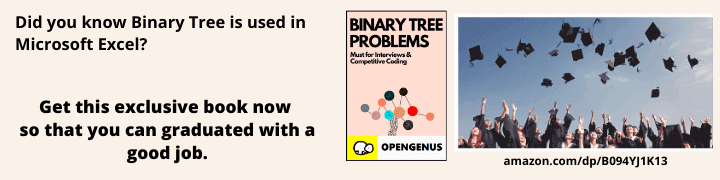
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
C programming language (from C99) supports Boolean data type (bool) and internally, it was referred as _Bool
as boolean was not a datatype in early versions of C. In C, boolean is known as bool data type. To use boolean, a header file stdbool.h must be included to use bool in C.
bool is an alias to _Bool
to avoid breaking existing C code which might be using bool as an identifier. You can learn about _Bool
here in detail.
#include <stdbool.h>
Note if we do not include the above header file, then we need to replace bool with _Bool
and the code will work as usually.
Standard logical operators AND (&&), OR(||) and NOT(!) can be used with the Boolean type in any combination.
In computer science, the Boolean data type is a data type that has one of two possible values, either TRUE or FALSE. Due to two possible values, it needs only 1 bit. In actual computing systems, the minimum amount of memory is set to a particular value (usually 8 bits) which is used (all bits as 0 or 1).
Memory
An object declared as type Bool is large enough to store the values 0 and 1.
printf("%zu", sizeof(bool));
The above code will give size 1 for bool, so generally bool store a 1 byte of memory. Note: it needs only 1 bit but takes 8 bits due to the structure of the computing system.
For example:
- true is denoted as 00000001
- false is denoted as 00000000
Declaration
To declare a variable as a boolean use:
bool variable_name = true;
Example:
#include <stdbool.h>
#include <stdio.h>
int main()
{
bool a = true;
if(a)
printf("Its ture");
return 0;
}
Output:
Its true
Bool with Logical Operators
We can use logical operators with boolean.
Types of logical operators:
- && (AND): takes 2 booleans; returns true only if both operands are true else false
- || (OR): returns true if either or both of the operands are true else false
- ! (NOT): takes 1 operand; return true if operand is false and false if operand is true
Example:
#include <stdio.h>
#include <stdbool.h>
int main(void)
{
bool a=true, b=false;
printf("%d\n", a&&b);
printf("%d\n", a||b);
printf("%d\n", !b);
}
Output:
0
1
1
Bool Array
#include <stdbool.h>
int main()
{
bool arr[2] = {true, false};
printf("Value at index 1 of array is %d",arr[1]);
return 0;
}
Output:
Value at index 1 of array is 0
How to convert a boolean to integer? (type casting)
A type cast is basically a conversion from one type to another.
An object declared as type Bool is large enough to store the values 0 and 1.
Example:
#include <stdio.h>
#include <stdbool.h>
int main() {
int n = 1;
bool x = true;
n = (bool)true;;
printf("%d",n); //Output: 1
return 0;
}
There's no need to cast to bool for built-in types because that conversion is implicit. On converting to other integral types, a true bool will become 1 and a false bool will become 0.
Question
Consider the following C code:
#include <stdbool.h>
#include <stdio.h>
int main()
{
bool a = true;
bool b = false;
if(a == 1)
printf("Its ture");
else if(b == 0)
printf("B is fasle");
else
printf("Did not work!");
return 0;
}