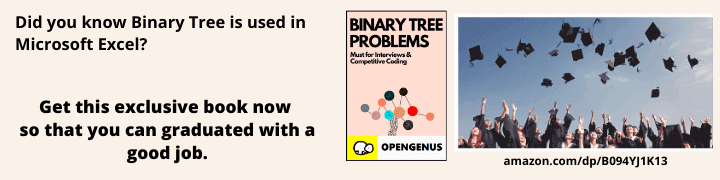
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 2 minutes
Map in C++ STL is a container that maps unique key values of some data type to values of a different or similar type such that the keys always remain sorted, The ordering relation Compare of keys can be customized by constructor parameters at the time of map initialization.
Some Properties
- Internally implemented as Red-Black trees which have an insertion Time Complexity of O(log n) and re-balancing rotation Time Complexity of O(1).
- Is a node-based dynamic container hence memory Allocation is not contiguous.
In this article, our focus will be to provide information about various methods of map initialization.
For a more descriptive overview of C++ STL maps in general, please click meVector Initialization Ways in C++
We'll demonstrate various Initialization methods by taking an example case where our need is to create the following map, mapping integer keys to integer values.
Key | Value |
---|---|
1 | 10 |
2 | 20 |
3 | 30 |
4 | 40 |
Generic Syntax
#include <map> //Header That implements map
template < class Key, // map::key_type
class T, // map::mapped_type
class Compare = less<Key>, // map::key_compare
class Alloc = allocator<pair<const Key,T> > // map::allocator_type
> class map;
Method 1 (Default Constructor)
Default constructor doesn't take any params and creates an empty map with no key-value pairs at the time of initialization.
#include <map>
int main()
{
// (1) Using Default constructor
std::map<int, int> mdefault;
mdefault[1] = 10;
mdefault[2] = 20;
mdefault[3] = 30;
mdefault[4] = 40;
return 0;
}
We've used '[ ]' operator to insert values into our map.
Method 2 (Range Constructor)
Range constructor is used to copy a range of values from an existing map.
Range is passed to the map constructor in format [start, end) which copies values starting from start till the element end but exclusive of end
#include <map>
int main()
{
std::map<int, int> m;
m[1] = 10;
m[2] = 20;
m[3] = 30;
m[4] = 40;
m[5] = 50;
m[6] = 60;
// (2) Using Range constructor
std::map<int, int> mrange(m.find(2), m.find(5));
return 0;
}
In the above code, we are creating our desired map mrange by copying a range of values from an existing map m.
Method 3 (Copy Constructor)
Copy constructor is used to copy a whole existing map and the map to be copied is passed as a single param to the constructor.
#include <map>
int main()
{
std::map<int, int> m;
m[1] = 10;
m[2] = 20;
m[3] = 30;
m[4] = 40;
// (3) Using Copy constructor
std::map<int, int> mcopy(m);
return 0;
}
In the above code, we are creating our desired map mcopy by copying the whole existing map m.
Method 4 (Move Constructor)
Move constructor is used to move a whole existing map, the map being moved is left in a valid state but no longer contains its elements. A single param is passed to the constructor which is move function taking the map to be moved as a param.
#include <map>
int main()
{
std::map<int, int> m;
m[1] = 10;
m[2] = 20;
m[3] = 30;
m[4] = 40;
// (4) Using Move constructor
std::map<int, int> mmove(std::move(m));
return 0;
}
In the above code, we are creating our desired map mmove by moving all elements from the existing map m, after the constructor has successfully completed creation of new map mmove the previous map m is left with no key-value pairs.
Method 5 (Initializer list Constructor)
An Initializer List is a sequence of values separated by commas and wrapped in Curly braces, they are used to initialize data members of classes which is a map in our case as can be seen below.
#include <map>
int main()
{
// (5) Using Initializer list constructor
std::map<int, int> minitlist {
{1, 10},
{2, 20},
{3, 30},
{4, 40},
};
return 0;
}
In the above code, we are creating our desired map minitlist by representing our desired map value in Initializer list form and eventually using Initializer list Constructor.