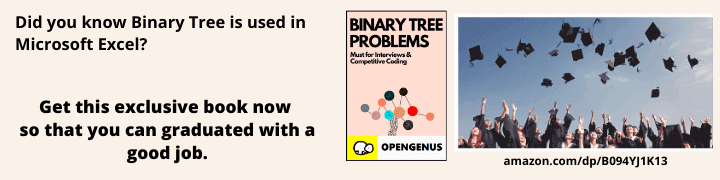
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
The ctype.h header file of the C Programming Language provide several functions that are used to testing different characters and changing some properties. Some common tasks are to check if a character is upper case, lower case, hexadecimal digit and more.
If one has a knowledge of mapping of characters to the numeric value, then developing such functions is easy.
We can include the ctype.h header file as:
#include <ctype.h>
Library Functions of ctype.h
The following are the functions defined in the header ctype.h:
- isalnum: is character alphanumeric?
- isalpha: is character alphabetic?
- iscntrl: is it a control character (like newline)?
- isdigit: is it a digit?
- isgraph: does it have a graphical representation?
- islower: is it a lower case character?
- ispunct: is it a punctuation?
- isspace: is it a space?
- isupper: is it a upper case character?
- isxdigit: is it a hexadecimal?
- tolower: convert to lower case character
- toupper: convert to upper case character
We will go through each function in detail now.
isalnum()
- isalnum() - This function checks whether the passed character is alphanumeric.
#include <stdio.h>
#include <ctype.h>
int main(void) {
char a;
printf("Enter a character: ");
scanf("%c", &a);
if (isalnum(a) == 0)
printf("%c is not an alphanumeric character.", a);
else
printf("%c is an alphanumeric character.", a);
return 0;
}
Output:
Enter a character: /
/ is not an alphanumeric character.
isalpha()
- isalpha() - This function checks whether the passed character is alphabetic.
#include <stdio.h>
#include <ctype.h>
int main(void) {
char a;
printf("Enter a character: ");
scanf("%c", &a);
if (isalpha(a) == 0)
printf("%c is not an alphabet.", a);
else
printf("%c is an alphabet.", a);
return 0;
}
Output:
Enter a character: a
a is an alphabet.
iscntrl()
- iscntrl() - This function checks whether the passed character is control character.
#include <stdio.h>
#include <ctype.h>
int main()
{
char a;
int outcome;
a = 'J';
outcome = iscntrl(a);
printf("When %c is passed to iscntrl() = %d\n", a, outcome);
a = '\n';
outcome = iscntrl(a);
printf("When %c is passed to iscntrl() = %d", a, outcome);
return 0;
}
Output:
When J is passed to iscntrl() = 0
When
is passed to iscntrl() = 2
isdigit()
- isdigit() - This function checks whether the passed character is decimal digit.
#include <stdio.h>
#include <ctype.h>
int main()
{
char a;
printf("Enter a character: ");
scanf("%c",&a);
if (isdigit(a) == 0)
printf("%c is not a digit.",a);
else
printf("%c is a digit.",a);
return 0;
}
Output:
Enter a character: a
a is not a digit.
isgraph()
- isgraph() - This function checks whether the passed character has graphical representation using locale.
#include <stdio.h>
#include <ctype.h>
int main()
{
int one = '7';
int two = ' ';
int three = 'r';
if( isgraph(one) ) {
printf("%c can be printed\n", one );
} else {
printf("%c can't be printed\n", one );
}
if( isgraph(two) ) {
printf("%c can be printed\n", two );
} else {
printf("%c can't be printed\n", two );
}
if( isgraph(three) ) {
printf("%c can be printed\n", three );
} else {
printf("%c can't be printed\n", three );
}
return(0);
}
Output:
7 can be printed
can't be printed
r can be printed
islower()
- islower() - This function checks whether the passed character is lowercase letter.
#include <stdio.h>
#include <ctype.h>
int main () {
int one = '9';
int two = 'z';
int three = 'B';
if( islower(one) ) {
printf("%c is lowercase character\n", one );
} else {
printf("%c is not lowercase character\n", one );
}
if( islower(two) ) {
printf("%c is lowercase character\n", two );
} else {
printf("%c is not lowercase character\n", two );
}
if( islower(three) ) {
printf("%c is lowercase character\n", three );
} else {
printf("%c is not lowercase character\n", three );
}
return(0);
}
Output:
9 is not lowercase character
z is lowercase character
B is not lowercase character
ispunct()
- ispunct() - This function checks whether the passed character is a punctuation character.
#include <stdio.h>
#include <ctype.h>
int main () {
int one = '.';
int two = 'w';
if( ispunct(one) ) {
printf("%c is a punctuation character\n", one );
} else {
printf("%c is not a punctuation character\n", one );
}
if( ispunct(two) ) {
printf("%c is a punctuation character\n", two );
} else {
printf("%c is not a punctuation character\n", two );
}
return 0;
}
Output:
. is a punctuation character
w is not a punctuation character
isspace()
- isspace() - This function checks whether the passed character is white-space.
#include <stdio.h>
#include <ctype.h>
int main () {
int one = ' ';
if( isspace(one) ) {
printf("%c is a white-space character\n", one );
} else {
printf("%c is not a white-space character\n", one );
}
return(0);
}
Output:
is a white-space character
isupper()
- isupper() - This function checks whether the passed character is an uppercase letter.
#include <stdio.h>
#include <ctype.h>
int main () {
int one = 'f';
if( isupper(one) ) {
printf("%c is an uppercase character\n", one );
} else {
printf("%c is not an uppercase character\n", one );
}
return(0);
}
Output:
f is not an uppercase character
isxdigit()
- isxdigit() - This function checks whether the passed character is a hexadecimal digit.
#include <stdio.h>
#include <ctype.h>
int main () {
char one[] = "2xP";
if( isxdigit(one[0]) ) {
printf("%s is a hexadecimal character\n", one );
} else {
printf("%s is not a hexadecimal character\n", one );
}
return(0);
}
Output:
2xP is a hexadecimal character
tolower()
- tolower() - This function converts uppercase letters to lowercase.
#include <stdio.h>
#include <ctype.h>
int main () {
int x = 0;
char a;
char str[] = "Hello World";
while( str[x] ) {
putchar(tolower(str[x]));
x++;
}
return(0);
}
Output:
hello world
toupper()
- toupper() - This function converts lowercase letters to uppercase.
#include <stdio.h>
#include <ctype.h>
int main () {
int x = 0;
char a;
char str[] = "Hello World";
while( str[x] ) {
putchar(toupper(str[x]));
x++;
}
return(0);
}
Output:
HELLO WORLD
With this, you have the complete knowledge to use ctype.h in C.