 20 10
9. rbegin() and rend() function
array::rbegin() returns a reverse iterator pointing to the last element in the array container.
array::rend() returns a reverse iterator pointing to the theoretical element preceding the first element in the array
#include <iostream>
#include <array>
using namespace std;
int main()
{
array<int, 5> arr = { 1, 5, 2, 4, 7 };
// prints all the elements
cout << "The array elements in reverse order are:\n";
for (auto it = arr.rbegin(); it != arr.rend(); it++)
cout << *it << " ";
return 0;
}
output
The array elements in reverse order are:
7 4 2 5 1
10. data() function
array::data() returns the pointer to the first element of the array
#include<iostream>
#include<array>
using namespace std;
int main(){
array<int , 5> arr{1,2,3,4,5};
cout<<"First Element of array = "<<*(arr.data());
return 0;
}
output
First Element of array = 1
11. operator[]
array::operator[] This operator is used to reference the element present at position given inside the operator. It is similar to the at() function
#include<iostream>
#include<array>
using namespace std;
int main(){
array<int,5> arr{1,2,3,4,5};
cout<<"Second Element = "<<arr[1]<<endl;
cout<<"Forth Element = "<<arr[3];
return 0;
}
output
Second Element = 2
Forth Element = 4
12. size() function
array::size() it returns the size of array or length of array
#include<iostream>
#include<array>
using namespace std;
int main(){
array<int,5> arr{1,2,3,4,5};
cout<<"Size of Array = "<<arr.size();
return 0;
}
output
Size of Array = 5
13. swap() function
array::swap() This function is used to swap the contents of one array with another array of same type and size.
#include <array>
#include <iostream>
using namespace std;
int main()
{
array<int, 4> myarray1{ 1, 2, 3, 4 };
array<int, 4> myarray2{ 3, 5, 7, 9 };
// using swap() function to swap elements of arrays
myarray1.swap(myarray2);
// printing the first array
cout<<"myarray1 = ";
for(auto it=myarray1.begin(); it<myarray1.end(); ++it)
cout<<*it<<" ";
// printing the second array
cout<<endl<<"myarray2 = ";
for(auto it=myarray2.begin(); it<myarray2.end(); ++it)
cout<<*it<<" ";
return 0;
}
output
myarray1 = 3 5 7 9
myarray2 = 1 2 3 4
With this article at OpenGenus, you have a strong idea of the different functionalities supported by Array container in C++ STL. You should definitely try using this in your code and observe how it eases implementation. Enjoy.