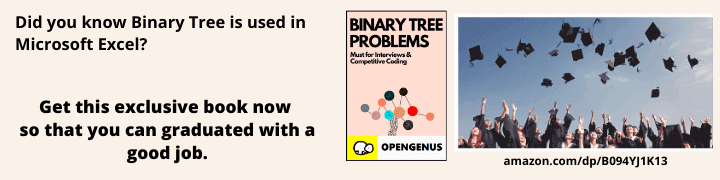
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Welcome to this article on math.h header file that we can use in C language to perform various mathematical operations like square root, trigonometric functions and a lot more.
I know it can be a tedious task to go through a bunch of articles in order to find what you're searching for in particular, that's why in this article I'll try to get you familiar with this header file upto an extent where you will be able to understand its usecase and also the functions included in it.
I have divided this article in three parts:
- In the first part I'll introduce you to various concepts like header files, how to include them in your program and what is math.h.
- In the second part we'll learn in more depth about various functionalities that are included in the math.h.
- There will be a small quiz of 4 questions to test your knowledge that you will again after reading this article
So, Let's begin 😃
First, let's understand what is a header file?
-
A header file is a file with extension .h which basically contains function declarations and macro definitions that we can share between several source files. Basically it is a general practice in C to keep all the constants, macros, system wide global variables, and all the standard library functions in the header files and include that header file wherever it is required.
-
Now you guys might have a question Why do we need header files? Why can't we just add the function required for the program right then and there. Your question is valid but here comes the characteristic of a GOOD PROGRAM i.e. it must be easy to read and understand and thus we write those function definitions in the header files that are often used in order to reduce the complexity of the program.
-
All of the header files are stored in standard library of the C programming language.
-
You can use a header file in your program by including it with the C preprocessing directive #include, like you have seen inclusion of stdio.h header file, which comes along with your compiler.
For example:-
#include<stdio.h> //This particular file is composed of several standard #defines(macros) to define some of the standard I/O operations.
#include<math.h>
#include<stdlib.h>
Explanation:
- #include is the directive that works by directing the C preprocessor to scan the specified file(i.e. the header file) as input before continuing with the rest of the program.
- When we do that all Ccode of the header files(including function definitions and macro definitions) are included in our program (in the above given case it'll include all the C code that is present in stdio.h, math.h, stdlib.h). Then, the current program is compiled by compiler and executed.
Now let's understand what is math.h header file in particular?
- math.h is a header file in the standard library of the C programming language designed for basic mathematical operations and transformations.
- Various mathematical operations that can be performed using this library are stored in the library in the form of functions that we can use once we we've included it in our program.
- Most of the functions involve the use of floating point values. Which implies that the functions available in this library take floating point values as arguments and also they'll return the result in floating point values.
Congratulations!! Now you're well versed with the concept of header files, how to include them in you program and what is math.h basically.
Now let's move on to the other part of the article where we will discuss various functions that are included in math.h header file.
Now let's learn about the various functions that are included in the math.h header file
NOTE: This article will not discuss all the functions that are present in the math.h header file. Rather it'll discuss all the functions that are often required and used on a regular basis. Don't worry you'll get everything that you need to know at this point of time to begin with this header file 😉 .
1. double acos(double x)
Description: This function is used to return the arc cosine of x in radians.
Declaration syntax: double acos(double x)
Parameters: x is a floating point value in the interval [-1, 1].
Illustration through example:
// Program to illustrate double acos(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
#define PI 3.14159265 //defined a macro to represent the value of Pi.
int main () {
double x, ret, val;
x = 0.7;
val = 180.0 / PI; // Will use this value to convert radians to degree.
printf("The arc cosine of %f is %f radians \n", x, acos(x)); // Here we used the function acos(x) and returned the value in radians.
ret = acos(x) * val; // Converting radians to degrees.
printf("The arc cosine of %f is %f degrees \n", x, ret); //In degrees.
return(0);
}
Output of the code above:
The arc cosine of 0.700000 is 0.795399 radians
The arc cosine of 0.700000 is 45.572996 degrees
[Finished in 0.8s]
2. double asin(double x)
Description: This function is used to return the arc sine of x in radians.
Declaration syntax: double asin(double x)
Parameters: x is a floating point value in the interval [-1, 1].
Illustration through example:
// Program to illustrate double asin(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
#define PI 3.14159265 //defined a macro to represent the value of Pi.
int main () {
double x, ret, val;
x = 0.7;
val = 180.0 / PI; // Will use this value to convert radians to degree.
printf("The arc sine of %f is %f radians \n", x, asin(x)); // Here we used the function asin(x) and returned the value in radians.
ret = asin(x) * val; // Converting radians to degrees.
printf("The arc sine of %f is %f degrees \n", x, ret); //In degrees.
return(0);
}
Output of the code above:
The arc sine of 0.700000 is 0.775397 radians
The arc sine of 0.700000 is 44.427004 degrees
[Finished in 0.3s]
3. double cos(double x)
Description: This function is used to return the cosine of a radian angle x.
Declaration syntax: double cos(double x)
Parameters: x is a floating point value representing an angle in radians.
Illustration through example:
// Program to illustrate double cos(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
#define PI 3.14159265 //defined a macro to represent the value of Pi.
int main () {
double x, ret, val;
x = 60.0;
val = PI / 180.0; // Will use this value to convert radians to degree.
printf("The cosine of %lf is %lf radians \n", x, cos(x)); // Here we used the function cos(x) where x was an angle in radians.
ret = cos(x * val); // Converting radians to degrees first and then computing the sine usings cos(x) where x was in degrees.
printf("The cosine of %lf is %lf degrees \n", x, ret); //In degrees.
return(0);
}
Output of the code above:
The cosine of 60.000000 is -0.952413 radians
The cosine of 60.000000 is 0.500000 degrees
[Finished in 1.9s]
4. double sin(double x)
Description: This function is used to return the sine of a radian angle x.
Declaration syntax: double sin(double x)
Parameters: x is a floating point value representing an angle in radians.
Illustration through example:
// Program to illustrate double sin(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
#define PI 3.14159265 //defined a macro to represent the value of Pi.
int main () {
double x, ret, val;
x = 90.0;
val = PI / 180.0; // Will use this value to convert radians to degree.
printf("The sine of %lf is %lf radians \n", x, sin(x)); // Here we used the function sin(x) where x was an angle in radians.
ret = sin(x * val); // Converting radians to degrees first and then computing the sine usings sin(x) where x was in degrees.
printf("The sine of %lf is %lf degrees \n", x, ret); //In degrees.
return(0);
}
Output of the code above:
The sine of 90.000000 is 0.893997 radians
The sine of 90.000000 is 1.000000 degrees
[Finished in 1.1s]
5. double ceil(double x)
Description: This function is used to return the smallest integer value greater than or equal to x.
Declaration syntax: double ceil(double x)
Parameters: x is any floating point value.
Illustration through example:
// Program to illustrate double ceil(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
float val1, val2, val3, val4;
val1 = -1.5;
val2 = 8.7;
val3 = 3.0;
printf ("value1 = %.1lf\n", ceil(val1));
printf ("value2 = %.1lf\n", ceil(val2));
printf ("value3 = %.1lf\n", ceil(val3));
return 0;
}
Output of the code above:
value1 = -1.0
value2 = 9.0
value3 = 3.0
[Finished in 0.8s]
6. double floor(double x)
Description: This function is used to return the largest integer value less than or equal to x.
Declaration syntax: double floor(double x)
Parameters: x is any floating point value value.
Illustration through example:
// Program to illustrate double floor(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
float val1, val2, val3, val4;
val1 = -1.5;
val2 = 8.7;
val3 = 3.0;
printf ("value1 = %.1lf\n", floor(val1));
printf ("value2 = %.1lf\n", floor(val2));
printf ("value3 = %.1lf\n", floor(val3));
return 0;
}
Output of the code above:
value1 = -2.0
value2 = 8.0
value3 = 3.0
[Finished in 1.5s]
Hey, Stop right there ✋
Did you notice something 🤔
In the output of 6 and 7 the values of val3 was same.
Do you know why???
I know you do BUT even if you don't, need not to worry. I'll explain it to you in a matter of seconds 😃.
See the definition of both the functions for ceil() function it says that it'll return the smallest integer value greater than or equal to x and on the other hand for the floor() function it says that it will return largest integer value less than or equal to x.
Focus on the underlined part that says or equal to x. It implies that if the value inside the variable is equal to the nearest integer value then the value to be returned would also be the same.
I hope you have understood my point.
Let's move ahead with other functions.
7. double fabs(double x)
Description: This function is used to return the absolute value of x.
Declaration syntax: double fabs(double x)
Parameters: x is any floating point value.
Illustration through example:
// Program to illustrate double fabs(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
float a, b;
a = 1234.1232;
b = -344.32;
printf("The absolute value of %f is %f\n", a, fabs(a));
printf("The absolute value of %f is %f\n", b, fabs(b));
return(0);
}
Output of the code above:
The absolute value of 1234.123169 is 1234.123169
The absolute value of -344.320007 is 344.320007
[Finished in 0.6s]
8. double fmod(double x, double y)
NOTE: This function takes two parameters in order to find the remainder.
Description: This function is used to return the remainder of x divided by y.
Declaration syntax: double fmod(double x, double y)
Parameters: x and y are any floating point values.
Illustration through example:
// Program to illustrate double fmod(double x, double y) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
float a, b, c;
a = 9.2;
b = 3.7;
c = 0;
printf("Remainder of %f / %f is %f\n", a, c, fmod(a,c));
printf("Remainder of %f / %f is %f\n", a, b, fmod(a,b));
return(0);
}
Output of the code above:
Remainder of 9.200000 / 0.000000 is nan
Remainder of 9.200000 / 3.700000 is 1.800000
[Finished in 0.7s]
Hey, Stop right there ✋
Did you notice something fishy in the output of this function?🤔
Look at the output of the first printf statement that basically gives remainder when a is divided by c. It says nan
What does it mean????
Don't worry if you don't know it, I'll explain it to you 😃
nan implies NOT A NUMBER. According to a definition this output is used to identify undefined or non-representable values for floating-point elements, such as the square root of negative numbers or the result of 0/0.
Now let's see why this output showed up inour case specifically.
Notice the highlighted line that says "the result of 0/0", although in our case there was no computation happening like this but it is somewhat similar.
Here's how?
In case of a/b i.e. 9.2/0 can you find any possible integer value that when you would multiply with 0 would return with a i.e. 9.2.
Take your time to think....
Did you find any? NO. Because any such value does not exist and that's why our output was nan.
I hope you understood what I wanted to convey.
Let's move ahead with some more functions.
9. double exp(double x)
Description: This function is used to return the value of e raised to the xth power..
Declaration syntax: double exp(double x)
Parameters: x is any floating point values.
Illustration through example:
// Program to illustrate double exp(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
float x = 0;
float y = 1;
float z = 2;
printf("The exponential value of %f is %f\n", x, exp(x));
printf("The exponential value of %f is %f\n", y, exp(y));
printf("The exponential value of %f is %f\n", z, exp(z));
return(0);
}
Output of the code above:
The exponential value of 0.000000 is 1.000000
The exponential value of 1.000000 is 2.718282
The exponential value of 2.000000 is 7.389056
[Finished in 0.6s]
10. double modf(double x, double *integer)
Description: This function is used to return the fraction component (part after the decimal), and sets integer to the integer component.
Declaration syntax: double modf(double x, double *integer)
Parameters: x is any floating point value and integer is the pointer to an object where the integralpart is to be stored.
Illustration through example:
// Program to illustrate double modf(double x, double *integer) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
float x, fractpart, intpart;
x = 5.143532;
fractpart = modf(x, &intpart);
printf("Integral part = %f\n", intpart);
printf("Fraction Part = %f\n", fractpart);
return(0);
}
Output of the code above:
Integral part = 5.000000
Fraction Part = 0.143532
[Finished in 0.6s]
11. double pow(double x, double y)
Description: This function is used to return the x raised to the power of y.
Declaration syntax: double pow(double x, double y)
Parameters: x and y are any floating point values.
Illustration through example:
// Program to illustrate double pow(double x, double y) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
printf("Value 5.0 ^ 3 = %f\n", pow(5.0, 3));
printf("Value 1.05 ^ 1.98 = %f\n", pow(1.05, 1.98));
return(0);
}
Output of the code above:
Value 5.0 ^ 3 = 125.000000
Value 1.05 ^ 1.98 = 1.101425
[Finished in 0.8s]
12. double sqrt(double x)
Description: This function is used to return the square root of x.
Declaration syntax: double sqrt(double x)
Parameters: x is any floating point value.
Illustration through example:
// Program to illustrate double sqrt(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
printf("Square root of %f is %f\n", 7.9, sqrt(7.9) );
printf("Square root of %f is %f\n", 5.123, sqrt(5.123) );
return 0;
}
Output of the code above:
Square root of 7.900000 is 2.810694
Square root of 5.123000 is 2.263405
[Finished in 0.9s]
13. double log(double x)
Description: This function is used to return the natural logarithm (base-e logarithm) of x.
Declaration syntax: double log(double x)
Parameters: x is any floating point value.
Illustration through example:
// Program to illustrate double log(double x) function.
#include <stdio.h> // included stdio.h header file to use standard I/O functions.
#include <math.h> // included math.h header file to use its functions.
int main () {
double x, ret;
x = 5.2;
ret = log(x);
printf("log(%f) = %f\n", x, ret);
return 0;
}
Output of the code above:
log(5.200000) = 1.648659
[Finished in 0.8s]
There are a lot more functions in math.h but in order to not make this article overwhelming I discussed only 13 of them. These functions are the ones that are regularly used by programmers/developers.
Finally in the second part of the artcile we've understood a lot of new functions of the math.h header file. not only that we've also learnt how to implement them in our programs.
That brings us to the 3rd and the final part of this article and that is the QUIZ.
BUT WAIT...I HAVE A SURPRISE FOR YOU GUYS AND I KNOW YOU DON'T WANT TO MISS IT 😉.
HERE YOU GO.... math.h header file contains a lot of functions to perform various mathematical operations but there is one more thing... and that is it contains ONE SPECIAL MACRO and it is 👇.
HUGE_VAL
This macro is used when the result of a function may not be representable as a floating point number. If magnitude of the correct result is too large to be represented, the function sets errno to ERANGE to indicate a range error, and returns a particular, very large value named by the macro HUGE_VAL or its negation (- HUGE_VAL).
If the magnitude of the result is too small, a value of zero is returned instead. In this case, errno might or might not be set to ERANGE.
Now we come to the last and the final part of this article and that's the quiz 👇.
QUIZ TIME 🥳
Do not miss this quiz as it'll help you to remember what you've learnt so far in this article.
Question 1.
How many arguments does the double ceil(double x) function accepts? and what does it return?
Question 2.
What is the full form of nan that shows up in the output?
Question 3.
How many macros are there in the math.h header file? And what are the respective names?
Question 4.
What is the extension of any header file in c language?
Ending note:
- I know this article can be overwhelming while reading it for the first time. But I assure you that if you'll read it twice or thrice you'll definetly feel that you've gained some good amount of knowledge.
- Practice makes a man perfect so don't just read the article and think that you've learn everything rather keep your pace slow but execute all the functions on your own ATLEAST ONCE. Beleive me it'll help you retain what you've learnt.
With this article at OpenGenus, you must have the complete idea of Header file math.h in C language. Enjoy.