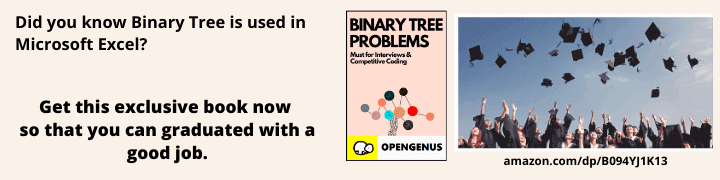
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
In Java, a constructor is a block of code similar to a method which is used to initialize objects. It is called when an instance of a class is created. A constructor is invoked every time an object is created using the new() operator. If a constructor is not defined, then the compiler provides a default constructor. The default constructor performs no particular task and takes up no arguments.
The key features of constructors are:
- A constructor does not have a return type
- The name of the constructor is the same as the name of the class
- A constructor is automatically called on object creation
- A class can have more than one constructor as long as the argument list is different. Thus, overloading of constructors is possible.
- A constructor can be public, private, protected and default
Types of Java Constructors
Constructors can be divided into two major categories:
- Default Constructor (no-args Constructor)
- Parameterized Constructor
Default Constructor in Java
These constructors do not accept any arguments. They are used to initialize the object and provide them with either values such as 0, null or to provide some predefined values. The following example of default constructor initializes the object f1 and sets length and breadth to 0.
public class Fields {
double length;
double breadth;
public Fields()
{
System.out.println("A new object under fields was created");
}
void display()
{
System.out.println("Length = "+length+" Breadth = "+breadth);
}
public static void main(String[] args){
Fields f1 = new Fields();
f1.display();
}
}
Output:
A new object under fields was created
Length = 0 Breadth = 0
Suppose when an object is created it is required for the object to have specific values for length and breadth i.e. predefined values. It can be done by simply specifying the values for the data members under the default constructor.
public class Fields {
double length;
double breadth;
public Fields()
{
length = 100;
breadth = 50;
}
void display()
{
System.out.println("Length = "+length+" Breadth = "+breadth);
}
public static void main(String[] args){
Fields f1 = new Fields();
f1.display();
}
}
Output:
Length = 100 Breadth = 50
Parameterized Constructor in Java
A parameterized constructor always accepts arguments when an object of the class is created. It is used to provide different values, given in by the users or otherwise, to distinct objects.
public class Fields {
double length;
double breadth;
public Fields(double len, double br)
{
length = len;
breadth = br;
}
void display()
{
System.out.println("Length = "+length+" Breadth = "+breadth);
}
public static void main(String[] args){
Fields f1 = new Fields(300, 200);
f1.display();
}
}
Output:
Length = 300 Breadth = 200
Constructor Overloading in Java
Constructor Overloading can be done in Java wherein more than one constructor, with different parameter list, can reside in a class. Each constructor performs a different task. They are differentiated by the compiler by the number of parameters in the argument list and their types.The following example demonstrates constructor overloading:
public class Fields {
double length;
double breadth;
public Fields()
{
}
public Fields(double len, double br)
{
length = len;
breadth = br;
}
public Fields(double dim)
{
length = breadth = dim;
}
void display()
{
System.out.println("Length = "+length+" Breadth = "+breadth);
}
public static void main(String[] args){
Fields f1 = new Fields();
Fields f2 = new Fields(300, 200);
Fields f3 = new Fields(500);
f1.display();
f2.display();
f3.display();
}
}
Output:
Length = 0.0 Breadth = 0.0
Length = 300.0 Breadth = 200.0
Length = 500.0 Breadth = 500.0
What are the Difference between Constructor and Method in Java?
- Constructor is used to initialize an object. Method performs operations on objects or are used to tell about the behaviour of objects.
- Constructor must have the same name as the Class. Method must have a different name from the class.
- A constructor is invoked implicitly. A method is invoked explicitly.
- A constructor does not have a return type. A method has a return type.
Copy Constructor in Java
Unlike C++, Java does not have a default(built-in) copy constructor. However, values of one object can be copied to another by writing your own copy constructor.
There are three ways to copy values of one object into another:
- By constructor
- By assigning the values of one object into another
- By clone() method of Object class
The following example illustrates the use of copy constructors:
public class Fields {
double length;
double breadth;
public Fields(double len, double br)
{
length = len;
breadth = br;
}
Fields(Fields f)
{
length = f.length;
breadth = f.breadth;
}
void display()
{
System.out.println("Length = "+length+" Breadth = "+breadth);
}
public static void main(String[] args){
Fields f1 = new Fields(300, 200);
Fields f2 = new Fields(f1);
System.out.println("Object f1:");
f1.display();
System.out.println("Object f2:");
f2.display();
}
}
Output:
Object f1:
Length = 300.0 Breadth = 200.0
Object f2:
Length = 300.0 Breadth = 200.0
Questions on Constructors in Java
1. How does the object creation take place when there exists a class with no constructor in it ?
When the compiler encounters a class with no constructor in it, it automatically creates a default constructor.
2. What is the use of a private constructor in Java ?
A private constructor ensures that no outside class can create an object of this class. That is, objects can only be created internally within the class.
3. How is a no-arg constructor different than a default constructor ?
In a no-arg constructor, the values of data members can be set to values other than the default values.
If the class has no constructor, then a default constructor with no formal parameters and no throws clause is implicitly declared by the compiler.