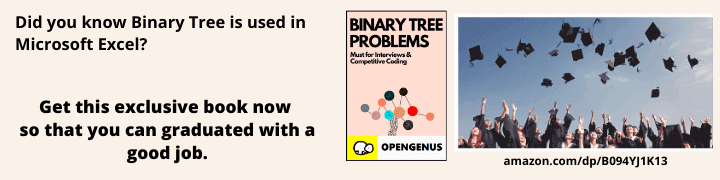
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
A constructor is a special member function which enables the object of a class to initialize itself when it is created. This is also known as automatic initialization of objects.
We have explored different types of constructors, constructor overloading and constructor with default arguments.
But the next question that arises is, why is it a special member function?
It is so because of the following characteristics :
- Its name is same as the class name
- Constructors do not return any values, hence do not have any return types (not even void)
- They are invoked automatically when the object is created
- Constructors cannot be virtual unlike destructors.
To understand constructors, we will take the example of a class called Student with the following attributes:
char name[50];
int age;
Types of Constructors in C++
The types of constructors are:
- Default constructor
- Parametrized Constructors
- Copy Constructors
- Default Constructor: It is a constructor that accepts no parameters. If no such constructor is defined, then the compiler supplies one as shown below:
Student s; // This statement invokes the default constructor of the compiler
We can explicitly define one too:
Student(){
cout << "Default constructor called!" << endl;
age = 20;
}
// We can invoke the constructor as:
Student s1;
- Parametrized Constructors: These type of constructors accept arguments and are useful when it is necessary to initialize data elements at the time of object creation.
Student (char n[50], int a) {
cout << "Parameterized constructor called!" << endl;
age = a;
strcpy(name, n);
}
//We can invoke the constructor as:
char x[50] = "Angelina";
Student s2(x, 20);
s2.print_details();
However, when a constructor has been parameterized, the object declaration like one below may not work unless a default constructor has been explicitly defined!
Student s1; // This may not work
- Copy Constructors: It is used to declare and initialize an object from another object. This process is known as copy initialization. A copy constructor takes a reference to an object of the same class as itself as an argument.
Student(Student & s) {
cout << "Copy constructor called!" << endl;
strcpy(name, s.name);
age = s.age;
}
//We can invoke the constructor as:
Student s3(s2);
// Another way to invoke it is,
Student s3 = s2;
Now one may ask, is it possible to have more than one type of constructor in a class to support different ways of object initialization. Fortunately, the answer is YES as discussed below!
Constructor Overloading
The process of sharing the same name by two or more functions is referred to as function overloading. Similarly, we say that the constructor is overloaded when more than one constructor function is defined in a class.
We can define all the types of constructors explained above in the class Student to demonstrate constructor overloading.
class Student {
char name[50];
int age;
public:
Student(){ age = 20;}
Student (char n[50], int a) {
age = a;
strcpy(name, n);
}
Student(Student & s) {
strcpy(name, s.name);
age = s.age;
}
};
// We can invoke the different constructors as below,
Student s1; // invokes default constructor
char x[50] = "Angelina";
Student s2(x, 20); // invokes parameterized constructor
Student s3 = s2;
s3.print_details(); // invokes copy constructor
Now, let's understand how to use constructors with default arguments!
Constructor with Default Arguments
Like other functions in C++, constructors support default arguments. However, the actual parameters, when specified, override the default value. Also, the default arguments must be the trailing ones. An example is shown below,
Student (char n[50], int a = 21) {
age = a;
strcpy(name, n);
}
char y[50] = "Charles";
Student s4(y); // assigns the default value of 21 to age attribute
Student s5(y, 25); // assigns the specified value of 25 to age attribute
This brings us to the end of the article at OpenGenus in which we have learnt about the major aspects of Constructors in C++!